Python os.get_inheritable Method
-
Syntax of Python
os.get_inheritable()
Method -
Example 1: Use the
os.get_inheritable()
Method to Get Value of File Descriptor’sinheritable
Flag -
Example 2: Create the Child Process With the
os.get_inheritable()
Method -
Example 3:
os.get_inheritable()
VSsocket.get_inheritable()
Method
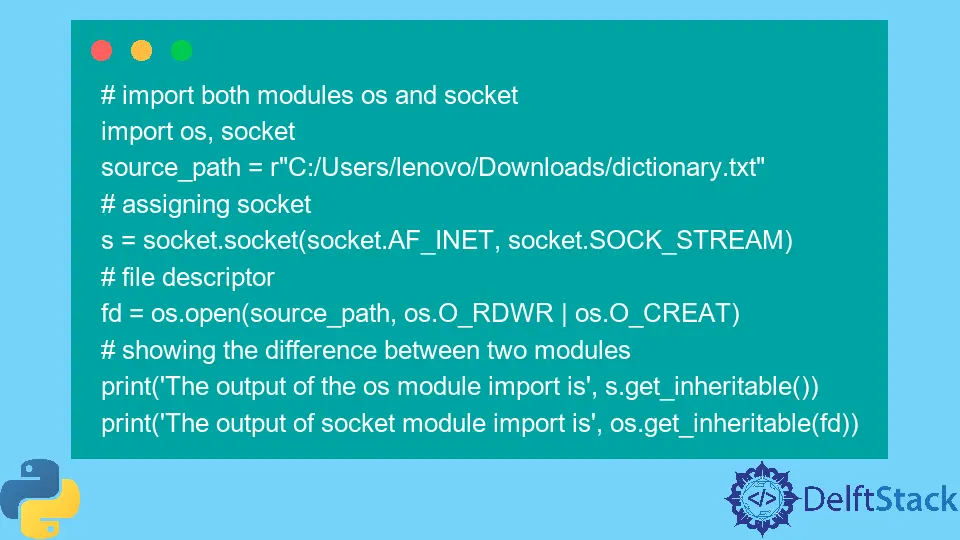
In Python, the OS module directly links with the standard utility module of Python. We can use operating system functions and methods by using this os
module.
One of the methods inside this module is os.get_inheritable()
, which gets the inheritable
flag value of the provided file descriptor.
These types of inheritable
flags tell us about the flag descriptor can be inherited by the child processes or not. This method is available on all operating systems.
Let’s take an example to understand the working of the inheritable
flag. For example, if the parent process creates a child process and the parent process has a file descriptor (let’s say 10), then the child process has the same file descriptor 10 for the same file.
This happens when the inheritable
flag of the fd
is set in the parent process.
Syntax of Python os.get_inheritable()
Method
os.get_inheritable(fd)
Parameter
fd |
a flag descriptor parameter for checking the inheritable flag. |
Return
This method returns the Boolean value, either True
or False
, for the inheritable
flag of the provided file descriptor.
Example 1: Use the os.get_inheritable()
Method to Get Value of File Descriptor’s inheritable
Flag
In this example, we work on using the os
module method os.get_inheritable()
. From the previous section, we know that this method is used to get the value of the inheritable
flag of the file descriptor fd
.
Now, let’s see an example to show how we can use this method in the source code. In the below example, we can see that we can also set the value of the inheritable
flag by the use of the os.set_inheritable()
method.
The method set_inheritable()
takes two parameters unlike the os.get_inheritable()
method.
# importing the os module to use its methods
import os
# soruce File path
source_path = r"C:/Users/lenovo/Downloads/dictionary.txt"
# using the os.open() method to open and get the file descriptor associated with it
file_descriptor = os.open(source_path, os.O_RDWR | os.O_CREAT)
# using os.get_inheritable() method to get the value of inheritable flag of fd
flag = os.get_inheritable(file_descriptor)
# print the Boolean value here
print("The inheritable flag value of the file descriptor fd is ", flag)
# using os.set_inheritable() method to set or unset the inheritable flag value
os.set_inheritable(file_descriptor, True)
flag = os.get_inheritable(file_descriptor)
print("The inheritable flag value of the file descriptor fd is ", flag)
Output:
The inheritable flag value of the file descriptor fd is False
The inheritable flag value of the file descriptor fd is True
Example 2: Create the Child Process With the os.get_inheritable()
Method
In the above example, we know that the method os.get_inheritable()
is used to get the inheritable
flag of the file descriptor. Look at the example below; we use the os.pipe()
method to create a pipe, which returns a pair of file descriptors read and write.
We pass these read and write file descriptors to the os.get_inheritable()
method as a parameter. Then we create the child process using the os.fork()
method.
We use the open()
method to write and read the file descriptors. Let’s see the source code demo below to further demonstrate this example.
import os
# pipe() method to create a pair of file descriptors, i.e., read and write
fd_read, fd_write = os.pipe()
# pass read and write fd to the os.get_inhertiable() method
print("The value of reading file descriptor is ", fd_read, os.get_inheritable(fd_read))
print(
"The value of writing file descriptor is ", fd_write, os.get_inheritable(fd_write)
)
# create the child process
child_pid = os.fork()
if child_pid == 0:
# method to close the provided fd. No longer refers to any file or resource
os.close(fd_read)
# write to a child process; if nothing is written
with open(fd_write, "w") as write_end:
# fd write_end uses write() method to write the given argument as string type
write_end.write("the child process is here ")
else:
os.close(fd_write)
# this method waits for a process's completion using the handle pid and returns the tuple data type.
os.waitpid(child_pid, 1)
# read from child process
with open(fd_read) as read_end:
message = read_end.read()
print("coming from child ", message)
Output:
The value of reading file descriptor is 6 False
The value of writing file descriptor is 7 False
The value of reading file descriptor is 6 False
The value of writing file descriptor is 7 False
coming from child the child process is here
Example 3: os.get_inheritable()
VS socket.get_inheritable()
Method
To differentiate between the same methods of different modules, os
and socket
. We need to import both the modules at the start and then use its method get_inheritable()
.
The os.get_inheritable
method is used to get the inheritable
flag of the provided file descriptor. While the method socket.get_inheritable()
gets the inheritable
flag of the socket handle or the socket file descriptor.
If we can inherit the child process, it returns True
; otherwise, it returns False
if we cannot inherit the child process. Let’s see an example to demonstrate the difference between the same methods with different imports.
# import both modules os and socket
import os
import socket
source_path = r"C:/Users/lenovo/Downloads/dictionary.txt"
# assigning socket
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
# file descriptor
fd = os.open(source_path, os.O_RDWR | os.O_CREAT)
# showing the difference between two modules
print("The output of the os module import is", s.get_inheritable())
print("The output of socket module import is", os.get_inheritable(fd))
Output:
The output of the os module import is False
The output of socket module import is False