How to Get Directory From Path in Python
- Differences in File Path in Various OS
-
Use
os.path.basename
to Get Filename From the File Path in Python -
Use
os.path.splittext
to Get Filename From the File Path in Python -
Use
os.path.dirname
to Get the Directory Name From the File Path in Python -
Use the
pathlib
Module to Extract Directory Name in Python -
Use
os.path.abspath
to Get the Directory Name From the File Path in Python -
Use
Path.cwd()
to Get Directory Name From the File Path in Python -
Use
os.getcwd()
to Get Directory Name From the File Path in Python - Conclusion
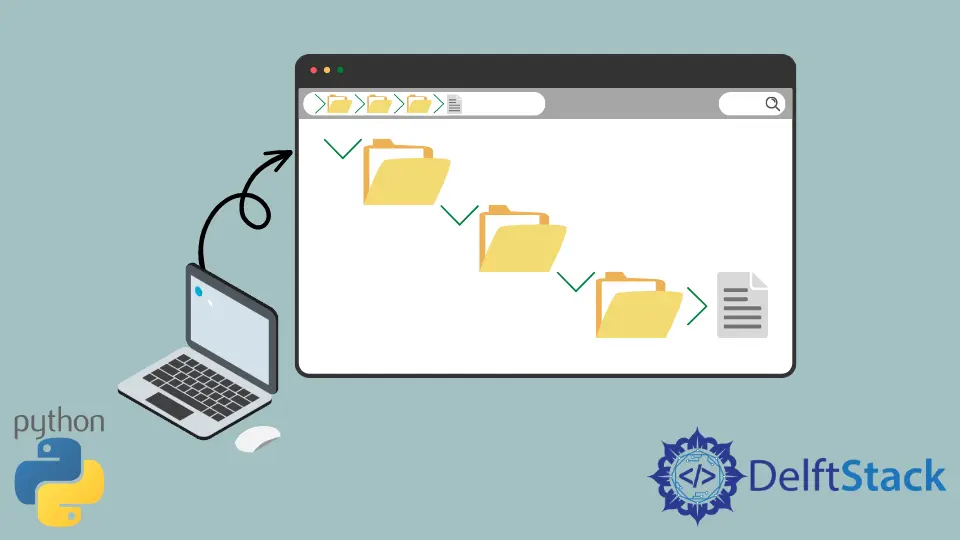
Whether it’s for data manipulation, file organization, or script execution, understanding how to work with file paths is essential. Python, with its rich standard library, offers multiple ways to interact with file paths and directories, making it a versatile choice for developers.
In this article, we delve into various Python methods such as os.getcwd()
, os.path.dirname
, and the pathlib
module, among others, to explore how they can be used to efficiently manage and retrieve directory information from file paths.
From beginners to seasoned programmers, this guide aims to provide a comprehensive understanding of directory manipulation in Python, ensuring that readers are well-equipped to handle file system paths in their projects.
Differences in File Path in Various OS
We use the forward-slash /
in the Linux directory structure (including MAC), while in Windows, we use the backward slash \
as the separator.
To check which separator your system uses, use the os.sep
or os.path.sep
. It will return the path separator used by your system.
Use os.path.basename
to Get Filename From the File Path in Python
The first and easiest way to extract part of the file path in Python is to use the os.path.basename()
function.
This function returns the filename from the file path along with its extension. Plus, it works for all the Python versions.
Example:
import os
# Example file path
file_path = "/home/user/documents/report.txt"
# First, get the directory of the file
directory_path = os.path.dirname(file_path)
# Now, use basename to get the last directory name
last_directory = os.path.basename(directory_path)
# Display the result
print("The last directory in the path is:", last_directory)
In this code, we start by defining a file path. Our goal is to extract the name of the last directory in this path.
We first use os.path.dirname
to get the complete directory path (/home/user/documents
) of the file.
Next, we apply os.path.basename
to this directory path. What os.path.basename
does here is that it treats the directory path as a normal path and extracts the last segment, which is documents
in this case.
Finally, we print the result using the print
function.
Output:
Use os.path.splittext
to Get Filename From the File Path in Python
While the function os.path.splitext
is typically known for splitting the extension from a filename, it can be creatively used in conjunction with other functions to extract directory information from a path.
The os.path.splitext
function is designed to separate the file extension from the rest of the filename. It splits the path string into a pair (root, ext)
such that root + ext == path
, and ext
is empty or begins with a period and contains at most one period.
Example:
import os
# Define the file path
fpath = "/home/user/example.txt"
# Split the extension and get the base path
base_path, _ = os.path.splitext(fpath)
# Extract the directory using os.path.dirname
directory = os.path.dirname(base_path)
print("The directory from the path is:", directory)
In this code, we start by importing the os
module, which is essential for handling various operating system-dependent functionalities, including file path operations.
Our first step involves defining a file path fpath
. We then use os.path.splitext(fpath)
to split this path into the base path and the extension.
However, since we aim to extract the directory, we are only interested in the base path, which is why we use _
(a conventional placeholder in Python for unwanted values) for the extension part.
The next step is crucial. We use os.path.dirname
on the base_path
to extract the directory path. This function is specifically designed to get the directory name from a given path.
Finally, we print the extracted directory to verify our result.
Output:
Use os.path.dirname
to Get the Directory Name From the File Path in Python
The function os.path.dirname()
is used to extract the directory name from the path. This function will return the directory name as the string on the Python console.
When you have a path, and you need to work with just the directory part (perhaps to check if the directory exists, to create a new file in the same directory, or to list all files in that directory), os.path.dirname
is the function you would use.
Example:
import os
# Define the file path
file_path = "/home/user/documents/report.txt"
# Extract the directory using os.path.dirname
directory = os.path.dirname(file_path)
# Display the extracted directory
print("Directory:", directory)
In this example, we begin by importing the os
module. This module is a standard utility in Python for interacting with the operating system, and it includes the os.path
suite for path manipulations.
