How to Change Working Directory in Python
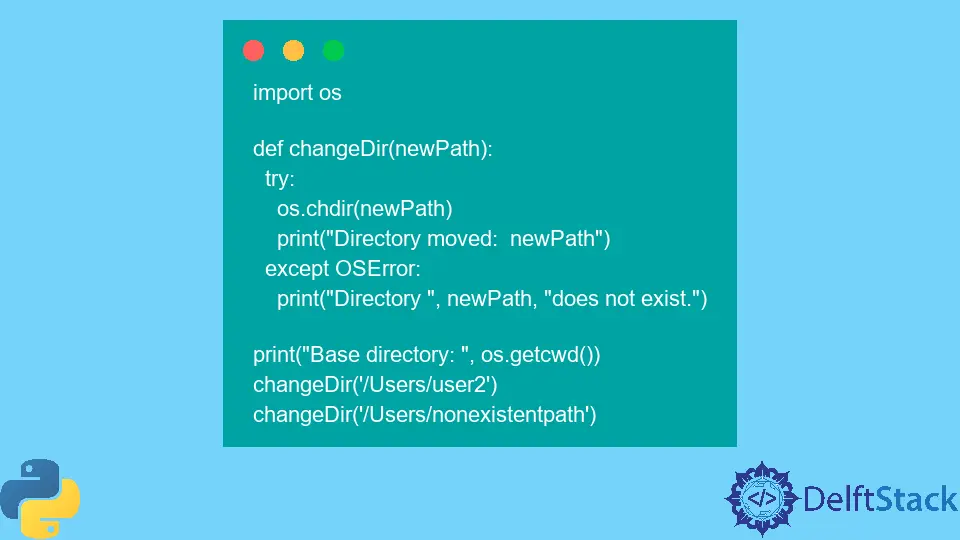
This article discusses how you can change the current working directory from Python into another location.
Use the os
Module to Change Directory in Python
Suppose, for some reason, you want to change your working directory via Python instead of the command console. In that case, the module os
provides tools for miscellaneous operating system utilities directly through Python.
import os
Let’s say you’re currently in a working directory in /Users/user
and want to change to Users/user2
. First, verify the current working directory you’re in by printing out the result of os.getcwd()
. Next is to call the os.chdir("Users/user2")
) block to switch the current working directory.
print(os.getcwd())
os.chdir("/Users/user2")
print(os.getcwd())
Output:
/Users/user
/Users/user2
It verifies that your program has successfully switched working directories from user
to user2
.
Exception Checking
The path is user-inputted, so input errors are very likely. For example, the user inputs a non-existent path; this function will likely be very prone to FileNotFound exceptions. Given that, we should implement exception checking.
Use if-else
to Check for Errors
The simple way to do it is to check if the path specified exists by using if-else
:
import os
def changeDir(newPath):
if os.path.exists(newPath):
os.chdir("Directory moved: ", newPath)
print(os.getcwd())
else:
print("Directory ", newPath, " not found.")
print("Base directory: ", os.getcwd())
changeDir("/Users/user2")
changeDir("/Users/nonexistentpath")
Let’s assume that /Users/user2
is an existing file path, and /Users/nonexistentpath
does not exist.
Output:
Base directory: /Users/user
Directory moved: /Users/user2
Directory Users/nonexistentpath not found.
The first call to the changeDir()
block went through the if
statement because the path exists. On the other hand, the second goes through the else
and prints an error-like message because the path doesn’t exist.
Use try...except
to Check for Errors
If the os.chdir()
command doesn’t find the file path, it will throw a FileNotFoundError
notification. To catch this, we need to wrap the code around a try...except
block.
import os
def changeDir(newPath):
try:
os.chdir(newPath)
print("Directory moved: newPath")
except OSError:
print("Directory ", newPath, "does not exist.")
print("Base directory: ", os.getcwd())
changeDir("/Users/user2")
changeDir("/Users/nonexistentpath")
Output:
Base directory: /Users/user
Directory moved: /Users/user2
Directory Users/nonexistentpath does not exist.
More or less, both produce the same output; however, using the try...except
exception handling is much safer than using an if-else
statement. It’s because user-defined conditions might not cover all the possible exceptions that could occur in tackling file path manipulation.
In summary, the os
module provides extensive support for file and directory manipulation, among the other utilities that it offers.
We can directly change the working directory using the os.chdir()
block, but it would be safer to wrap it around either if-else
or try...except
blocks to avoid exceptions from happening.
If you want to explore more on file manipulation, exception handling and updating file names or content are incredibly important.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedInRelated Article - Python Directory
- How to Get Home Directory in Python
- How to List All Files in Directory and Subdirectories in Python
- How to Fix the No Such File in Directory Error in Python
- How to Get Directory From Path in Python
- How to Execute a Command on Each File in a Folder in Python
- How to Count the Number of Files in a Directory in Python