Python os.get_handle_inheritable Method
- File and Directory Descriptor in Python
- Understanding File Handle Inheritance
-
Purpose of Python
os.get_handle_inheritable
Method -
Syntax of Python
os.get_handle_inheritable()
Method -
Example 1: Use the
os.get_handle_inheritable()
Method in Python -
Example 2: Explore the
os.get_handle_inheritable()
Method Inside theos
Module -
Example 3: Use
os.get_handle_inheritable
to Check if File Handle Is Inheritable - Practical Use Cases
- Conclusion
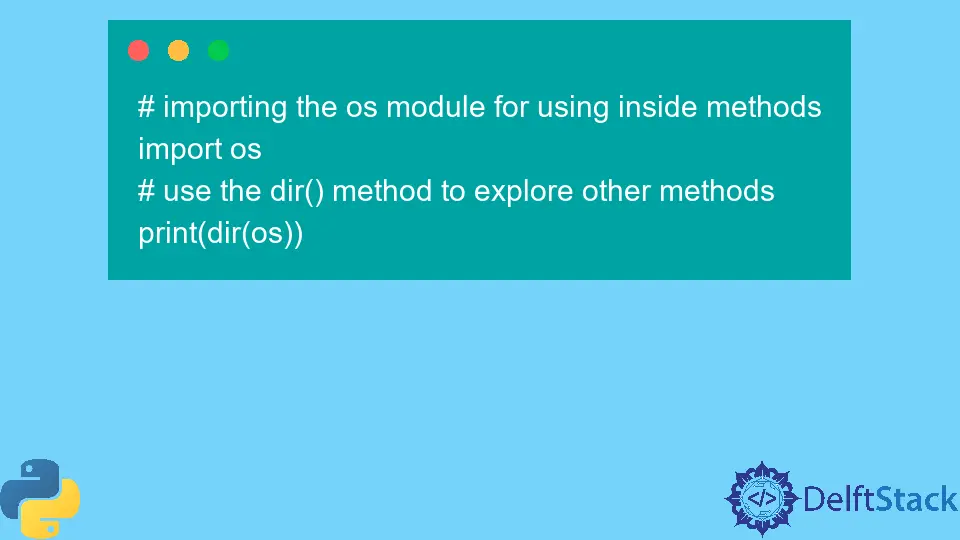
In Python, the OS
module provides several methods to work and communicate with the operating system. The os
import includes and inherits many methods, which help us do different operations with the operating system.
One of the methods is os.get_handle_inheritable()
, which gets the inheritable
flag of the specified handle. The official Python site says this method is only available on Windows.
Python has the os.get_inheritable()
method for descriptors and the os.get_handle_inheritable()
method for handles. The method is used for socket handles in a factor.
Now, the question here is, what are a file and a directory descriptor?
File and Directory Descriptor in Python
To communicate directly with the OS kernel, we have low-level file descriptors. These file descriptors are in the form of an integer that finds the open file in a table of all open files stored by the kernel of each process.
Several system calls can accept file descriptors (that are recommended to interact with) that have:
- Manual error handling
- Several retries in a few conditions
- Requires fixed-sized buffers
To make interacting with files convenient and with less error, we need Python classes that are file objects that wrap file descriptors.
If you see a file descriptor referring to a directory, then dir_fd
isn’t None
, and the operational path is relative to that directory. The dir_fd
is only ignored if the path is absolute.
We can also check the availability and support of the dir_fd
by using the command os.supports_dir_fd
on our platform. The platform will show and raise an error NotImplementedError
if dir_fd
is unavailable.
Symlinks
The symbolic link will operate on a method if the last item of the path to work on is a symbolic link and if follow_symlinks
is False
.
Similar to the file and directory descriptor availability for a particular platform, we can also check the follow_symlinks
support on our platform for a method by using the command os.supports_follow_symlinks
.
The exception NotImplementedError
will be raised if the symlinks
are unavailable.
Implementation
During implementation, if we want to check whether the provided path can be known as a file descriptor for a particular method on your operating system, we can use os.supports_fd
method.
