Python에서 작업 디렉토리 변경
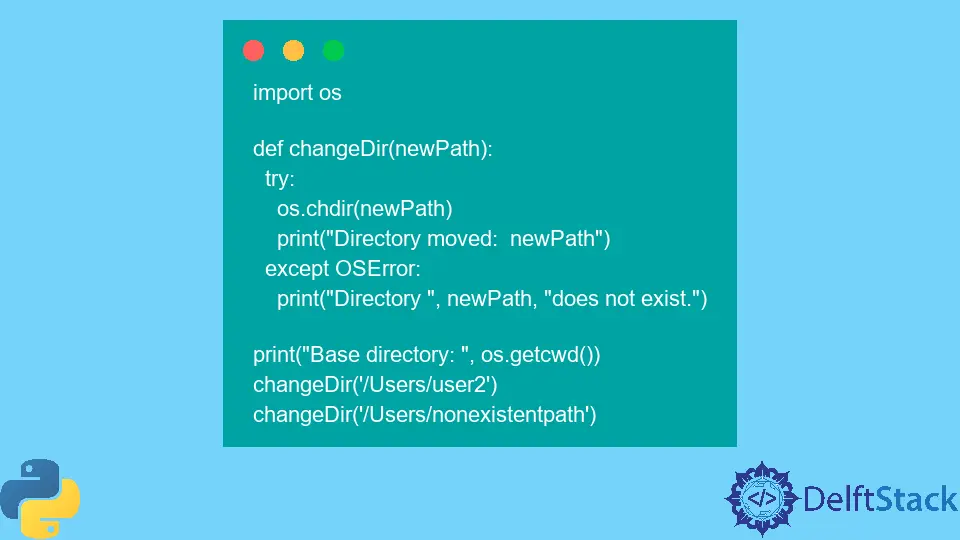
이 기사에서는 현재 작업 디렉토리를 Python에서 다른 위치로 변경하는 방법에 대해 설명합니다.
os
모듈을 사용하여 Python에서 디렉토리 변경
어떤 이유로 명령 콘솔 대신 Python을 통해 작업 디렉토리를 변경하려고한다고 가정합니다. 이 경우 os
모듈은 Python을 통해 직접 기타 운영 체제 유틸리티를위한 도구를 제공합니다.
import os
현재/Users/user
의 작업 디렉토리에 있고Users/user2
로 변경하고 싶다고 가정 해 보겠습니다. 먼저 os.getcwd()
의 결과를 출력하여 현재 작업 디렉토리를 확인하십시오. 다음은 os.chdir("Users/user2")
블록을 호출하여 현재 작업 디렉토리를 전환하는 것입니다.
print(os.getcwd())
os.chdir("/Users/user2")
print(os.getcwd())
출력:
/Users/user
/Users/user2
프로그램이 작업 디렉토리를user
에서user2
로 성공적으로 전환했는지 확인합니다.
예외 확인
경로는 사용자가 입력하므로 입력 오류가 발생할 가능성이 높습니다. 예를 들어, 사용자가 존재하지 않는 경로를 입력합니다. 이 함수는 FileNotFound 예외가 발생하기 쉽습니다. 이를 감안할 때 예외 검사를 구현해야합니다.
if-else
를 사용하여 오류 확인
이를 수행하는 간단한 방법은if-else
를 사용하여 지정된 경로가 존재하는지 확인하는 것입니다.
import os
def changeDir(newPath):
if os.path.exists(newPath):
os.chdir("Directory moved: ", newPath)
print(os.getcwd())
else:
print("Directory ", newPath, " not found.")
print("Base directory: ", os.getcwd())
changeDir("/Users/user2")
changeDir("/Users/nonexistentpath")
/Users/user2
가 기존 파일 경로이고/Users/nonexistentpath
가 존재하지 않는다고 가정합니다.
출력:
Base directory: /Users/user
Directory moved: /Users/user2
Directory Users/nonexistentpath not found.
경로가 존재하기 때문에changeDir()
블록에 대한 첫 번째 호출은if
문을 통과했습니다. 반면에 두 번째는 else
를 거쳐 경로가 존재하지 않기 때문에 오류와 같은 메시지를 출력합니다.
try...except
를 사용하여 오류 확인
os.chdir()
명령이 파일 경로를 찾지 못하면FileNotFoundError
알림이 발생합니다. 이것을 잡으려면try...except
블록을 코드로 감쌀 필요가 있습니다.
import os
def changeDir(newPath):
try:
os.chdir(newPath)
print("Directory moved: newPath")
except OSError:
print("Directory ", newPath, "does not exist.")
print("Base directory: ", os.getcwd())
changeDir("/Users/user2")
changeDir("/Users/nonexistentpath")
출력:
Base directory: /Users/user
Directory moved: /Users/user2
Directory Users/nonexistentpath does not exist.
둘 다 동일한 출력을 생성합니다. 그러나try...except
예외 처리를 사용하는 것이if-else
문을 사용하는 것보다 훨씬 안전합니다. 사용자 정의 조건이 파일 경로 조작을 처리 할 때 발생할 수있는 가능한 모든 예외를 포함하지 않을 수 있기 때문입니다.
요약하면os
모듈은 제공하는 다른 유틸리티 중에서 파일 및 디렉토리 조작에 대한 광범위한 지원을 제공합니다.
os.chdir()
블록을 사용하여 작업 디렉토리를 직접 변경할 수 있지만 예외가 발생하지 않도록if-else
또는try...except
블록을 감싸는 것이 더 안전합니다.
파일 조작에 대해 자세히 알아 보려면 예외 처리 및 파일 이름 또는 콘텐츠 업데이트가 매우 중요합니다.
Skilled in Python, Java, Spring Boot, AngularJS, and Agile Methodologies. Strong engineering professional with a passion for development and always seeking opportunities for personal and career growth. A Technical Writer writing about comprehensive how-to articles, environment set-ups, and technical walkthroughs. Specializes in writing Python, Java, Spring, and SQL articles.
LinkedIn