Python os.chdir() Method
-
Syntax of the
os.chdir()
Method -
Example 1: Use the
os.chdir()
Method to Change the Directory in Python -
Example 2: Use
os.getcwd()
With theos.chdir()
Method in Python -
Example 3: Check Status of New Directory in the
os.chdir()
Method in Python -
Example 4: Set Path Address as Empty in the
os.chdir()
Method in Python
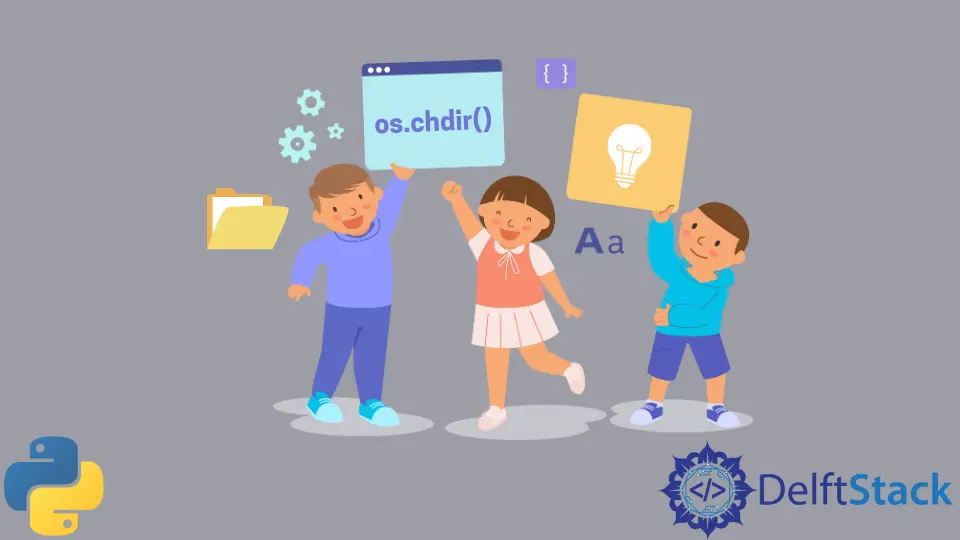
Python os.chdir()
method is the most common way of changing the path of the current working directory to another valid path. This method takes the path of a directory as a parameter and then shifts the current working directory to that new path.
Syntax of the os.chdir()
Method
os.chdir(path)
Parameters
path |
It is an address object of a file system path or a symlink. The address should be the new path for the current directory. |
Return
In the execution process, this method does not return any value.
Example 1: Use the os.chdir()
Method to Change the Directory in Python
import os
os.chdir("/home/user/Desktop")
print("The directory has changed.")
Output:
The directory has changed.
os.chdir()
only works when the parameter path refers to an open directory, not an open file.
Example 2: Use os.getcwd()
With the os.chdir()
Method in Python
import os
directory = os.getcwd()
print(f"Current directory: Before= {directory}")
path = "/usr/"
os.chdir(path)
directory = os.getcwd()
print(f"Current working directory: After= {directory}")
Output:
Current working directory: Before= /home
Current working directory: After= /usr
We can enter an absolute or relative path as a parameter to the os.chdir()
method.
Example 3: Check Status of New Directory in the os.chdir()
Method in Python
import os
directory = "/home/user/Hi"
try:
os.chdir(directory)
print("Your current working directory is : {0}".format(directory))
except FileNotFoundError:
print("The directory: {0} does not exist".format(directory))
except NotADirectoryError:
print("{0} is not a valid directory".format(directory))
except PermissionError:
print("You do not have access to change to {0}".format(directory))
Output:
The directory: /home/user/Hi does not exist
The os.chdir()
method returns errors if the parameter path is not valid.
NotADirectoryError
is raised if the directory is invalid.- If the specified directory has no existence, then a
FileNotFoundError
is thrown. - If the user doesn’t have the necessary permissions to access a directory, a
PermissionError
is thrown.
Example 4: Set Path Address as Empty in the os.chdir()
Method in Python
import os
os.chdir("")
print("Directory has changed.")
Output:
FileNotFoundError: [Errno 2] No such file or directory: ''
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn