How to Fix NameError: Variable Is Not Defined in Python
- Scope of Variables in Python
-
the
NameError
in Python -
Fix the
NameError: Variable is not defined
in Python
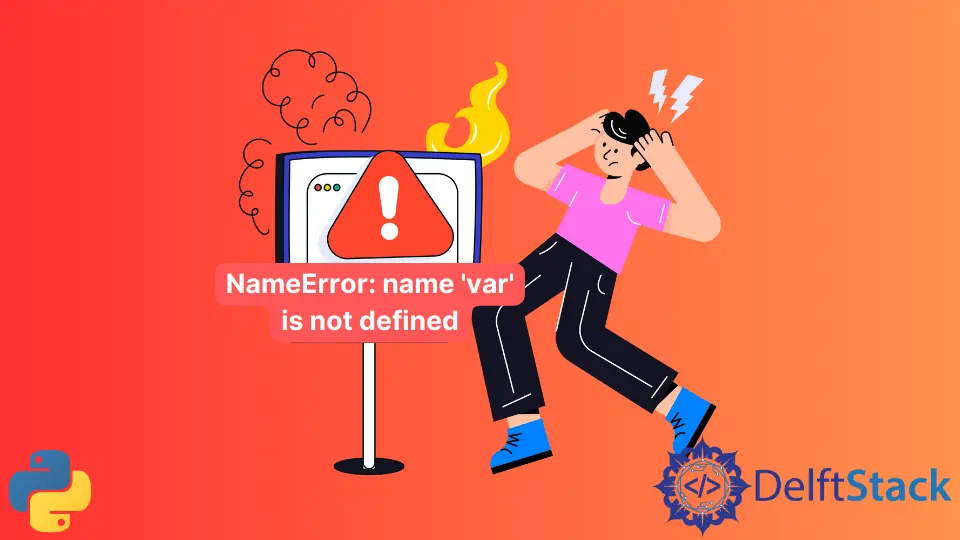
This article will discuss the causes of NameError
in Python and how to fix the particular error NameError: Variable is not defined
.
Scope of Variables in Python
Scope of variables implements accessibility constraints on variables that either can be accessed out of a specific block or not. The lifetime of some variables is just inside a particular block, while others can be accessed throughout the program.
Let us understand it through an example:
# global scope
a = 3
# Function to add two numbers
def displayScope():
# local varaible
b = 2
# sum a and b
c = a + b
print("The sum of a & b = ", c)
displayScope()
Output:
The sum of a & b = 5
In this example, the variable a
is defined at the top, and it isn’t enclosed in any block, so it is accessible throughout the program. But the variable b
is defined locally inside the function block; therefore, it isn’t accessible outside the block.
the NameError
in Python
In Python, the NameError
occurs in run time during the execution of a variable, library, function, or a string without the single or double quotes, which are types in the code without any declaration. Secondly, when you call the functions or variables whose scope is local and cannot be accessed globally, the Python interpreter throws the NameError
and says that the name 'name' is not defined
.
Causes of the NameError
in Python
The cause of the NameError
is the call to an invalid function, variable, or library. To understand the reason clearly, let’s have an example:
# invalid funciton call
def test_ftn():
return "Test function"
print(test_ft()) # calling the the function which does not exist
# printing invalid varaible
name = "Zeeshan Afridi"
print(Name) # printing variable `Name` which does not exist
Both are the causes of NameError
in Python because, in the first example, we called an unavailable function. The function name is test_ftn
, whereas we are calling the test_ft
function.
In the second example, the name
variable is assigned to the string Zeeshan Afridi
, but we are printing Name
, which is not even declared in the program. That’s why we obtained the NameError: name 'test_ft' is not defined
.
Fix the NameError: Variable is not defined
in Python
In the above examples, we got the NameError
because we have called a variable out of scope. Let’s see how we can fix this NameError: variable is not defined
.
# global scope
a = 3
# Function to add two numbers
def displayScope():
# local varaible
b = 2
print("The value of a = ", a)
print("The value of b = ", b)
Output:
The value of a = 3
NameError: name 'b' is not defined
The above code has displayed the value of a
because it is accessible throughout the program. On the other hand, the variable b
is locally defined, so it is only known to the function displayScope()
; you cannot access it out of the scope.
This has caused the error NameError: name 'b' is not defined
.
Fortunately, Python has introduced the global
reserve keyword to fix this problem. This global
reserve keyword is used to increase the scope of a local variable so the variable can be accessed throughout the program globally.
Let’s understand it through an example:
# global scope
a = 3
# Function to add two numbers
def displayScope():
# local scope
global c
z = 2
c = a + b
print("The value of c =", c)
Output:
The value of c = 5
In this example, the variable c
is defined inside the local scope of displayScope()
, but we can access it globally because of the magic keyword global
. Now, c
is accessible throughout because it is defined as global
.
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedInRelated Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python