If it is unavailable for your operating system, an exception will be raised by showing the NotImplementedError
exception.
Understanding File Handle Inheritance
Before diving into the intricacies of the os.get_handle_inheritable
method, it is essential to grasp the concept of file handle inheritance. In the context of operating systems, a file handle is a mechanism used to access files or resources.
When a new process is created, it may inherit file handles from its parent process. In Python, the os
module provides tools to manage the inheritance of file handles between parent and child processes.
Purpose of Python os.get_handle_inheritable
Method
The primary purpose of os.get_handle_inheritable
is to retrieve the inheritance flag of a given file handle. The method takes a file handle as an argument and returns a Boolean value indicating whether the file handle is marked as inheritable or not.
If a file handle is inheritable, child processes will receive and use it; otherwise, they won’t inherit it.
Syntax of Python os.get_handle_inheritable()
Method
os.get_handle_inheritable(handle)
Parameters
handle
- A file handle whose inheritable status needs to be checked. These are file handles having datatype integers containing 0, 1, or 2. This is a single parameter of the get_handle_inheritable()
method.
Return Value
This method does not return anything; instead, it gets the inheritable
flag, a Boolean value of either True
or False
.
Example 1: Use the os.get_handle_inheritable()
Method in Python
# This is how you can use the os.get_handle_inheritable() method
def get_inheritable(self):
return os.get_handle_inheritable(self.fileno())
The method os.get_handle_inheritable()
gets the file descriptor’s inherited flag. This flag shows and highlights whether the descriptor can be inherited by its child processes or not.
In Python version 3.4 (by default), the file descriptor created by Python is non-inherited.
Windows:
In child processes, file handles and non-inherited handles are closed except only for the original streams that are always inherited (the file handles 0, 1, and 2, i.e., stdin
, stdout
and stderr
).
Unix:
When a new program is executed, the non-inherited file descriptors are closed in the child processes. In contrast, the other (file descriptors) are inherited.
Example 2: Explore the os.get_handle_inheritable()
Method Inside the os
Module
As we have discussed, the os
module provides different methods to work with if you forget or want to explore all the methods inside the os
module.
You can always use the dir()
method inside the print
statement to output all these methods. See the source code below to find the different methods and the os.get_handle_inheritable()
method.
# importing the os module for using inside methods
import os
# use the dir() method to explore other methods
print(dir(os))
Output:
Example 3: Use os.get_handle_inheritable
to Check if File Handle Is Inheritable
Let’s consider a scenario where a Python script opens a file and spawns a child process. We can use os.get_handle_inheritable
to check if the file handle is inheritable or not.
import os
# Open a file
file_handle = os.open("example.txt", os.O_RDWR)
# Check if the file handle is inheritable
inheritable = os.get_handle_inheritable(file_handle)
print(f"The file handle is inheritable: {inheritable}")
# Close the file handle
os.close(file_handle)
Output:
The file handle is inheritable: False
In this example, os.open
is used to open a file, and os.get_handle_inheritable
is employed to determine whether the file handle is inheritable or not. The result is then printed to the console.
Note that this code will only work on Python 3.10 versions and later on Windows OS.
Practical Use Cases
-
Secure File Handling: In scenarios where sensitive information is being processed, ensuring that child processes do not inherit file handles is crucial for security.
os.get_handle_inheritable
can be used to verify and control the inheritance status of file handles. -
Interprocess Communication: In multiprocessing applications, controlling which file handles are inherited can be essential for effective interprocess communication. By selectively marking file handles as inheritable or not, developers can manage data flow between processes.
-
Resource Management: Python scripts dealing with numerous file handles can utilize
os.get_handle_inheritable
to dynamically control which resources are passed down to child processes. This can optimize resource usage and prevent unnecessary inheritance.
Conclusion
The os.get_handle_inheritable
method in Python’s os
module is a powerful tool for managing file handle inheritance between parent and child processes. Understanding and utilizing this method can significantly enhance the security, efficiency, and resource management capabilities of Python scripts, particularly in scenarios involving multiprocessing or interprocess communication.