-
Understand the Root Cause of the
ValueError: math domain error
in Python -
Replicate the
ValueError: math domain error
in Python -
Solve the
ValueError: math domain error
in Python
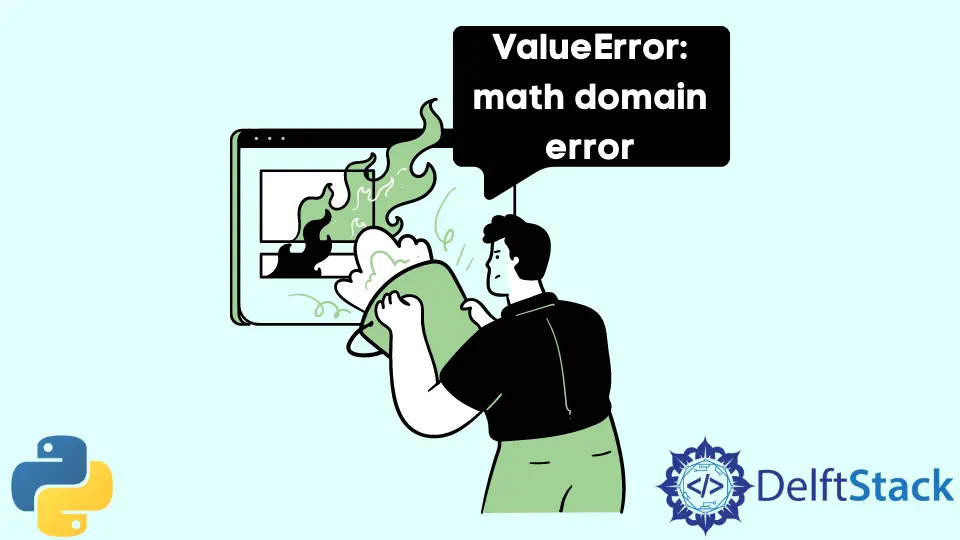
+++
title = “How to Solve ValueError: Math Domain Error in Python”
date = 2022-07-09
draft = false
keywords = [“python math domain error”]
description = “This tutorial demonstrates how to resolve the ValueError: math domain error in Python.”
tags = [“Python”, “Python Error”]
author = “Preet Sanghavi”
reviewer = “Jhonabylle Manzano”
inarticle = true
+++
In this tutorial, we aim to explore different methods to resolve the ValueError: math domain error
in Python.
This article tackles the following topics.
- Understanding the root cause of the problem.
- Replicating the issue.
- Resolving the issue.
Understand the Root Cause of the ValueError: math domain error
in Python
A ValueError: math domain error
is generally raised in Python whenever there is an inherent flaw in the usage of Mathematics (Basic or Advanced) in the coding aspect.
Dividing any integer or floating point value by zero, taking the logarithm of any non-positive number or multiplying any integer by infinity are some examples that generally lead to the ValueError: math domain error
.
Replicate the ValueError: math domain error
in Python
Now that we understand the reason behind the issue let us try replicating it. This can be done in Python with the help of the following block of code.
from numpy import zeros, array
from math import sin, log
def f(x):
f = log(-3) - 7.0
return "Executed successfully"
x = array([1.0, 1.0, 1.0])
a = f(x)
print(a)
Here, we are trying to calculate a simple expression that subtracts the result of log(-3)
and 7
. The code above gives us the error below.
line 5, in f
f = log(-3) - 7.0
ValueError: math domain error
This error is because we are trying to calculate the log
of a negative number, which is impossible.
Similarly, we get the ValueError: math domain error
if we use the sqrt
function with a negative number in Python.
This error can be replicated with the help of the following block of code.
from math import sqrt
print(sqrt(-4))
The output of the above code can be illustrated as follows.
line 2, in print(srqt(-4))
ValueError: math domain error
The primary reason is that a negative integer’s square root is a complex number.
Similarly, while using the pow
function in Python, we can get the ValueError: math domain error
with the following code block.
import math
print(math.pow(-2, 0.5))
The output of the above code block can be illustrated as:
line 2, in print(math.pow(-2, 0.5))
ValueError: math domain error
The primary reason is that a negative number cannot be raised to a fractional power in Python.
Solve the ValueError: math domain error
in Python
We can use the absolute operator abs
in Python to resolve the above issues. The following block of code can help us eliminate the error mentioned.
from numpy import zeros, array
from math import sin, log
def f(x):
f = log(abs(-3)) - 7.0
return "Executed successfully"
x = array([1.0, 1.0, 1.0])
a = f(x)
print(a)
The output of the above code can be illustrated as follows.
Executed successfully
Similarly, if we ever want to take the square root of a negative number, we can also use abs
. This can be understood better with the help of the following block of code.
from math import sqrt
print(sqrt(abs(-4)))
The output of the above code can be illustrated as follows.
2.0
The same logic can be used to calculate a negative number’s power.
import math
print(math.pow(abs(-2), 0.5))
The output of the above code can be illustrated as follows.
1.4142135623730951
Thus, with the help of this tutorial, we have managed to get rid of the ValueError: math domain error
in Python.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook