修复 Python 中数组索引过多的错误
Vaibhav Vaibhav
2022年5月18日
Python
Python Error
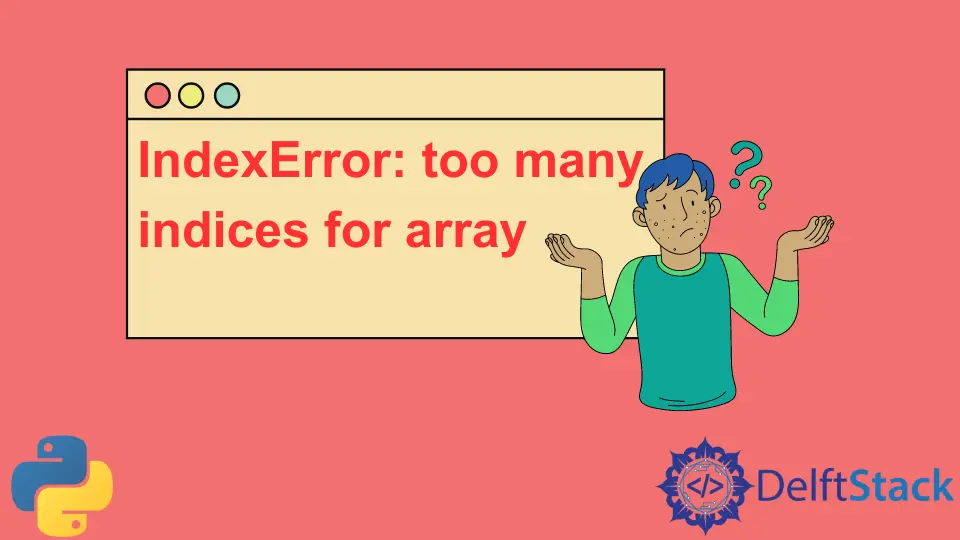
数组或列表和元组是 Python 中的连续数据结构。它们可以将属于一种数据类型和多种数据类型的元素存储在一起。例如,我们可以有一个包含五个整数元素、三个浮点数、七个类对象、两个集合和八个布尔值的列表、数组或元组。
要访问这些元素,我们可以使用这些元素的索引。这些数据结构是基于 0 索引的。假设我们尝试访问索引大于或等于数据结构长度的元素。在这种情况下,我们会遇到 IndexError
异常。
在本文中,我们将学习如何在 Python 中解决此问题。
如何修复 Python 中的 too many indices for array error
要修复 IndexError
异常,应确保他们输入的索引不等于或大于数据结构的长度。
上面提到的方法是显而易见的。但是,我们仍然可以编写存根或实用函数来从列表或元组中获取值。此函数将确保我们在索引处获取值(如果存在)并安全地处理无效的索引值。请参阅以下 Python 代码。
def get_value(structure, index):
if not isinstance(index, int):
return None
if not isinstance(structure, (list, tuple)):
return None
if index >= len(structure):
return None
return structure[index]
a = ["Hello", "World", 1, 2.0, 3.00000, True, False]
print(get_value([], 4.0))
print(get_value(a, 4))
print(get_value(a, -1))
print(get_value(None, 8))
print(get_value("Hello World", "Python"))
输出:
None
3.0
False
None
None
在返回指定索引处的值之前,上面的存根函数确保索引是 int
类型,数据结构是 list
或 tuple
类型,并且索引小于数据结构的长度.一旦通过了所有检查,我们就可以安全地在索引处返回所需的值。
get_value()
函数的一种更简单的实现是使用 try
和 except
块。请参阅以下 Python 代码。
def get_value(structure, index):
try:
return structure[index]
except:
return None
a = ["Hello", "World", 1, 2.0, 3.00000, True, False]
print(get_value([], 4.0))
print(get_value(a, 4))
print(get_value(a, -1))
print(get_value(None, 8))
print(get_value("Hello World", "Python"))
输出:
None
3.0
False
None
None
如果出现任何问题,try
和 except
块将返回 None
,如果成功满足所有必需条件,则返回实际值。
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Vaibhav Vaibhav