How to Fix TypeError: Must Be Real Number, Not STR
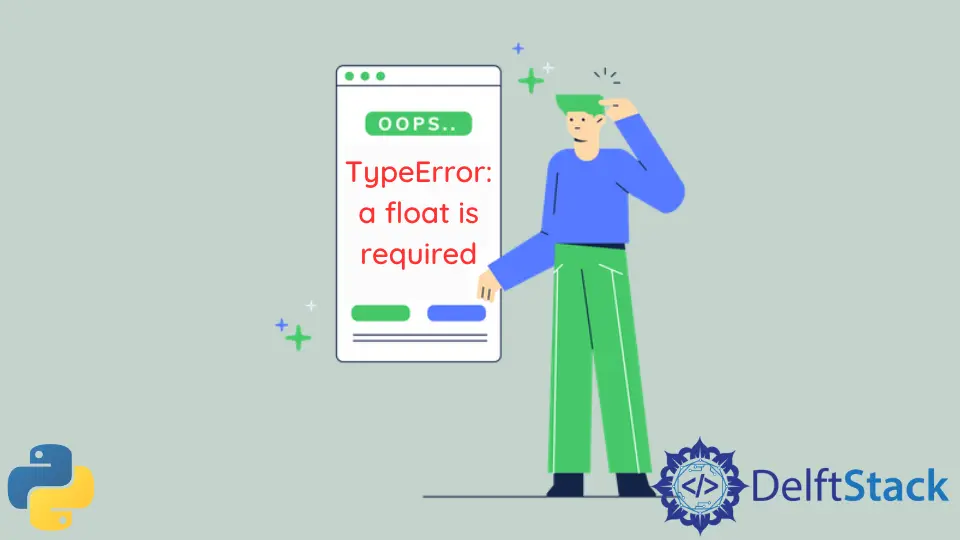
The TypeError: must be real number, not str
error involves using a wrong type and a non-real number, and in this case, an str
type.
Working with datatypes can be tricky, but it is important to enforce or ensure you are parsing the right data type to a function to avoid such TypeError
.
This article will explain how the TypeError: must be real number, not str
error occurs in the first place and how to solve it using type conversion.
Use float()
or int()
to Solve TypeError: must be real number, not str
in Python
When working with functions, especially built-in functions, the arguments required are often of a particular type. It could be any of the primitive data types, int
, float
, string
, or Boolean
.
Therefore, it is important to make sure that the values we are working with and parsing are of the right data.
A typical example is working with the built-in input()
function to take a number and work with the number in a simple mathematical expression.
number = input("Enter a Number: ")
print(number / 34 + 45 * number)
Output:
Enter a Number: 12
Traceback (most recent call last):
File "C:\Users\akinl\Documents\Python\steps.py", line 2, in <module>
print(number/34 + 45 * number)
TypeError: unsupported operand type(s) for /: 'str' and 'int'
Here, we have a TypeError
message because the number binding held a string data 12; instead of an integer or float number, 12.
However, this TypeError: unsupported operand type(s) for /: 'str' and 'int'
is different from this TypeError: must be real number, not str
error. The distinction is in the operation that’s happening.
Let’s use the math
library in Python to round down the number that the user inputs.
import math
number = input("Enter a Number: ")
print(math.floor(number))
Output:
Enter a Number: 12.45
Traceback (most recent call last):
File "C:\Users\akinl\Documents\Python\steps.py", line 3, in <module>
print(math.floor(number))
TypeError: must be real number, not str
Now, the TypeError
is different because we are parsing the number
binding, which contains a string to the floor()
method, and the method requires a number, float
or integer
.
Therefore, to solve the problem, we need to convert the datatype of the value the user passes to the number
binding to float
or integer
, depending on what we need.
The built-in float()
function is most appropriate when dealing with floating point numbers. Given that we need decimal numbers to round up, we need the float()
function.
import math
number = float(input("Enter a Number: "))
print(math.floor(number))
Output:
Enter a Number: 123.45
123
The int()
function can be useful for cases where the number needed is a whole number. If we only need whole numbers to be parsed to the sin()
method, we can use the int()
method.
import math
number = int(input("Enter a Number: "))
print(math.sin(number))
Output:
Enter a Number: 12
-0.5365729180004349
It can be easier to solve for simpler cases but trickier for some complex or harder scenarios. For example, it might be hard to see where you need to convert if you are working on a randomized trigonometric calculator.
It is always better to convert immediately, especially before the operation expression. In this case, the mathematical operation.
import random
import math
def create():
global sideA
sideA = format(random.uniform(1, 100), ".0f")
global sideB
sideB = format(random.uniform(1, 100), ".0f")
global angleA
angleA = format(random.uniform(1, 180), ",.3f")
global angleB
angleB = ANGLE_B()
return angleB
def ANGLE_B():
angle = format(math.asin(sideB * (math.sin(angleA) / sideA)), ".3f")
return angle
print(create())
Output:
Traceback (most recent call last):
File "c:\Users\akinl\Documents\Python\float.py", line 18, in <module>
print(create())
File "c:\Users\akinl\Documents\Python\float.py", line 11, in create
angle_b = ANGLE_B()
File "c:\Users\akinl\Documents\Python\float.py", line 15, in ANGLE_B
ang = format(math.asin(side_b*(math.sin(angle_a)/side_a)), '.3f')
TypeError: must be real number, not str
If you trace the error you can see it starts from print(create())
which calls the ANGLE_B()
function uses the binding sideA
, sideB
, and angleA
.
These bindings are parsed to the math
methods, which require float
and int
data values. However, the binding’s datatype is strings
and needs to be converted to either float
or int
.
In this case, the more responsible way to solve the code issue is to convert the datatype before use in mathematical expression since we don’t need to change the value again.
import random
import math
def create():
global sideA
sideA = float(format(random.uniform(1, 100), ".0f"))
global sideB
sideB = float(format(random.uniform(1, 100), ".0f"))
global angleA
angleA = float(format(random.uniform(1, 180), ",.3f"))
global angleB
angleB = ANGLE_B()
return angleB
def ANGLE_B():
angle = math.asin(sideB * (math.sin(angleA) / sideA))
return angle
print(create())
Output:
0.7293575839721542
Therefore, be defensive when dealing with datatypes, and make sure that after working with the date, convert the data to the necessary datatype.
Olorunfemi is a lover of technology and computers. In addition, I write technology and coding content for developers and hobbyists. When not working, I learn to design, among other things.
LinkedInRelated Article - Python TypeError
- How to Fix the Python TypeError: List Indices Must Be Integers, Not List
- How to Fix TypeError: Iteration Over Non-Sequence
- How to Solve the TypeError: An Integer Is Required in Python
- How to Fix Python TypeError: Unhashable Type: List
- How to Solve the TypeError: Not All Arguments Converted During String Formatting in Python
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python