如何在 Python 中改變工作目錄
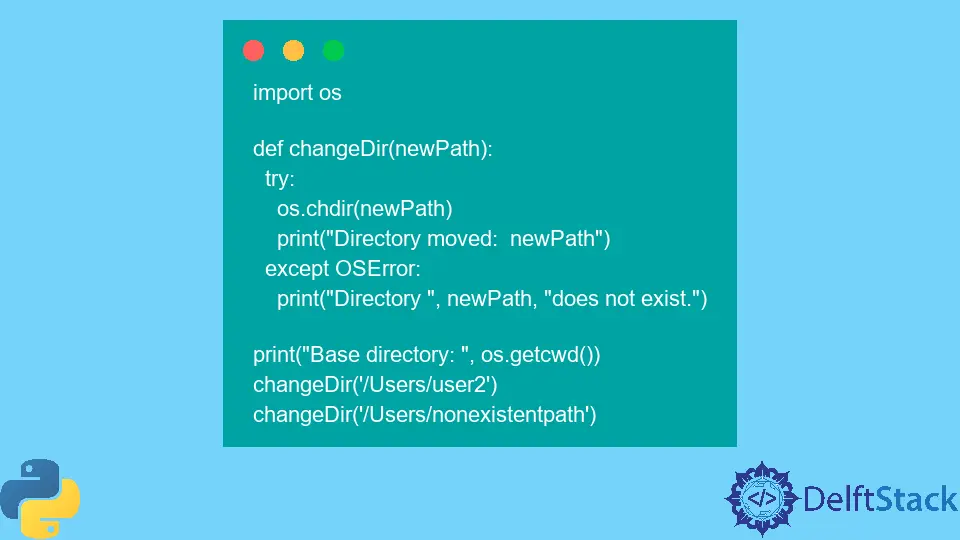
本文討論的是如何將 Python 中的當前工作目錄改變到其他位置。
使用 os
模組來改變 Python 中的目錄
假設出於某種原因,你想通過 Python 而不是命令控制檯來改變你的工作目錄。在這種情況下,模組 os
提供了直接通過 Python 實現各種作業系統實用程式的工具。
import os
比方說,你當前的工作目錄是/Users/user
,想改成 Users/user2
:首先,通過列印出 os.getcwd()
的結果來驗證當前的工作目錄。接下來就是呼叫 os.chdir("Users/user2")
塊來切換當前的工作目錄。
print(os.getcwd())
os.chdir("/Users/user2")
print(os.getcwd())
輸出:
/Users/user
/Users/user2
它驗證了你的程式已經成功地將工作目錄從 user
切換到 user2
。
異常檢查
路徑是使用者輸入的,所以很可能出現輸入錯誤。例如,使用者輸入了一個不存在的路徑;這個函式很可能會非常容易出現 FileNotFound 異常。鑑於此,我們應該實現異常檢查。
使用 if-else
檢查錯誤
簡單的方法是使用 if-else
檢查指定的路徑是否存在。
import os
def changeDir(newPath):
if os.path.exists(newPath):
os.chdir("Directory moved: ", newPath)
print(os.getcwd())
else:
print("Directory ", newPath, " not found.")
print("Base directory: ", os.getcwd())
changeDir("/Users/user2")
changeDir("/Users/nonexistentpath")
假設/Users/user2
是一個現有的檔案路徑,而/Users/nonexistentpath
不存在。
輸出:
Base directory: /Users/user
Directory moved: /Users/user2
Directory Users/nonexistentpath not found.
對 changeDir()
塊的第一個呼叫通過了 if
語句,因為路徑存在。另一方面,第二個經過 else
,並列印一個類似錯誤的資訊,因為路徑不存在。
使用 try...except
來檢查錯誤
如果 os.chdir()
命令沒有找到檔案路徑,它將丟擲一個 FileNotFoundError
通知。為此,我們需要在程式碼中使用 try...except
塊。
import os
def changeDir(newPath):
try:
os.chdir(newPath)
print("Directory moved: newPath")
except OSError:
print("Directory ", newPath, "does not exist.")
print("Base directory: ", os.getcwd())
changeDir("/Users/user2")
changeDir("/Users/nonexistentpath")
輸出:
Base directory: /Users/user
Directory moved: /Users/user2
Directory Users/nonexistentpath does not exist.
或多或少,兩者都會產生相同的輸出;然而,使用 try...except
異常處理比使用 if-else
語句要安全得多。因為使用者定義的條件可能無法覆蓋處理檔案路徑操作中可能發生的所有異常。
綜上所述,os
模組為檔案和目錄操作提供了廣泛的支援,除此之外,它還提供了其他實用程式。
我們可以直接使用 os.chdir()
塊來改變工作目錄,但為了避免異常情況的發生,還是用 if-else
或 try...except
塊來包住它比較安全。
如果你想探索更多關於檔案操作的問題,異常處理和更新檔名或內容是非常重要的。
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn