Python os.stat_result Class
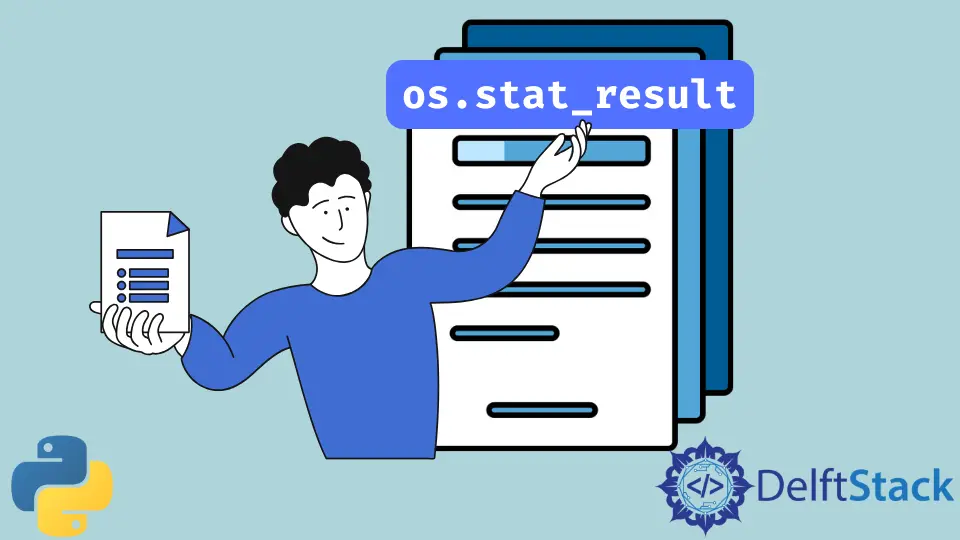
The stat_result
class belongs to the os
module. The os
is a built-in module in Python programming language that offers various utilities to interact with the operating system.
The stat_result
class represents a state of a file descriptor. Simply put, it stores details about a file such as last update time, creation time, owner’s name, file mode and type, etc.
This article will discuss the os.stat_result
class in detail, along with some examples.
Syntax
os.stat_result
Parameters
Since it is a class, it does not have any parameters.
Returns
Since it is a class, it does not return anything.
Attributes
Attribute | Platform | Explanation |
---|---|---|
st_mode |
All | The file type and the file mode bits or permission details. |
st_ino |
All | The inode number on Unix systems and the file index on Windows systems. |
st_dev |
All | The identifier of the device on which a file lives. |
st_nlink |
All | The number of hard links. |
st_uid |
All | The user identifier of the file owner. |
st_gid |
All | The group identifier of the file owner. |
st_size |
All | The file size in bytes. In the case of a symbolic link, it is the length of the file system path it stores. |
st_atime |
All | The most recent access time in seconds. |
st_mtime |
All | The time of the most recent modification in seconds. |
st_ctime |
All | The time of most recent metadata modification in seconds on Unix systems and the time of creation on Windows systems in seconds. |
st_atime_ns |
All | The time of most recent access in nanoseconds. |
st_mtime_ns |
All | The time of the most recent modification in nanoseconds. |
st_ctime_ns |
All | The time of most recent metadata modification in nanoseconds on Unix systems and the time of creation on Windows systems in nanoseconds. |
st_blocks |
Unix | The number of 512-byte blocks allocated for the file. If the file has holes, it might be smaller than st_size / 512 . |
st_blksize |
Unix | The preferred block size for efficient file system input-output operations. Note that writing to a file in small chunks may cause inefficient read-modify-rewrite. |
st_rdev |
Unix | The type of device, in case of an inode device. |
st_flags |
Unix | The user-defined flags for the file. |
st_gen |
Unix | The file generation number. |
st_birthtime |
Unix | The time of creating the file. |
st_fstype |
Solaris | A string that uniquely identifies the type of file system that stores the file. |
st_rsize |
macOS | The real size of the file. |
st_creator |
macOS | The creator of the file. |
st_type |
macOS | The file type. |
st_file_attributes |
Windows | The dwFileAttributes member of the BY_HANDLE_FILE_INFORMATION structure returned by the GetFileInformationByHandle() method. |
st_reparse_tag |
Windows | When the st_file_attributes has the FILE_ATTRIBUTE_REPARSE_POINT set, this attribute stores the tag identifying the type of reparse point. |
Example: Status of a File
Code Snippet:
import os
path = os.getcwd() + "/file.txt"
fd = os.open(path, os.O_RDONLY)
print(os.stat(fd))
Output:
os.stat_result(
st_mode=33206,
st_ino=1407374884353234,
st_dev=503946240,
st_nlink=1,
st_uid=0,
st_gid=0,
st_size=242,
st_atime=1662906051,
st_mtime=1662906051,
st_ctime=1662906023
)
First, create a file file.txt
in the current working directory and add some random data. The Python code first defines a path
to this file, and using the os.open()
method, it opens the file and creates a file descriptor.
Next, using the os.stat()
and the file descriptor, it gets the status of the file and prints the output. The os.stat()
method returns a stat_result
object.
Note that, instead of os.stat()
, one can also use os.fstat()
method because it also gets the same result.