Python TypeError: 해시할 수 없는 유형: 목록
-
Python의
TypeError: unhashable type: 'list'
- Python의 해시 함수
-
Python에서
TypeError: unhashable type: 'list'
수정
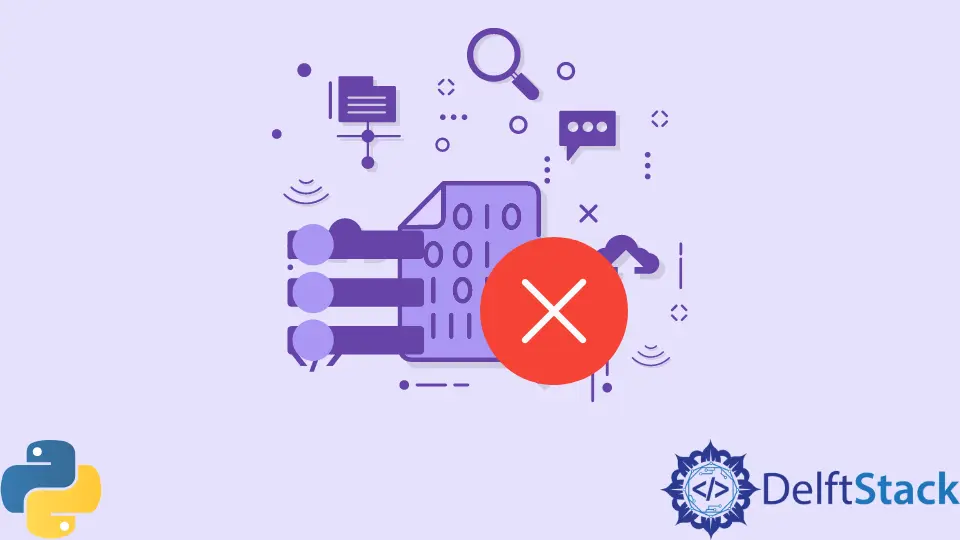
이 기사에서는 TypeError: unhashable type: 'list'
와 Python에서 이를 수정하는 방법에 대해 설명합니다.
Python의 TypeError: unhashable type: 'list'
이 오류는 목록과 같은 해시할 수 없는 객체를 Python 사전에 키로 전달하거나 함수의 해시 값을 찾을 때 발생합니다.
사전은 키-값 쌍으로 작동하는 Python의 데이터 구조이며 모든 키에는 그에 대한 값이 있으며 값의 값에 액세스하려면 배열 인덱스와 같은 키가 필요합니다.
사전 구문:
dic = {"key": "Values"}
해시 가능한 객체는 시간이 지나도 값이 변경되지 않지만 동일한 튜플
로 유지되는 객체이며 문자열
은 해시 가능한 객체 유형입니다.
암호:
# creating a dictionary
dic = {
# list as a key --> Error because lists are immutable
["a", "b"]: [1, 2]
}
print(dic)
출력:
TypeError: unhashable type: 'list'
목록 ["a","b"]
를 키
로 사용했지만 컴파일러에서 TypeError: unhashable type: 'list'
가 발생했습니다.
목록의 해시 값을 수동으로 찾아봅시다.
암호:
lst = ["a", "b"]
hash_value = hash(lst)
print(hash_value)
출력:
TypeError: unhashable type: 'list'
hash()
함수는 주어진 객체의 해시 값을 찾는 데 사용되지만 객체는 string
, tuple
등과 같이 변경할 수 없어야 합니다.
Python의 해시 함수
hash()
함수는 불변 개체를 암호화하고 개체의 해시 값으로 알려진 고유한 값을 할당하는 암호화 기술입니다. 데이터의 크기에 관계없이 동일한 크기의 고유한 값을 제공합니다.
암호:
string_val = "String Value"
tuple_val = (1, 2, 3, 4, 5)
msg = """Hey there!
Welcome to DelfStack"""
print("Hash of a string object\t\t", hash(string_val))
print("Hash of a tuple object\t\t", hash(tuple_val))
print("Hash of a string message\t", hash(tuple_val))
출력:
Hash of a string object -74188595
Hash of a tuple object -1883319094
Hash of a string message -1883319094
해시 값은 크기가 같고 모든 값에 대해 고유합니다.
Python에서 TypeError: unhashable type: 'list'
수정
Python에서 TypeError를 수정하려면 불변 객체를 사전의 키와 hash()
함수의 인수로 사용해야 합니다. 위의 코드에서 hash()
함수는 tuple
및 string
과 같은 가변 개체와 완벽하게 작동합니다.
사전에서 TypeError: unhashable type: 'list'
를 수정하는 방법을 살펴보겠습니다.
암호:
# creating a dictionary
dic = {
# string as key
"a": [1, 2]
}
print(dic)
출력:
{'a': [1, 2]}
이번에는 "a"
문자열을 키로 제공합니다. 문자열
은 변경 가능하기 때문에 작업하기에 좋습니다.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn관련 문장 - Python Error
- AttributeError 수정: Python에서 'generator' 객체에 'next' 속성이 없습니다.
- AttributeError 해결: 'list' 객체 속성 'append'는 읽기 전용입니다.
- AttributeError 해결: Python에서 'Nonetype' 객체에 'Group' 속성이 없습니다.
- AttributeError: 'Dict' 객체에 Python의 'Append' 속성이 없습니다.
- AttributeError: 'NoneType' 객체에 Python의 'Text' 속성이 없습니다.
- AttributeError: Int 객체에 속성이 없습니다.