修復 Python 中陣列索引過多的錯誤
Vaibhav Vaibhav
2022年5月18日
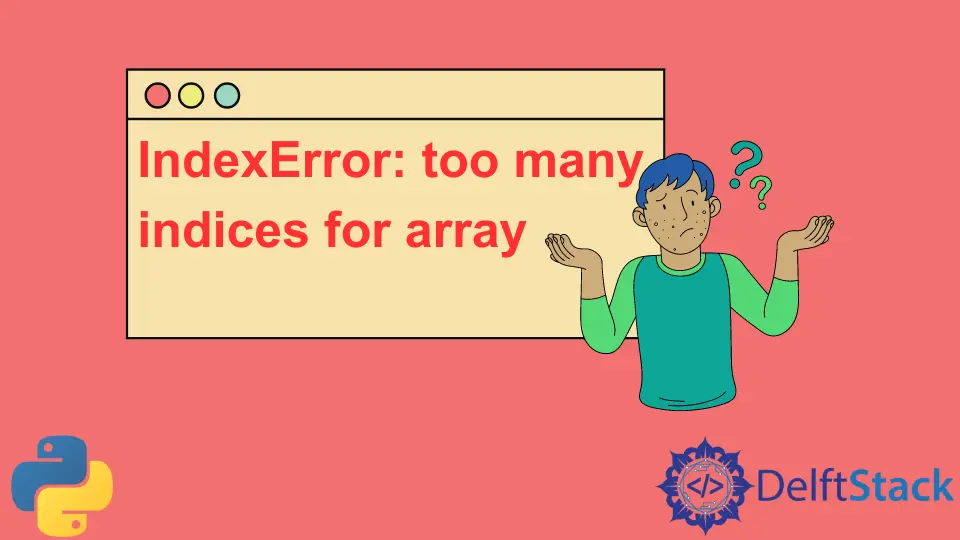
陣列或列表和元組是 Python 中的連續資料結構。它們可以將屬於一種資料型別和多種資料型別的元素儲存在一起。例如,我們可以有一個包含五個整數元素、三個浮點數、七個類物件、兩個集合和八個布林值的列表、陣列或元組。
要訪問這些元素,我們可以使用這些元素的索引。這些資料結構是基於 0 索引的。假設我們嘗試訪問索引大於或等於資料結構長度的元素。在這種情況下,我們會遇到 IndexError
異常。
在本文中,我們將學習如何在 Python 中解決此問題。
如何修復 Python 中的 too many indices for array error
要修復 IndexError
異常,應確保他們輸入的索引不等於或大於資料結構的長度。
上面提到的方法是顯而易見的。但是,我們仍然可以編寫存根或實用函式來從列表或元組中獲取值。此函式將確保我們在索引處獲取值(如果存在)並安全地處理無效的索引值。請參閱以下 Python 程式碼。
def get_value(structure, index):
if not isinstance(index, int):
return None
if not isinstance(structure, (list, tuple)):
return None
if index >= len(structure):
return None
return structure[index]
a = ["Hello", "World", 1, 2.0, 3.00000, True, False]
print(get_value([], 4.0))
print(get_value(a, 4))
print(get_value(a, -1))
print(get_value(None, 8))
print(get_value("Hello World", "Python"))
輸出:
None
3.0
False
None
None
在返回指定索引處的值之前,上面的存根函式確保索引是 int
型別,資料結構是 list
或 tuple
型別,並且索引小於資料結構的長度.一旦通過了所有檢查,我們就可以安全地在索引處返回所需的值。
get_value()
函式的一種更簡單的實現是使用 try
和 except
塊。請參閱以下 Python 程式碼。
def get_value(structure, index):
try:
return structure[index]
except:
return None
a = ["Hello", "World", 1, 2.0, 3.00000, True, False]
print(get_value([], 4.0))
print(get_value(a, 4))
print(get_value(a, -1))
print(get_value(None, 8))
print(get_value("Hello World", "Python"))
輸出:
None
3.0
False
None
None
如果出現任何問題,try
和 except
塊將返回 None
,如果成功滿足所有必需條件,則返回實際值。
作者: Vaibhav Vaibhav