How to Fix the Too Many Indices for Array Error in Python
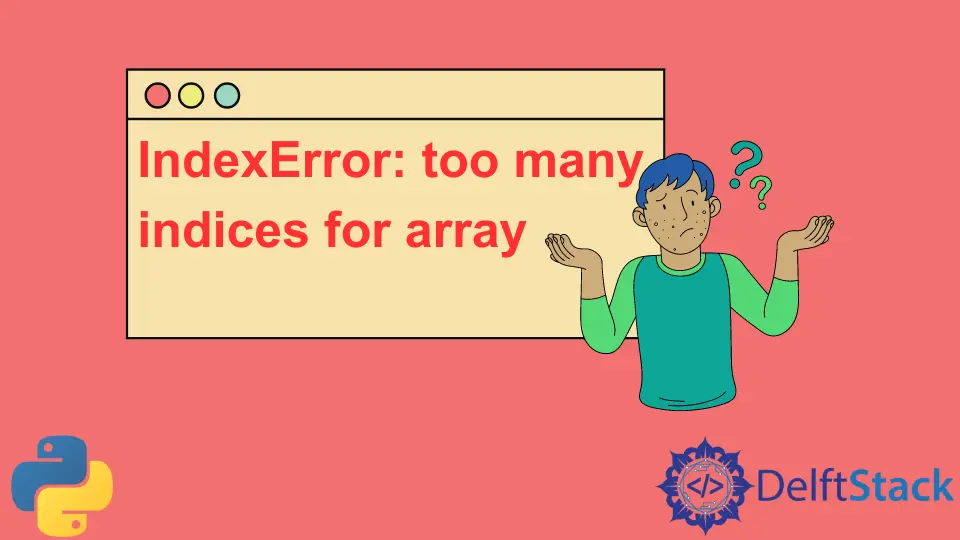
Arrays or Lists and Tuples are contiguous data structures in Python. They can store elements belonging to a single data type and multiple data types together. For example, we can have a list or array or tuple that contains five integer elements, three floating-point numbers, seven class objects, two sets, and eight boolean values.
To access these elements, we can use the indexes of these elements. These data structures are 0-index based. Suppose we try to access an element at an index greater than or equal to the length of the data structure. In that case, we run into the IndexError
exception.
In this article, we will learn how to fix this issue in Python.
How to Fix the too many indices for array error
in Python
To fix the IndexError
exception, one should make sure that they don’t enter an index equal to or greater than the length of the data structure.
The approach mentioned above is an obvious one. However, we can still write a stub or a utility function to grab a value from a list or tuple. This function will ensure we get the value at an index if it exists and safely handle invalid index values. Refer to the following Python code for the same.
def get_value(structure, index):
if not isinstance(index, int):
return None
if not isinstance(structure, (list, tuple)):
return None
if index >= len(structure):
return None
return structure[index]
a = ["Hello", "World", 1, 2.0, 3.00000, True, False]
print(get_value([], 4.0))
print(get_value(a, 4))
print(get_value(a, -1))
print(get_value(None, 8))
print(get_value("Hello World", "Python"))
Output:
None
3.0
False
None
None
Before returning the value at the specified index, the stub function above ensures that the index is of type int
, the data structure is of type list
or tuple
, and the index is less than the length of the data structure. Once all the checks are passed, we can safely return the required value at the index.
One easier implementation of the get_value()
function would be using the try
and except
block. Refer to the following Python code for the same.
def get_value(structure, index):
try:
return structure[index]
except:
return None
a = ["Hello", "World", 1, 2.0, 3.00000, True, False]
print(get_value([], 4.0))
print(get_value(a, 4))
print(get_value(a, -1))
print(get_value(None, 8))
print(get_value("Hello World", "Python"))
Output:
None
3.0
False
None
None
The try
and except
block would return None
if anything goes wrong and an actual value if all the required conditions are successfully met.
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python