Python os.getcwd() Method
-
Syntax of the
os.getcwd()
Method -
Example Codes: Working With the
os.getcwd()
Method in Python -
Example Codes: Create a New File and Use the
os.getcwd()
Method in Python -
Example Codes: Check the Return Type of the
os.getcwd()
Method in Python
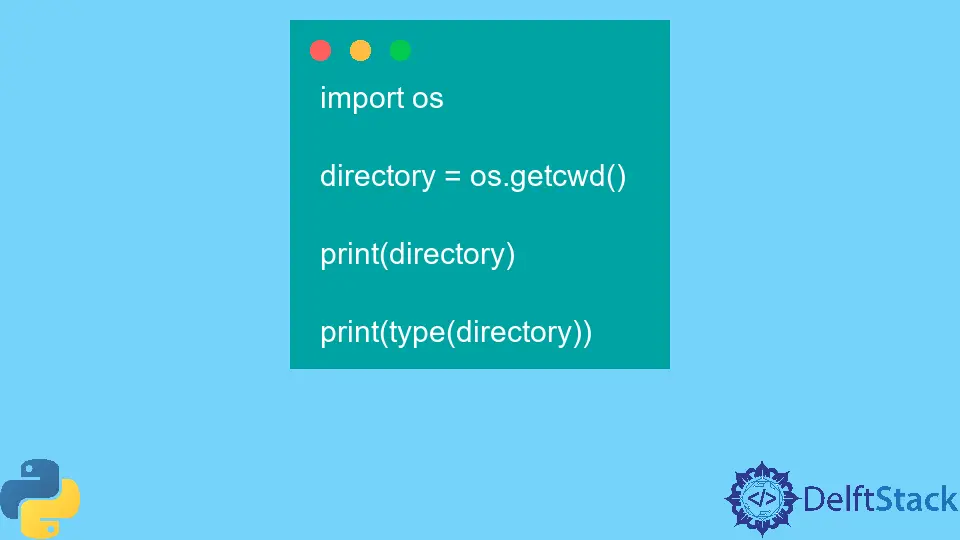
Python os.getcwd()
method is an efficient way of knowing the location of the current working directory of any process. Every process executing on an OS has an associated working directory.
Syntax of the os.getcwd()
Method
os.getcwd()
Parameters
This method does not take any parameters.
Return
The return type of this method is a path string containing the path address of the current working directory.
Example Codes: Working With the os.getcwd()
Method in Python
import os
directory = os.getcwd()
print("Your current working directory is:", directory)
Output:
Your current working directory is: /home
A file descriptor fd
is an integer value that is abstract in nature and acts as a handle to perform various lower-level I/O operations like read
, write
, send
, etc.
Example Codes: Create a New File and Use the os.getcwd()
Method in Python
import os
print("Your current working directory is:")
print(os.getcwd())
file_name = "File.txt"
create = open(file_name, "w+")
print("The full path of the new file is:")
print(os.path.realpath(file_name))
os.mkdir("New_Directory")
os.chdir("New_Directory")
print("Your current working directory after changing it is:")
print(os.getcwd())
Output:
Your current working directory is:
/home
The full path of the new file is:
/home/File.txt
Your current working directory after changing it is:
/home/New_Directory
Note that the os.getcwd()
method returns an absolute path of the current working directory. It has a similar working to os.path.abspath()
.
If you require the entire path address, use the os.path.realpath()
method.
Example Codes: Check the Return Type of the os.getcwd()
Method in Python
import os
directory = os.getcwd()
print(directory)
print(type(directory))
Output:
/home
<class 'str'>
If no process or file is in the OS, then a FileNotFoundError
is returned by this method. In other cases, if the user doesn’t have the necessary permissions to access a directory, then a PermissionError
is returned.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn