How to Fix the TypeError: must be str, not int in Python
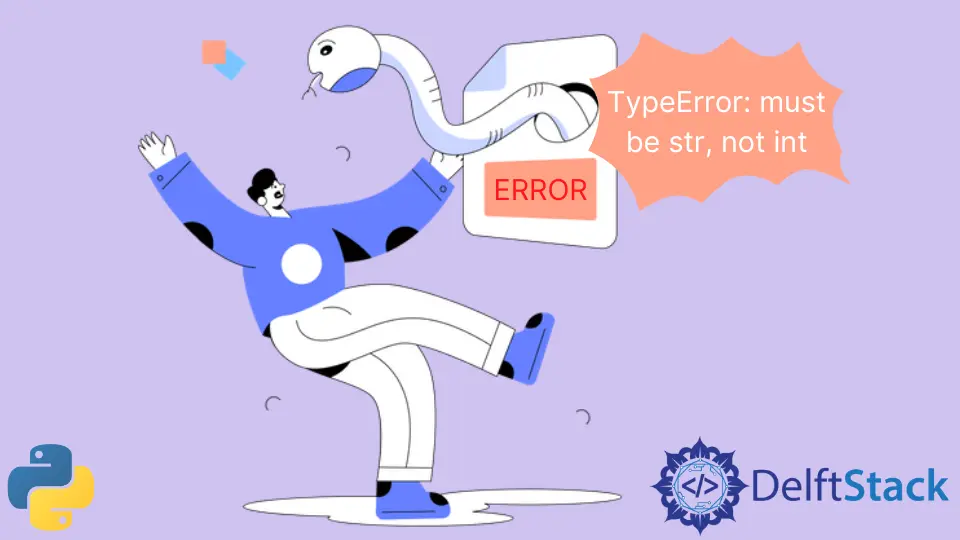
String concatenation refers to combining multiple strings into a single string. In Python, we can use the +
symbol between variables referring to strings or raw strings themselves to join them together. Or, we can put all the strings that we wish to join inside a list and use the built-in join()
method Python to merge them into one.
While using both the methods above, if we are not careful with the data types of the variables and the raw values, we can run into the TypeError
exception. This article will talk about how to fix this issue in Python.
Fix the TypeError: must be str, not int
in Python
We will discuss a couple of ways that we can use to fix this error in Python.
the Obvious Approach
The first solution is an obvious one; be alert about the variables and the raw values you are using. Try not to concatenate a string with an integer, class object, or boolean value.
the str()
Method in Python
The second solution is to use the built-in str()
method in Python. This method returns a string version of the passed object, for example, an integer, a floating-point value, a boolean, a class object, a list, etc. For class objects, this method returns the result of the __repr__()
method or the __str__()
method. Refer to the following Python code to understand this str()
function practically.
class A:
def __init__(self, x):
self.x = x
def __str__(self):
return f"{self.x}"
print(str(1))
print(str(111.1))
print(str(False))
print(str(True))
print(str(None))
print(str(A(11111)))
print(str([1, 2, 3, 4, 5]))
Output:
1
111.1
False
True
None
11111
[1, 2, 3, 4, 5]
Now, let us see how to use this function for string concatenation. Refer to the following code for the same.
a = "Hello World "
b = 1923
c = " Python "
d = 1235.23
e = " Numbers: "
f = [100, 200, 400, 800, 1600]
x = a + str(b) + c + str(d) + e + str(f)
print(x)
Output:
Hello World 1923 Python 1235.23 Numbers: [100, 200, 400, 800, 1600]
The str()
function will convert all the values into their respective string values. And, further, we can safely concatenate the strings together without running into any exceptions.
Formatted Strings in Python
The third way is to use formatted strings. Formatted strings refer to strings prefixed with an f
. These strings allow us to insert variables or logic inside regular strings. The formulated or the final string would have the string representations of the values stored by the variables and the values returned by the logic or function calls. The logic and the variables are inserted inside {}
present inside the strings.
Refer to the following Python code for the discussed approach.
a = 1923
b = 1235.23
c = [100, 200, 400, 800, 1600]
print(f"Hello World {a} Python {b} Numbers: {c}")
Output:
Hello World 1923 Python 1235.23 Numbers: [100, 200, 400, 800, 1600]
Note the presence of an f
at the beginning of the string and multiple {}
inside the string.
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python