Python os.renames() Method
-
Syntax of Python
os.renames()
Method -
Example Codes: Use of the
os.renames()
Method -
Example Codes:
FileNotFoundException
in theos.renames()
Method -
Example Codes: Rename the Specified File and Move to a New Directory With the
os.renames()
Method -
Example Codes:
FileExistsError
Exception When Using theos.renames()
Method -
Example Codes: The
os.renames()
vs.shutil.move()
Methods
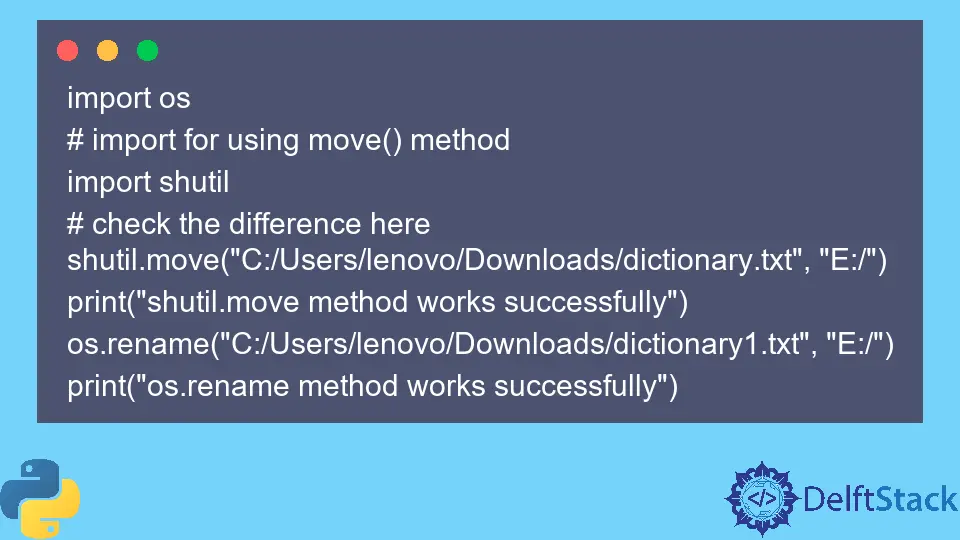
Under the Python standard utility modules, we have the os
module to interact with the operating system.
This article discusses the os.renames()
method used to rename a recursive directory or a file. This method is quite similar in behavior to the os.rename()
method, which does the same, except it also creates the intermediate directories, which need to make the full pathname.
This process is done first by the method before changing the file name. This os.renames()
method does not only change the name of the original file but also deletes the previous (original) file using the os.removedirs()
method.
Syntax of Python os.renames()
Method
os.renames(new, old)
Parameters
old |
It accepts a path-like object and shows the original name of the file or directory to be renamed |
new |
It accepts a path-like object and indicates the new name of the file or directory. This parameter can also include directories that do not exist in the current system file |
Return
This method does not return anything. Instead, it changes the name of the file or directory.
Example Codes: Use of the os.renames()
Method
To demonstrate the concept of the os.renames()
method, the source code below shows all the files inside the source_path
directory.
# importing os module to interact with os methods
import os
# source path to find the file
source_path = "C:/Users/lenovo/Downloads"
# change the directory
os.chdir(source_path)
print("Old file or directory name: ", os.listdir(os.getcwd()))
# to rename a file
os.renames("dictionary.txt", "new_name.txt")
# show newly renamed file
print("Renamed file and directory: ", os.listdir(os.getcwd()))
# to rename a directory
os.renames(source_path + "/remove", source_path + "/create")
print("Folder is successfully renamed")
Output:
Old file or directory name: ['image.jpg', 'slides.pptx', 'dictionary.txt', 'MyApplication.zip']
Renamed file and directory: ['image.jpg', 'slides.pptx', 'new_name.txt', 'MyApplication.zip']
Folder is successfully renamed
Example Codes: FileNotFoundException
in the os.renames()
Method
In the os.renames()
method, if the old
parameter is not a valid file or a directory. Then, the os.renames()
returns an exception FileNotFoundException
in the console.
import os
source_path = "C:/Users/lenovo/Downloads/"
# if the file or directory does not exist
os.renames(source_path + "dictionary.txt", "new_name.txt")
print("Renamed file or directory: ", os.listdir(os.getcwd()))
Output:
FileNotFoundError: [WinError 2] The system cannot find the file specified: 'C:/Users/lenovo/Downloads/dictionary.txt' -> 'new_name.txt'
Example Codes: Rename the Specified File and Move to a New Directory With the os.renames()
Method
If you want to rename a file and move it to a new directory, you can still use the os.renames()
method. The new
parameter inside the os.renames()
method can take the file name only or the directory along with the filename.
If the new directory is not valid or does not exist, the method creates a new directory with the specified name without any error exception.
import os
source_path = "C:/Users/lenovo/Downloads/"
os.chdir(source_path)
print("Old file or directory name: ", os.listdir(os.getcwd()))
# rename the file and move to a new directory
os.renames("dictionary.txt", "remove/new_name.txt")
print("File is renamed, and directory is created successfully.")
Output:
File is renamed, and directory is created successfully.
Example Codes: FileExistsError
Exception When Using the os.renames()
Method
As we have discussed, the os.renames()
method uses two parameters, and the latter is used to rename the specified file. The exception FileExistsError
occurs when a file with the same name as the provided renamed file already exists.
The method returns the exception but does not override the same file. The below example shows how such an exception occurs and how to handle it.
import os
source_path = "C:/Users/lenovo/Downloads/"
os.chdir(source_path)
# if new_name.txt already exist in specified source_path
os.renames("dictionary.txt", "new_name.txt")
print(os.listdir(os.getcwd()))
print("File renamed successfully.")
Output:
FileExistsError: [WinError 183] Cannot create a file when that file already exists: 'dictionary.txt' -> 'new_name.txt'
To solve such a situation, we can either:
- Rename the existing file with the same name (by the
rename()
method). - Remove the file if it already exists (by the
remove()
method). - Change the directory of the file using exception handling.
Example Codes: The os.renames()
vs. shutil.move()
Methods
The os
module might fail when the source and destination paths are on different system drives. The os.renames()
method returns the OSError
exception, showing that the system cannot move the file, while the shutil.move()
method checks whether the source and destination paths are on different drives.
If the destination path is different, the method makes sure to copy the file to another drive and delete it from its original location. Hence, proving that the os.renames()
method works on low-level functions and the shutil.move()
method works on higher-level functions.
import os
# import for using move() method
import shutil
# check the difference here
shutil.move("C:/Users/lenovo/Downloads/dictionary.txt", "E:/")
print("shutil.move method works successfully")
os.rename("C:/Users/lenovo/Downloads/dictionary1.txt", "E:/")
print("os.rename method works successfully")
Output:
# program works fine for shutil.move() method
shutil.move method works successfully
# program throws exception on os.renames() method
OSError: [WinError 17] The system cannot move the file to a different disk drive: 'C:/Users/lenovo/Downloads/dictionary1.txt' -> 'E:/'
the invalid cross-device link
Exception Error
Sometimes, when using the os.renames()
method and defining source and destination paths differently, the user may face a different exception, for example, an invalid cross-device link
.
To solve this exception, we need to use the move()
method inside the shutil
module as proposed above. The os
module is discouraged when we want to work in a different system drive.
Hi, I'm Junaid. I am a freelance software developer and a content writer. For the last 3 years, I have been working and coding with Python. Additionally, I have a huge interest in developing native and hybrid mobile applications.
LinkedIn