Python os.set_handle_inheritable() Method
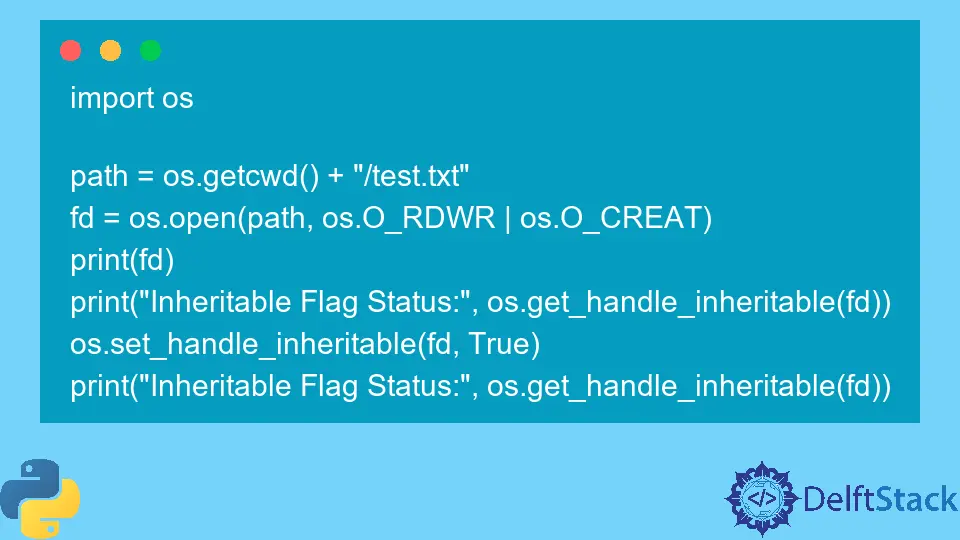
The Python programming language offers an os
library that lets us interact with the system’s operating system and perform many low-level operations such as file management, environment-related tasks, reading files, spawning new threads and processes, etc.
Whenever a resource is opened, a file descriptor is associated with that file. A file descriptor is essentially an integer value uniquely identifying an open file on a system.
The UNIX system is known as a file descriptor, while on the flip side, it is commonly termed a handler on Windows systems.
A file descriptor or a handler has an inheritable
flag that signifies if any child processes can inherit that file descriptor. Every file descriptor or handler is set as non-inheritable by default.
On UNIX and Windows systems, non-inheritable file descriptors or handlers are closed inside child processes except for inheritable ones.
The os
module offers a set_handle_inheritable()
method that we can use to set an inheritable
flag of a valid handler to a boolean value. Note that we can only use it if we are working on Windows operating system.
Syntax
os.set_handle_inheritable(handle, inheritable)
Parameters
Type | Explanation | |
---|---|---|
handle |
Integer | A handler associated with a valid resource. |
inheritable |
Boolean | A boolean value for the inheritable attribute. |
Returns
The set_handle_inheritable()
method does not return anything. It sets the inheritable
flag of a handler to a boolean value on a Windows machine.
Example Code: Set the inheritable
Flag for a File
Code Snippet:
import os
path = os.getcwd() + "/test.txt"
fd = os.open(path, os.O_RDWR | os.O_CREAT)
print(fd)
print("Inheritable Flag Status:", os.get_handle_inheritable(fd))
os.set_handle_inheritable(fd, True)
print("Inheritable Flag Status:", os.get_handle_inheritable(fd))
Output:
Inheritable Flag Status: False
Inheritable Flag Status: True
The Python code above first defines a path
to a file. It can be a file system path
to any file, but the above code considers a file test.txt
in the current working directory.
The os.getcwd()
method returns a string representation for the current working directory. Next, we open the file using the os.open()
method.
Note that if the file is missing, it is created and ultimately opened in read and write mode. Currently, the file descriptor is inheritable
(refer to the first line of the output).
Lastly, we set the inheritable
flag to True
. The second line in the output depicts the same. Note that the get_handle_inheritable()
method returns True
if the child can inherit the handle and False
otherwise.
Similarly, set_handle_inheritable()
sets an inheritable
flag of the given handle. You can dig more about these methods here.