修复 Python 中的 TypeError: must be str, not int
Vaibhav Vaibhav
2022年5月18日
Python
Python Error
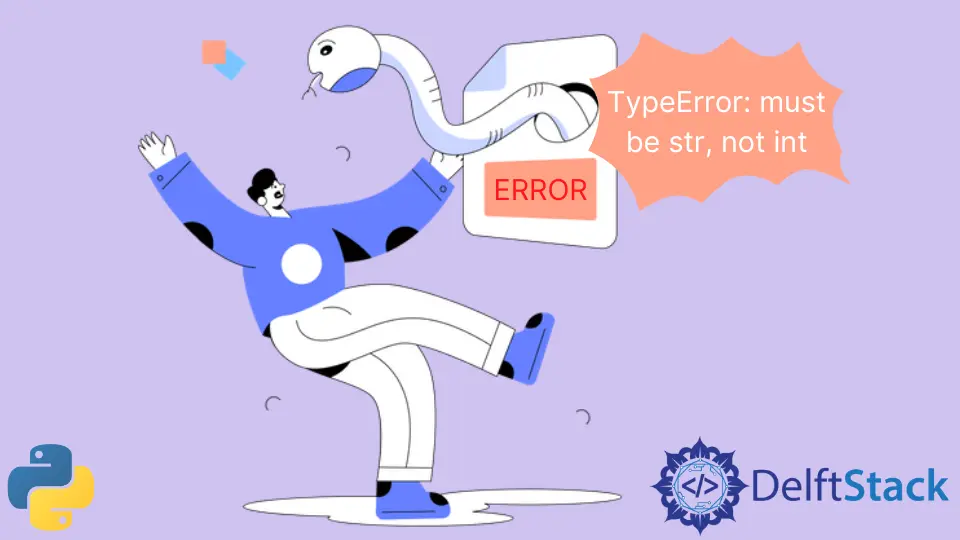
字符串连接是指将多个字符串组合成一个字符串。在 Python 中,我们可以在引用字符串或原始字符串本身的变量之间使用 +
符号将它们连接在一起。或者,我们可以将所有希望加入的字符串放在一个列表中,并使用 Python 内置的 join()
方法将它们合并为一个。
在使用上述两种方法时,如果我们不注意变量和原始值的数据类型,我们可能会遇到 TypeError
异常。本文将讨论如何在 Python 中解决此问题。
修复 Python 中的 TypeError: must be str, not int
我们将讨论几种可用于在 Python 中修复此错误的方法。
显而易见的方法
第一个解决方案是显而易见的;请注意你正在使用的变量和原始值。尽量不要将字符串与整数、类对象或布尔值连接。
Python 中的 str()
方法
第二种解决方案是使用 Python 中内置的 str()
方法。此方法返回传递对象的字符串版本,例如整数、浮点值、布尔值、类对象、列表等。对于类对象,此方法返回 __repr__()
方法或 __str__()
方法。请参考下面的 Python 代码来实际理解这个 str()
函数。
class A:
def __init__(self, x):
self.x = x
def __str__(self):
return f"{self.x}"
print(str(1))
print(str(111.1))
print(str(False))
print(str(True))
print(str(None))
print(str(A(11111)))
print(str([1, 2, 3, 4, 5]))
输出:
1
111.1
False
True
None
11111
[1, 2, 3, 4, 5]
现在,让我们看看如何使用此函数进行字符串连接。请参阅以下代码。
a = "Hello World "
b = 1923
c = " Python "
d = 1235.23
e = " Numbers: "
f = [100, 200, 400, 800, 1600]
x = a + str(b) + c + str(d) + e + str(f)
print(x)
输出:
Hello World 1923 Python 1235.23 Numbers: [100, 200, 400, 800, 1600]
str()
函数会将所有值转换为它们各自的字符串值。此外,我们可以安全地将字符串连接在一起而不会遇到任何异常。
Python 中的格式化字符串
第三种方法是使用格式化字符串。格式化字符串是指以 f
为前缀的字符串。这些字符串允许我们在常规字符串中插入变量或逻辑。公式化的或最终的字符串将具有由变量存储的值和由逻辑或函数调用返回的值的字符串表示。逻辑和变量被插入到字符串中的 {}
中。
有关讨论的方法,请参阅以下 Python 代码。
a = 1923
b = 1235.23
c = [100, 200, 400, 800, 1600]
print(f"Hello World {a} Python {b} Numbers: {c}")
输出:
Hello World 1923 Python 1235.23 Numbers: [100, 200, 400, 800, 1600]
注意字符串开头有一个 f
,字符串内有多个 {}
。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Vaibhav Vaibhav