Python os.set_inheritable() Method
-
Introduction to the
os.set_inheritable()
in Python -
Syntax of the Python
os.set_inheritable()
Method -
Example 1: Use the
os.set_inheritable()
to Set theinheritable
Flag for a File in Python -
Example 2: Use the
os.set_inheritable()
to Set thenon-inheritable
Flag for a File in Python - Practical Applications
- Considerations and Best Practices
- Conclusion
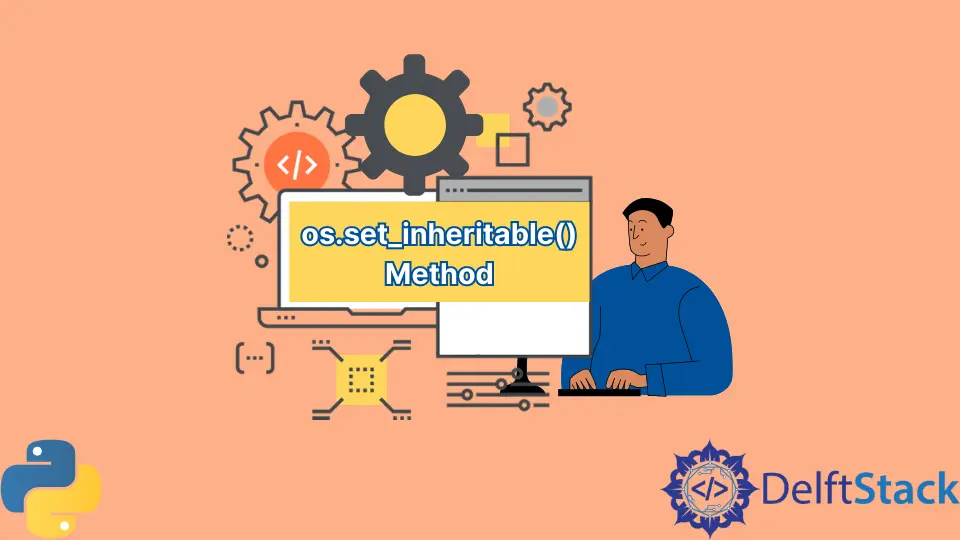
The Python programming language offers an os
module to interact with the operating system and perform various low-level operations such as reading files, spawning new processes, file management, environment operations, etc. Among these, the os.set_inheritable()
method is a crucial tool for managing file descriptor inheritance within processes.
Whenever a file is opened, a file descriptor is associated with that file. A file descriptor is essentially an integer value uniquely identifying an open file on a system.
A file descriptor has an inheritable
flag that indicates if any child process can inherit that file descriptor. By default, all the file descriptors are set as non-inheritable
.
On UNIX and Windows systems, non-inheritable
file descriptors are closed inside child processes except for inheritable
file descriptors.
The os
module has a set_inheritable()
method that we can use to set the inheritable
flag of a valid file descriptor to a Boolean value. In this article, we will explore the purpose, functionality, and practical applications of os.set_inheritable()
.
Introduction to the os.set_inheritable()
in Python
The os
module in Python is a powerful library for system interactions. One of its key functions, os.set_inheritable()
, enables developers to control whether child processes inherit a file descriptor.
Understanding File Descriptors
Before diving into os.set_inheritable()
, it’s crucial to have a solid understanding of file descriptors.
In Unix-like systems, a file descriptor is a unique non-negative integer associated with an open file, socket, or other I/O resource. It serves as a reference to the resource.
File Descriptor Inheritance
When a new process is created in Unix-based systems, it often inherits file descriptors from its parent process. This inheritance allows the child process to access the same resources (like files or sockets) as its parent.
However, there are scenarios where you may want to control which file descriptors are inherited.
the Role of the os.set_inheritable()
os.set_inheritable(fd, inheritable)
is a method that allows you to explicitly set whether a file descriptor (fd
) should be inherited by child processes. The inheritable
argument is a Boolean value (True
or False
) indicating whether the file descriptor should be inherited.
Syntax of the Python os.set_inheritable()
Method
os.set_inheritable(fd, inheritable)
Parameters
Type | Explanation | |
---|---|---|
fd |
Integer | It is a file descriptor associated with a valid resource. The file descriptor you want to control. |
inheritable |
Boolean | A Boolean value (True or False ) indicating whether the file descriptor should be inherited. |
Returns
The set_inheritable()
method does not return anything. Instead, it sets a file descriptor’s inheritable
flag to a Boolean value.
Example 1: Use the os.set_inheritable()
to Set the inheritable
Flag for a File in Python
This code snippet demonstrates the use of the os.get_inheritable()
and the os.set_inheritable()
methods.
import os
path = os.getcwd() + "/file.txt"
fd = os.open(path, os.O_RDWR | os.O_CREAT)
print("Inheritable Flag Status:", os.get_inheritable(fd))
os.set_inheritable(fd, True)
print("Inheritable Flag Status:", os.get_inheritable(fd))
Output:
Inheritable Flag Status: False
Inheritable Flag Status: True
The Python code above first defines a path
to a file. It can be a file system path
to any file, but the above code considers a file file.txt
in the current working directory.
The os.getcwd()
method returns a string representation for the current working directory. Next, we open the file using the os.open()
method.
Note that if the file is missing, it is created and ultimately opened in read and write mode. Currently, the file descriptor is inheritable
(refer to the first line of the output)
Lastly, we set the inheritable
flag to True
. The second line in the output depicts the same.
Example 2: Use the os.set_inheritable()
to Set the non-inheritable
Flag for a File in Python
Let’s go through another example to demonstrate how to use the os.set_inheritable()
. Below is a simple example of how to use the os.open()
function to open a file and then use the os.set_inheritable()
to mark the file descriptor as non-inheritable
(set to False
).
import os
# Open a file
file_descriptor = os.open("myfile.txt", os.O_RDONLY)
# Set the file descriptor as non-inheritable
os.set_inheritable(file_descriptor, False)
In this example, we first open a file called myfile.txt
in read-only mode, obtaining a file descriptor. Next, we use the os.set_inheritable()
to mark this file descriptor as non-inheritable
.
The purpose of setting a file descriptor as non-inheritable
is to ensure that it is not passed down to any child processes that may be spawned later in the program. This can be important for managing resources and ensuring that child processes do not accidentally access or modify files that they shouldn’t.
It’s worth noting that in practice, you would typically perform additional operations on the file using its file descriptor after it has been opened and then later close the file descriptor when it is no longer needed to release system resources.
Practical Applications
Controlling File Descriptor Inheritance
By using the os.set_inheritable()
, you can precisely control which file descriptors are inherited by child processes. This can be crucial in scenarios where you want to restrict access to certain resources or prevent unintended interactions.
Managing Communication Channels
In scenarios where inter-process communication is required, you can use the os.set_inheritable()
to carefully manage the file descriptors used for communication, ensuring they are only accessible to the intended processes.
Considerations and Best Practices
- Resource Security: Use
os.set_inheritable()
to limit access to sensitive resources, enhancing security in multi-process environments. - Clear Documentation: Clearly document which file descriptors are set as
inheritable
and which are not, especially in complex applications.
Conclusion
os.set_inheritable()
is a powerful method for controlling file descriptor inheritance in Python. By understanding and leveraging this function, you gain precise control over which resources are accessible to child processes.
This is invaluable in scenarios where security and controlled resource access are paramount. By implementing this method effectively, you can design robust and secure multi-process applications.