修復 Python 中的 TypeError: must be str, not int
Vaibhav Vaibhav
2022年5月18日
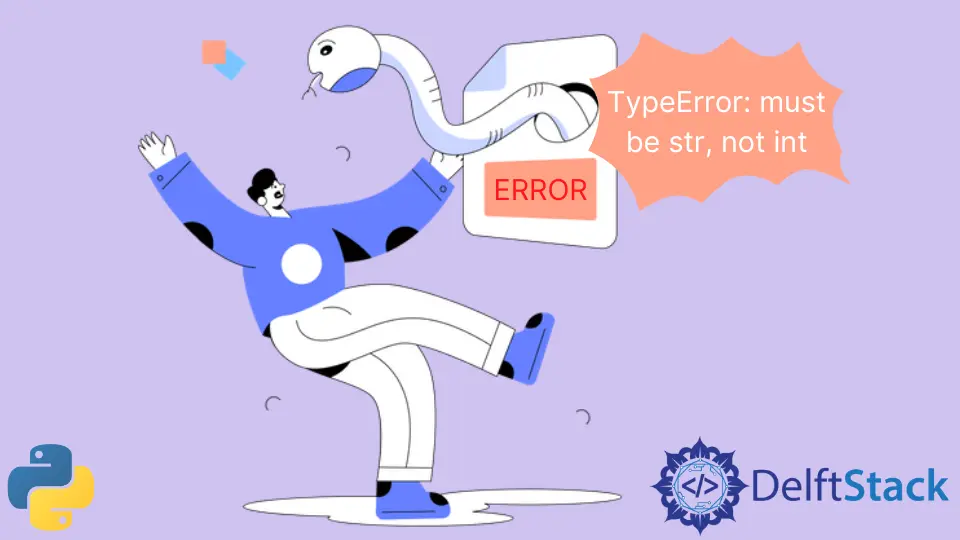
字串連線是指將多個字串組合成一個字串。在 Python 中,我們可以在引用字串或原始字串本身的變數之間使用 +
符號將它們連線在一起。或者,我們可以將所有希望加入的字串放在一個列表中,並使用 Python 內建的 join()
方法將它們合併為一個。
在使用上述兩種方法時,如果我們不注意變數和原始值的資料型別,我們可能會遇到 TypeError
異常。本文將討論如何在 Python 中解決此問題。
修復 Python 中的 TypeError: must be str, not int
我們將討論幾種可用於在 Python 中修復此錯誤的方法。
顯而易見的方法
第一個解決方案是顯而易見的;請注意你正在使用的變數和原始值。儘量不要將字串與整數、類物件或布林值連線。
Python 中的 str()
方法
第二種解決方案是使用 Python 中內建的 str()
方法。此方法返回傳遞物件的字串版本,例如整數、浮點值、布林值、類物件、列表等。對於類物件,此方法返回 __repr__()
方法或 __str__()
方法。請參考下面的 Python 程式碼來實際理解這個 str()
函式。
class A:
def __init__(self, x):
self.x = x
def __str__(self):
return f"{self.x}"
print(str(1))
print(str(111.1))
print(str(False))
print(str(True))
print(str(None))
print(str(A(11111)))
print(str([1, 2, 3, 4, 5]))
輸出:
1
111.1
False
True
None
11111
[1, 2, 3, 4, 5]
現在,讓我們看看如何使用此函式進行字串連線。請參閱以下程式碼。
a = "Hello World "
b = 1923
c = " Python "
d = 1235.23
e = " Numbers: "
f = [100, 200, 400, 800, 1600]
x = a + str(b) + c + str(d) + e + str(f)
print(x)
輸出:
Hello World 1923 Python 1235.23 Numbers: [100, 200, 400, 800, 1600]
str()
函式會將所有值轉換為它們各自的字串值。此外,我們可以安全地將字串連線在一起而不會遇到任何異常。
Python 中的格式化字串
第三種方法是使用格式化字串。格式化字串是指以 f
為字首的字串。這些字串允許我們在常規字串中插入變數或邏輯。公式化的或最終的字串將具有由變數儲存的值和由邏輯或函式呼叫返回的值的字串表示。邏輯和變數被插入到字串中的 {}
中。
有關討論的方法,請參閱以下 Python 程式碼。
a = 1923
b = 1235.23
c = [100, 200, 400, 800, 1600]
print(f"Hello World {a} Python {b} Numbers: {c}")
輸出:
Hello World 1923 Python 1235.23 Numbers: [100, 200, 400, 800, 1600]
注意字串開頭有一個 f
,字串內有多個 {}
。
作者: Vaibhav Vaibhav