Python os.path.realpath() Method
-
Syntax of Python
os.path.realpath()
: -
Example Codes: Use the
os.path.realpath()
Method to Obtain the Canonical Path -
Example Codes: Difference Between the
os.path.realpath()
andos.path.abspath()
Methods -
Example Codes: Difference Between the
os.path.realpath()
andPath.resolve()
Methods
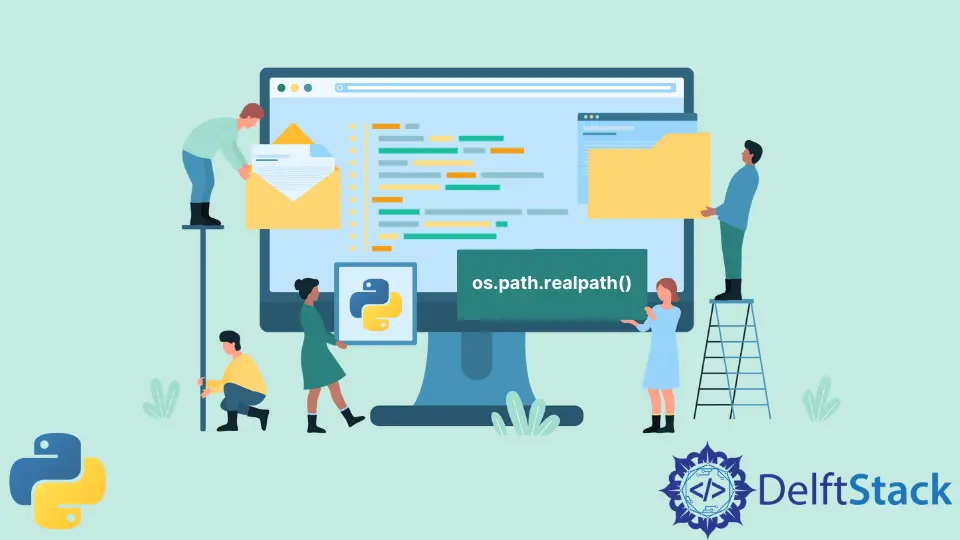
We use several different OS module methods in Python to work with the OS path files. These methods provide us with the facility to manipulate file paths.
One of the methods is os.path.realpath()
, which provides us with the canonical path of the provided file name. The canonical path means the unique path from the file system’s root; this can also be an absolute path but not vice versa.
The realpath()
method eliminates any symbolic links encountered in the path, depending on whether the operating system supports them. The os.path.realpath()
method gets update in different Python versions.
- Update in Python version 3.6: The method takes a path-like object.
- Update in Python version 3.8: In Windows, symbolic links are solved.
- Update in Python version 3.10: The
strict
parameter, which takes a Boolean value, was added.
Syntax of Python os.path.realpath()
:
os.path.realpath(path, *, strict)
Parameters
path |
A specified path. This can be a string or a byte representing a path-like object on a file system. |
strict |
This is a Boolean type parameter. OSError will be raised if a symlink loop is found or a file is not found, which only happens when the strict value is true; otherwise, the method returns an appended value without exception if the strict parameter value is false. |
Return
This method returns a string data type, which shows the canonical path of the specified file by removing the symbolic link found in the path name.
Example Codes: Use the os.path.realpath()
Method to Obtain the Canonical Path
In this example, we will work on the os.path.realpath()
method to get the canonical path by removing any symbolic link in the provided path. The source code contains three different paths to evaluate the behavior of the os.path.realpath()
method.
Let us see the below example to demonstrate the concept further.
# importing os module
import os
# first Path with full path (root to path extension)
source_path = "/Users/lenovo/Downloads/myfile.txt"
# Retreiving the canonical path by eliminating any symbolic links
canonical_path = os.path.realpath(source_path)
print("Canonical path for the first source: ", canonical_path)
# second Path with file name only
source_path = "myfile.txt"
canonical_path = os.path.realpath(source_path)
print("Canonical path for the second source: ", canonical_path)
# third Path without naming the root directory
source_path = "/../myfolder/first.py"
canonical_path = os.path.realpath(source_path)
print("Canonical path for the third source: ", canonical_path)
Output:
Canonical path for the first source: C:\Users\lenovo\Downloads\myfile.txt
Canonical path for the second source: C:\Users\lenovo\.spyder-py3\myfile.txt
Canonical path for the third source: C:\myfolder\first.py
Example Codes: Difference Between the os.path.realpath()
and os.path.abspath()
Methods
As we have seen from the above example, the realpath()
method returns the canonical path of the specified parameter by removing the symbolic links. Similarly, another method, os.path.abspath()
, returns the absolute normalized path from the path name provided to the method as a parameter.
The abspath()
method also removes characters like .
and ..
from the provided path name.
This method os.path.abspath()
also takes a path as a string data type. To further understand the difference between the two methods, let us see the below example for clarification.
There are four different parameters for the abspath()
and realpath()
methods.
# os module for using paths methods realpath and abspath
import os
# same parameters for both the methods
print("Output for abspath() method")
print(os.path.abspath("./"))
print(os.path.abspath("./subdir/../data"))
print()
# this will show the difference between the two methods' output
print("Output for realpath() method")
print(os.path.realpath("./"))
print(os.path.realpath("./subdir/../"))
print()
# both abspath and realpath will not resolve or remove
print(os.path.abspath("~/myfile"))
print(os.path.realpath("~/myfile"))
# the returned path will be invalid
print(os.path.exists(os.path.abspath("~/myfile")))
print(os.path.exists(os.path.realpath("~/myfile")))
Output:
Output for abspath() method
C:\Users\lenovo\.spyder-py3
C:\Users\lenovo\.spyder-py3\data
Output for realpath() method
C:\Users\lenovo\.spyder-py3
C:\Users\lenovo\.spyder-py3
C:\Users\lenovo\.spyder-py3\~\myfile
C:\Users\lenovo\.spyder-py3\~\myfile
False
False
Example Codes: Difference Between the os.path.realpath()
and Path.resolve()
Methods
To interact with the OS paths, we work on methods linked to the os.path
module. We have seen the examples of the os.path.realpath()
method above.
The pathlib
module also provides methods similar to the os.path
module. To find a similar method in pathlib
, we have the Path.resolve()
method.
This method does not perform the same thing that os.path.realpath(
) provides. The resolve()
method differs when there is no path file.
This method also has a parameter, which is strict
. If this parameter value is true, this method returns the FileNotFoundError: No such file or directory
if no path exists for the specified path name, which is sent as a parameter to the path.resolve()
method.
It means that pathlib
does not give a way to check whether the given parameter exists or not (no validation is provided).
Let’s take an example to elaborate on the difference between the two methods further.
# comparison example for os.path vs. pathlib
# for os.path methods
import os
# for pathlib methods
from pathlib import Path
# the provided file does not exist in the system
print("The os.path.realpath() method returns: ", os.path.realpath("/abc.txt"))
print(
"The Path.resolve() method returns, when strict parameter False: ",
Path("/abc.txt").resolve(),
)
print(
"The Path.resolve() method returns, when strict parameter True: ",
Path("/abc.txt").resolve(True),
)
Output:
The os.path.realpath() method returns: C:\abc.txt
The Path.resolve() method returns, when strict parameter False: C:\abc.txt
FileNotFoundError: [WinError 2] The system cannot find the file specified: '\\abc.txt'
Hi, I'm Junaid. I am a freelance software developer and a content writer. For the last 3 years, I have been working and coding with Python. Additionally, I have a huge interest in developing native and hybrid mobile applications.
LinkedIn