How to Get Home Directory in Python
-
Get Home Directory Using the
os.path.expanduser
Module in Python -
Get Home Directory Using the
pathlib
Library in Python
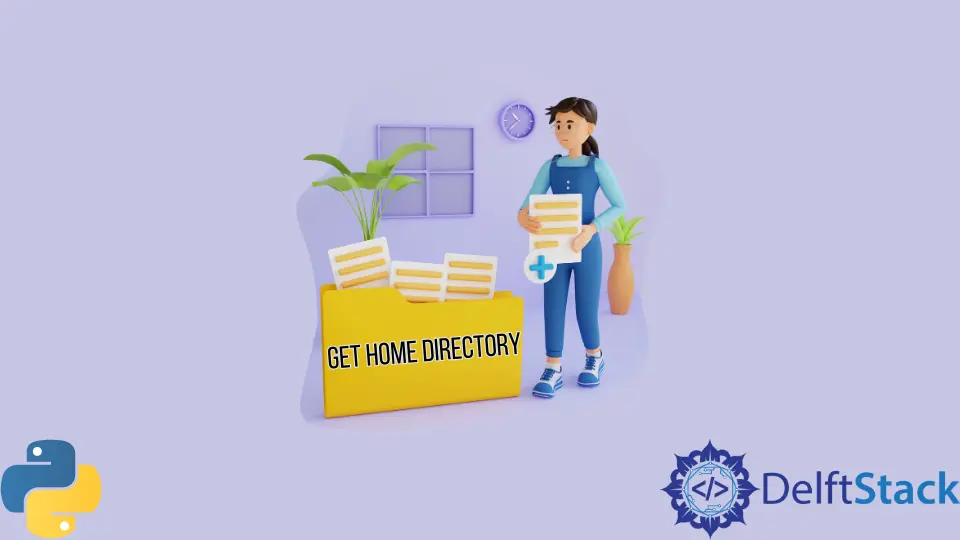
This tutorial will discuss getting the path of the home directory using the os.path.expanduser
or pathlib
library in Python.
Get Home Directory Using the os.path.expanduser
Module in Python
To get a user’s home directory, we can use the os.path.expanduser
in Python. We have to pass a string character ~
inside the os.path.expanduser()
function, which will return the home directory path of the current user logged in.
It uses the built-in password database or pwd
module to search for the path of the home directory, and the pwd
module provides the account details of a user like a login name and password. This module works on all platforms, like Windows and Linux.
For example, let’s get the home directory using the os.path.expanduser
module. See the code below.
from os.path import expanduser
home_directory = expanduser("~")
print(home_directory)
Output:
C:\Users\ammar
The above code is tested on Windows, and the output might change depending on the user and platform. If we want to find the path of the home directory of another user who is not currently logged in, we can add the user’s name after the ~
character in the above code.
We can also join two paths using the os.path.join()
function, like joining the home directory path with another path. We can pass the paths we want to join inside the os.path.join()
function.
For example, let’s join the above path with another path using the os.path.join()
function. See the code below.
from os.path import expanduser, join
home_directory = expanduser("~")
new_directory = join(home_directory, "newPathName")
print(home_directory)
print(new_directory)
Output:
C:\Users\ammar
C:\Users\ammar\newPathName
In the above code, we joined the home directory path with another path. We can also join multiple paths together, like 2 or more.
We cannot add a special character like backslash \
inside the join()
function because the backslash combined with some characters will not output the proper result. For example, if we change the above path to newPathName\tpath2
, the result will contain a tab space because \t
is used as a tab space in a string.
We recommend adding each path individually inside the join()
function. For example, if we want to join another path with the above path, we have to pass it as the third argument, and the join()
function will put it at the end of the new path, and the backslash will be added automatically.
See the example code below.
join(path1, path2, path3, ....)
Ensure to import the join()
and expanduser()
functions before using them inside the code. Check the os.path.expanduser
library for more details.
Get Home Directory Using the pathlib
Library in Python
We can also use the pathlib
library to get the path of the home directory using its home()
function. For example, let’s find the home directory using the pathlib
library.
See the code below.
from pathlib import Path
home_directory = str(Path.home())
print(home_directory)
Output:
C:\Users\ammar
In the above code, we used the str
function to convert the output of the home()
function into a string. This will work on all platforms, e.g., Windows and Linux; however, the output might change depending on the user and platform.
We can also join two or more paths using the PureWindowsPath().joinPath()
function of pathlib
library. The starting path will be passed inside the PureWindowsPath()
function and the new path or paths will be added in the joinPath()
function to get one combined path.
For example, let’s combine the above path with two paths using the PureWindowsPath().joinPath()
function. See the code below.
from pathlib import Path, PureWindowsPath
home_directory = Path.home()
new_directory = PureWindowsPath(home_directory).joinpath("newPathName", "path2")
print(home_directory)
print(new_directory)
Output:
C:\Users\ammar
C:\Users\ammar\newPathName\path2
In the above code, we added two paths, but we can add as many paths as we like, but we have to avoid the backslash inside the same string. This is because backslash combined with some special characters will create problems like \n
will add a new line, and \t
will add a tab space in the output.
We recommend adding each path individually inside the joinPath()
function.
Make sure to import the Path()
and PureWindowsPath()
function before using them inside the code. Check the pathlib
library for more details.