Next, we define a variable file_path
containing a string that represents the full path to a file. The path includes both the directory structure (/home/user/documents
) and the file name (report.txt
).
The crucial part of this script is the line where we call os.path.dirname(file_path)
. This function analyzes the provided file_path
, identifies the directory component of the path, and returns it as a string.
In our case, it will extract /home/user/documents
as the directory path.
We then print the extracted directory to confirm our operation was successful.
Output:
Use the pathlib
Module to Extract Directory Name in Python
Another way to get the directory from the file path is to use the pathlib
module. This is specifically available in Python versions 3.4+.
When it comes to extracting a directory from a path, pathlib
provides a more straightforward and object-oriented approach. This is particularly useful in applications that involve file and directory management, such as automated scripting, data organization, and more.
Example:
from pathlib import Path
# Define the file path
file_path = "/home/user/documents/report.txt"
# Create a Path object
path = Path(file_path)
# Extract the directory
directory = path.parent
# Display the extracted directory
print("Directory:", directory)
In our code, we start by importing Path
from the pathlib
module. We then create a Path
object from a string that represents our file path.
This object-oriented approach makes the subsequent operations more intuitive.
The parent
attribute of the Path
object is used to obtain the directory part of the path. The beauty of pathlib
lies in its simplicity and the way it makes path manipulations more readable and straightforward.
Finally, we print the directory to verify the output. It’s worth noting that path.parent
returns a Path
object.
When printed, it displays the path as a string, but it retains its object properties for further manipulation if needed.
Output:
Use os.path.abspath
to Get the Directory Name From the File Path in Python
The OS module also offers the functionality to extract a directory from the file path.
This os.path.abspath
method takes two different arguments: backslash and dot character. The backslash character returns the root directory, and the dot returns the current directory.
The primary purpose of os.path.abspath
is to return a normalized absolutized version of the pathname. In simpler terms, it converts a relative or incomplete path to an absolute path.
Example:
import os
# Define a relative path
relative_path = "documents/report.txt"
# Get the absolute path
absolute_path = os.path.abspath(relative_path)
# Extracting the directory from the absolute path
directory = os.path.dirname(absolute_path)
# Display the directory
print("Directory:", directory)
In our code, we start by importing the os
module, which provides a portable way of using operating system-dependent functionality.
We define relative_path
, a string representing a relative path to a file. The path is relative because it doesn’t start from the root directory.
We then use os.path.abspath(relative_path)
to convert this relative path to an absolute path. The abspath
method normalizes the path and prepends the current working directory to form a complete path.
After obtaining the absolute path, we employ os.path.dirname
to extract the directory part of the absolute path. This method separates the directory path from the file name.
Finally, we print the directory to confirm the operation.
Output:
Use Path.cwd()
to Get Directory Name From the File Path in Python
The Path.cwd()
method, part of the modern pathlib
module, serves an important role in this domain. It provides a straightforward way to retrieve the current working directory of a Python script.
Knowing the working directory is essential for tasks like reading files, writing files, and generally managing file paths relative to the script’s execution location. This is particularly important for scripts that depend on relative paths.
Example:
from pathlib import Path
# Get the current working directory
current_directory = Path.cwd()
# Display the current working directory
print("Current Working Directory:", current_directory)
In our example, we start by importing the Path
class from the pathlib
module. The pathlib
module is a Python standard library module that provides an object-oriented interface for working with file system paths.
We then use Path.cwd()
to retrieve the current working directory. This method returns a Path
object representing the directory where the Python script is executed.
It’s important to note that this is different from the directory where the script is located if it’s being run from a different directory.
Finally, we print the current working directory. The Path
object is automatically converted to a string when printed, which represents the path of the current working directory.
Output:
Use os.getcwd()
to Get Directory Name From the File Path in Python
The os.getcwd()
method, a part of Python’s standard os
module, is a straightforward tool for this purpose. It’s widely used for obtaining the path of the directory in which the Python interpreter is currently executing.
os.getcwd()
stands for get current working directory
. This function is crucial for scenarios where the path of the executing script or the location of files relative to it matters.
Example:
import os
# Get the current working directory
current_directory = os.getcwd()
# Display the current working directory
print("Current Working Directory:", current_directory)
In the provided code, we begin by importing the os
module. This module provides a portable way of using operating system-dependent functionality like file and directory operations.
The function os.getcwd()
is then called to retrieve the current working directory. This function returns the absolute path as a string, which points to where the Python script is being executed.
It’s important to note that the current working directory is not necessarily the location of the Python script. It is the directory from which the script or the Python interpreter was invoked.
Finally, we print the obtained path. This is especially useful for debugging purposes or when your script’s behavior depends on the current working directory.
Output:
Conclusion
Throughout this article, we have explored different methods available in Python for managing and extracting directory information from file paths. Each method, whether it’s os.getcwd()
, os.path.dirname
, or the use of the pathlib
module, offers unique advantages and can be selected based on the specific requirements of a project.
Understanding these different techniques is crucial for Python developers, as file and directory manipulation forms a fundamental part of many programming tasks. Whether it’s for simple script execution or complex file management systems, the knowledge of these methods ensures that developers can handle paths and directories with ease and efficiency.
With this guide, readers should now feel confident in their ability to work with file paths in Python, enhancing their overall programming proficiency and capability to develop more robust and error-resistant applications.
Related Article - Python Directory
- How to Get Home Directory in Python
- How to List All Files in Directory and Subdirectories in Python
- How to Fix the No Such File in Directory Error in Python
- How to Execute a Command on Each File in a Folder in Python
- How to Count the Number of Files in a Directory in Python