Python os.supports_fd Method
-
Introduction to
os.supports_fd
in Python -
Syntax of the Python
os.supports_fd
Method -
Example 1: Checking
os.supports_fd
Support in Python -
Example 2: Use the
os.supports_fd
Method in Python -
Example 3: Use the
os.supports_fd
Method on a Few Methods in Python - Practical Applications
- Considerations and Best Practices
- Conclusion
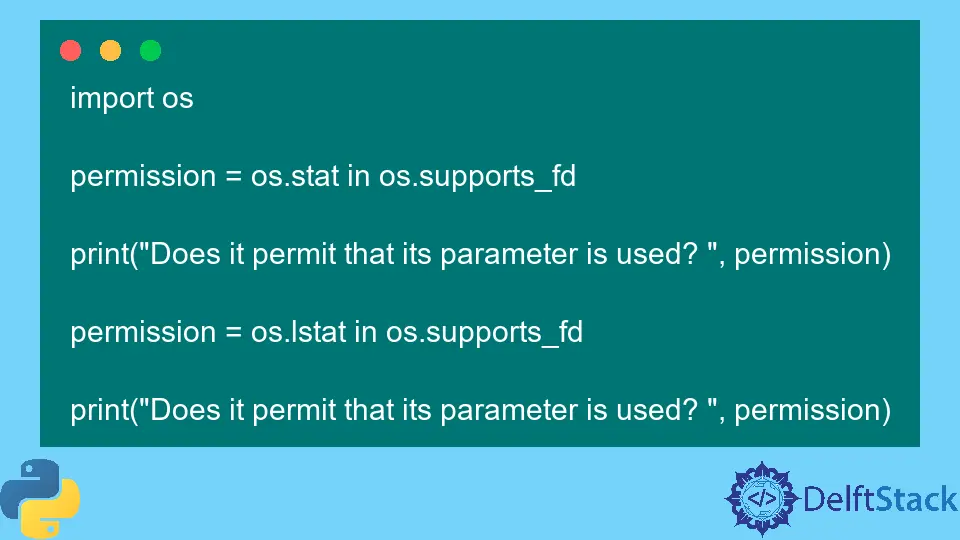
The Python os
module is a versatile library that allows interaction with the underlying operating system. Among its functionalities is the os.supports_fd
method, which serves as a valuable tool for checking if the system supports file descriptor-based operations.
Python’s os.supports_fd
method is an efficient way of checking if a specific OS module allows a method to use its path parameter as an open fd
or not. Many platforms provide various functionality, so open fd
as path arguments functionality needs to be checked.
In this article, we will explore the purpose, functionality, and practical applications of os.supports_fd
in Python.
Introduction to os.supports_fd
in Python
The os
module in Python provides a platform-independent way to interact with the underlying operating system. One of the functions it offers is the os.supports_fd
, which allows you to determine whether the system supports file descriptor-based operations.
Understanding File Descriptors
Before delving into the details of os.supports_fd
, it’s essential to grasp the concept of file descriptors.
In Unix-like operating systems, everything is treated as a file, including regular files, directories, sockets, pipes, and more. Each open file is assigned a unique integer called a file descriptor.
File descriptors are crucial for performing I/O operations, as they serve as an abstraction that allows programs to interact with files and other I/O resources without having to understand the intricacies of the underlying hardware or filesystem. This abstraction simplifies the process of reading from or writing to files, regardless of the specific device or medium.
the Significance of os.supports_fd
os.supports_fd
serves as a method to check if the system supports file descriptor-based operations. This can be particularly useful when you need to perform operations on files using file descriptors, which can provide certain advantages in specific scenarios.
Syntax of the Python os.supports_fd
Method
os.supports_fd
Parameter
It is a non-callable object, so no parameter is needed.
Return
The return type of this method is a set object representing all the methods in the OS module that allows their path argument to be used as an open file descriptor.
Example 1: Checking os.supports_fd
Support in Python
Using os.supports_fd
is straightforward. The function returns True
if the system supports file descriptor-based operations and False
otherwise.
import os
supports_fd = os.supports_fd
if supports_fd:
print("The system supports file descriptor-based operations.")
else:
print("The system does not support file descriptor-based operations.")
This code checks if the system supports file descriptor-based operations and prints an appropriate message based on the result. If os.supports_fd
is True
, it means the system supports file descriptor-based operations, and if it’s False
, it means the system does not support them.
Output:
The system supports file descriptor-based operations.
Example 2: Use the os.supports_fd
Method in Python
This code checks and prints the set of methods in the os
module that allow their path parameter to be used as an open file descriptor. The output will be a list of methods, and this information can be useful for developers who want to understand which methods support using file descriptors as path parameters.
import os
List = os.supports_fd
print(
"Following are the method that allows their path parameter to be used as an open fd: ",
List,
)
Output:
Following are the method that allows their path parameter to be used as an open fd: {<built-in function utime>, <built-in function chmod>, <built-in function listdir>, <built-in function stat>, <built-in function pathconf>, <built-in function scandir>, <built-in function statvfs>, <built-in function chdir>, <built-in function truncate>, <built-in function chown>, <built-in function execve>}
Note that to determine whether any Python method permits using an open file descriptor for its path parameter, we can use the in
operator on the supports_fd
set.
Example 3: Use the os.supports_fd
Method on a Few Methods in Python
This code checks if the os.stat
and os.lstat
functions can be used with file descriptor-based operations. It then prints out whether each of these functions is supported.
The result will be True
if the function is supported and False
otherwise.
import os
permission = os.stat in os.supports_fd
print("Does it permit its parameter to be used? ", permission)
permission = os.lstat in os.supports_fd
print("Does it permit its parameter to be used? ", permission)
Output:
Does it permit its parameter to be used? True
Does it permit its parameter to be used? False
In the code, we have checked whether the os.stat()
and os.lstat
methods allow its path parameter to be used as an open file descriptor.
Practical Applications
Efficient File Operations
If the system supports file descriptor-based operations, you can utilize file descriptors to perform operations on files. This can lead to more efficient and optimized file handling in certain situations.
Avoiding Race Conditions
Using file descriptors for file operations can help in avoiding race conditions, as file descriptors provide a more atomic way to interact with files.
Improved Resource Management
File descriptors can be advantageous for managing resources, especially in scenarios where you need precise control over file operations.
Considerations and Best Practices
- Platform Compatibility: Keep in mind that the availability of file descriptor-based operations may vary depending on the underlying operating system.
- Fallback Mechanism: If
os.supports_fd
returnsFalse
, be prepared to implement an alternative approach for file operations. - Use Case Specific: Utilize file descriptor-based operations when they offer clear advantages in terms of performance or resource management.
Conclusion
os.supports_fd
is a valuable tool for determining if the system supports file descriptor-based operations. By understanding and leveraging this function, you can make informed decisions about how to perform file operations efficiently and with precise control.
While not all systems may support file descriptor-based operations, having the capability to check for support allows you to implement fallback mechanisms or alternative strategies as needed. This function empowers Python developers to navigate file systems with greater flexibility and efficiency.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn