Python Traceback Most Recent Call Last
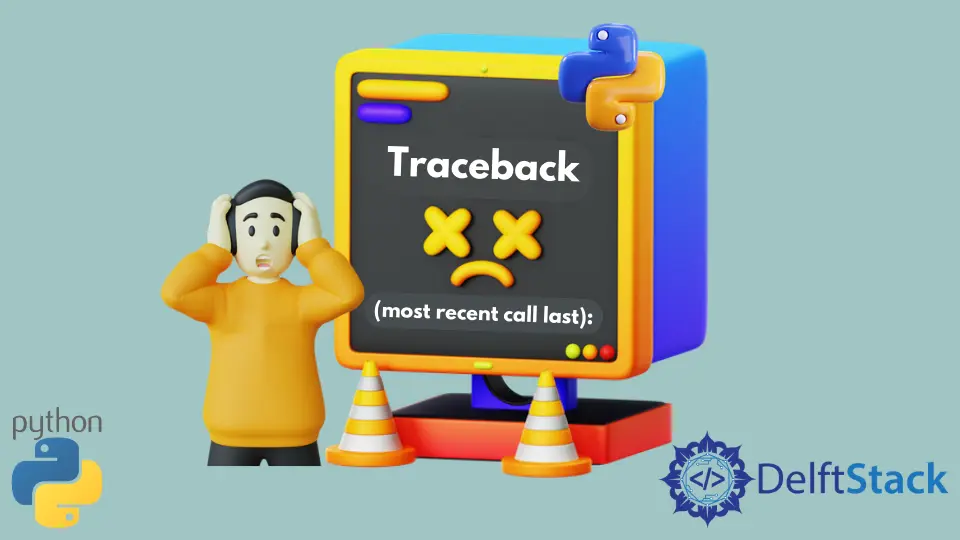
The Python traceback reports information about an exception that occurred in the code. It helps to find the error type and additional information in your code.
The traceback shows the exact line where the exception has been raised. Some of the common traceback errors are IndexError
, ImportError
, NameError
, ValueError
, SyntaxError
, TypeError
, AttributeError
, and KeyError
.
This tutorial will teach you to read and fix the traceback error in Python.
Fix the Traceback (most recent call last)
Error in Python
Let’s see examples of a few traceback errors in Python.
-
IndexError
: TheIndexError
occurs when you try to get an index from a list that is not present in the list.The following example raises an
IndexError
when it is run.mylist = ["car", "bus", "truck"] mylist[3]
Output:
Traceback (most recent call last): File "c:\Users\rhntm\myscript.py", line 2, in <module> mylist[3] IndexError: list index out of range
Below the
Traceback (most recent call last):
, you can find the file name and line number where the error has occurred. Themylist[5]
indicates the exact code which causes the exception.The traceback error also shows the type of error and information about that error. The above case is
IndexError: list index out of range
.You can fix it using the valid index number to retrieve an item from a list.
mylist[2]
Output:
'truck'
-
NameError
: TheNameError
is raised when you use a variable or function not defined in your code.Here is an example of a
NameError
in Python.name = input("Enter your name: ") print(username)
Output:
Enter your name: Rohan Traceback (most recent call last): File "c:\Users\rhntm\myscript.py", line 2, in <module> print(username) NameError: name 'username' is not defined
The error says
NameError: name 'username' is not defined
because the variableusername
is not defined in the code.So you must only call the variable or function defined in the code.
name = input("Enter your name: ") print(name)
Output:
Enter your name: Rohan Rohan
-
ValueError
: TheValueError
is raised when you give a valid argument to a function, but it is an invalid value.For example, you will get the
ValueError
when you provide a negative number to thesqrt()
function of amath
module.import math math.sqrt(-5)
Output:
Traceback (most recent call last): File "c:\Users\rhntm\myscript.py", line 2, in <module> math.sqrt(-5) ValueError: math domain error
Because the function taking a number argument is correct, but the negative value is invalid, resulting in a
ValueError: math domain error
.
Now you know different traceback errors in Python. The tracebacks help find the errors in the code.
You can read tracebacks to know why the exception occurred in the code. Then you can fix the errors and run the code again successfully.
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python