How to Fix Python TypeError: Missing 1 Required Positional Argument
- Not Instantiating an Object in Python
- Incorrectly Instantiating a Class Object in Python
-
Fix the
TypeError: missing 1 required positional argument: 'self'
Error in Python - Conclusion
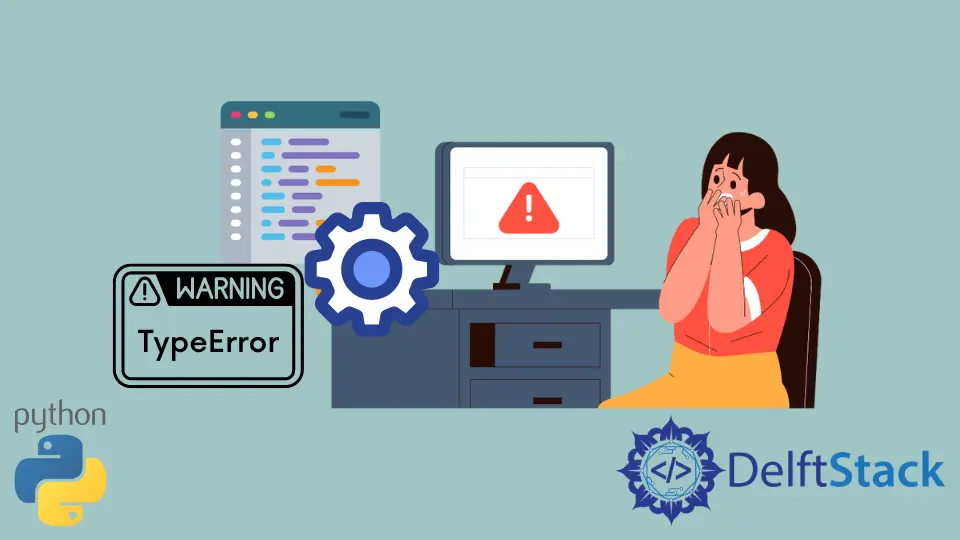
Classes are one of the fundamental features of Object-Oriented Programming languages. Every object belongs to some class in Python.
We can create our class as a blueprint to create objects of the same type. We use the class
keyword to define a class in Python.
A very important feature in Python is using the self
attribute while defining classes. The self
attribute represents the object’s data and binds the arguments to the object.
This tutorial will discuss the TypeError: missing 1 required positional argument: 'self'
error in Python and how we can solve it.
Let us discuss the situations where this error is raised.
Not Instantiating an Object in Python
Positional arguments refer to the data we provide a function with. For creating class objects, we use a constructor.
If the class requires any data, we must pass it as arguments in the constructor function.
This error is raised when we forget to instantiate the class object or wrongly instantiate the class instance.
See the code below.
class Delft:
def __init__(self, name):
self.name = name
def fun(self):
return self.name
m = Delft.fun()
print(m)
Output:
TypeError: fun() missing 1 required positional argument: 'self'
In the above example, we get the error because we forget to instantiate the instance of the class and try to access the function of the class. As a result, the self
attribute is not defined and cannot bind the attributes to the object.
Note that this is a TypeError
, which is raised in Python while performing some unsupported operation on a given data type. It is not supported to directly access the class method without creating an instance.
Incorrectly Instantiating a Class Object in Python
Another scenario where this error can occur is when we incorrectly instantiate the class object. This can be a small typing error like ignoring the parentheses or not providing the arguments.
For example:
class Delft:
def __init__(self, name):
self.name = name
def fun(self):
return self.name
m = Delft
a = m.fun()
print(a)
Output:
TypeError: fun() missing 1 required positional argument: 'self'
Let us now discuss how to fix this error in the section below.
Fix the TypeError: missing 1 required positional argument: 'self'
Error in Python
To fix this error, we can create the class instance and use it to access the class method.
See the code below.
class Delft:
def __init__(self, name):
self.name = name
def fun(self):
return self.name
m = Delft("Stack")
a = m.fun()
print(a)
Output:
Stack
In the example, we first create an instance of the class. This is then used to access the class method to return some value and print it; the error is fixed.
Another fix involves the use of static methods. Static methods are functions bound to a particular class and not to an object.
We can use the @staticmethod
decorator to create such methods.
For example:
class Delft:
def __init__(self, name):
self.name = name
@staticmethod
def fun(abc):
return abc
a = Delft.fun("Stack")
print(a)
Output:
Stack
In the above example, we changed the fun()
function to a static function. This way, we could use it without instantiating any class objects.
Conclusion
This tutorial discussed the TypeError: missing 1 required positional argument: 'self'
error in Python and how we can solve it.
We discussed situations where this error might occur and how to fix the error by instantiating the object of the class. We can also use static methods to work around this error.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python TypeError
- How to Fix the Python TypeError: List Indices Must Be Integers, Not List
- How to Fix TypeError: Iteration Over Non-Sequence
- How to Fix TypeError: Must Be Real Number, Not STR
- How to Solve the TypeError: An Integer Is Required in Python
- How to Fix Python TypeError: Unhashable Type: List
- How to Solve the TypeError: Not All Arguments Converted During String Formatting in Python
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python