How to Set File Path in Python
- Setting File Paths in Python
-
How to Set the File Path Using the (
\
) Character - How to Set the File Path Using the Raw String Literals
-
How to Set the File Path Using the
os.path.join()
Function -
How to Set the File Path Using the
pathlib.Path()
Function - How to Set Absolute Paths in Python
- How to Set Relative Paths in Python
- Conclusion
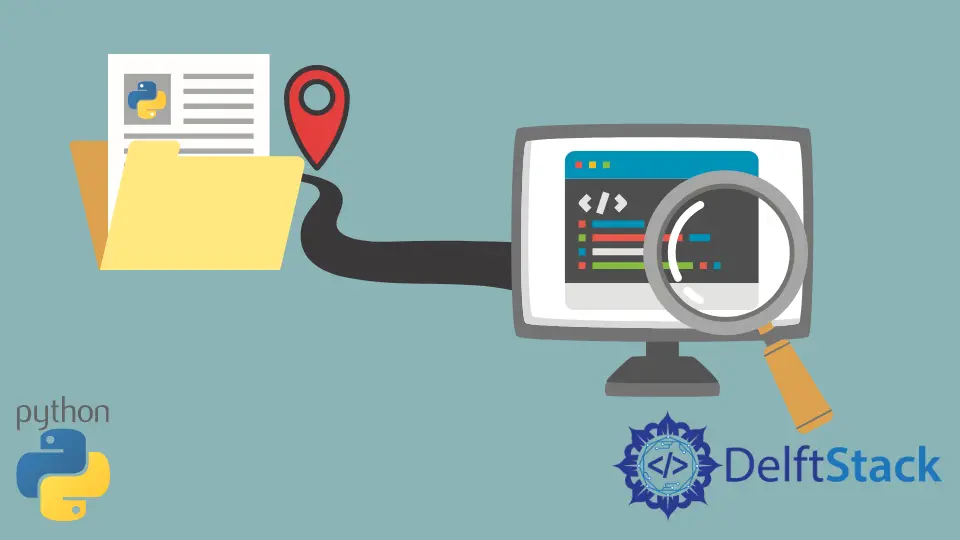
A file path is a string that specifies the unique location of a file or directory. Setting file paths in Python is a fundamental skill for working with files and directories.
Understanding how to specify paths correctly is crucial for reading, writing, or manipulating files. This tutorial will discuss how to set the path for a file in Python on Windows operating systems.
Setting File Paths in Python
Mostly, we are provided with the default path variable when we install Python. But sometimes, we have to set these variables manually, or if we want to set a different path, we have to do it manually.
To execute functions on files stored in our directories, it’s crucial to provide the program with the complete file path; a directory path often resembles "C:\Folder"
.
In this guide post, we explore best practices to overcome these nuances and ensure seamless file path handling in your Python scripts.
How to Set the File Path Using the (\
) Character
On Windows systems, the backslash (\
) is commonly used as the directory separator. We can use the (\\
) character in place of a single (\
) to provide the same path in Python.
Setting a file path in Python using the backslash (\
) character requires careful consideration due to its interpretation as an escape character in Python strings.
The syntax for this is shown below.
"C:\\Directory\\File"
Below is an example demonstrating how to set a file path using the (\
) character.
Code Input:
folder = "documents"
filename = "example.txt"
file_path = "C:\\Users\\" + folder + "\\" + filename
print("File Path using the \ Character:", file_path)
Output:
In this example, we build a file path using the backslash (\
) character. Variables folder and filename are set to "documents"
and "example.txt"
.
Concatenating them in the file_path
string, starting with "C:\\Users\\"
, creates the complete file path. The output displays this constructed path, ensuring proper formatting for a Windows file path.
How to Set the File Path Using the Raw String Literals
We can use raw string literals to provide paths for the files as a raw string will treat these backslashes as a literal character.
To make a raw string, note that we have to write the (r
) character before the quotes for the string.
The syntax for using raw string literals is shown below.
r"C:\Directory"
Below is an example demonstrating how to set a file path using raw string literals.
Code Input:
folder = "documents"
filename = "example.txt"
file_path = r"C:\Users\Username" + "\\" + folder + r"\\" + filename
print("File Path using using raw string literal:", file_path)
Output:
In this example, we build a file path object using a raw string literal, indicated by the 'r'
prefix. The folder
variable is set to "documents"
, and the filename
variable is set to "example.txt"
.
By concatenating these variables within the file_path
string and using a raw string literal "C:\Users\Username\\"
as the base, we create the complete file path. The output then displays this constructed path, ensuring that backslashes are treated as literal characters, maintaining their intended role as separators in a Windows file path.
This approach helps avoid unintended interpretations of backslashes as escape characters within the string.
How to Set the File Path Using the os.path.join()
Function
We can also use the os.path()
function of the setting up the path to same directory. The advantage of using the path()
function is that we don’t need to specify the complete path for the local file, we have to provide the directory name and the file name.
This method automatically adapts to the correct configuration for the working directory based on the operating system you are using on your device. We have to use the join()
function to combine the directory and the root filename.
Code Input:
import os
print(os.path.join("C:", os.sep, "Users"))
Output:
In this example, we use the os.path.join()
method to create a file path. The components "C:"
, the default OS separator (os.sep
), and "Users"
are joined together.
The method ensures proper formatting of folder paths specific to the operating system. The output, C:\Users
, showcases a correctly constructed user file path for Windows.
How to Set the File Path Using the pathlib.Path()
Function
In Python 3.4 and above, we can use the Path()
function from the pathlib
module to specify the file paths in Python. Its use is similar to the os.path()
function.
Set the File Path in Python 3.4 Using the pathlib.Path()
Function
Code Input:
from pathlib import Path
print(Path("C:", "/", "Users"))
Output:
In this example, using the Path
class, we construct a file path object with components "C:"
, "/"
, and "Users"
. The output, C:\Users
, demonstrates the successful creation of a platform-independent path, enhancing code clarity and portability.
How to Set Absolute Paths in Python
Setting an absolute file path in Python involves specifying the complete and unambiguous location of a file within the file system. Below are examples using both the os.path module and the pathlib module, two commonly used approaches.
How to Set Absolute Path Using the os.path
Module
Code Input:
import os
# Specify folder and filename
folder = "documents"
filename = "example.txt"
# Set absolute path manually
absolute_path = os.path.join("C:/Users", folder, filename)
print("Absolute Path using os.path:", absolute_path)
Output:
How to Set Absolute Path Using the pathlib
Module
Code Input:
from pathlib import Path
# Specify folder and filename
folder = "documents"
filename = "example.txt"
# Set absolute path manually
absolute_path = Path("C:/Users") / folder / filename
print("Absolute Path using pathlib:", absolute_path)
Output:
How to Set Relative Paths in Python
Setting relative file paths is often necessary when you want to reference files or directories based on their position relative to the location of your Python script or the current working directory. It’s an important concept, especially when you need your code to be portable across different systems or when organizing files and folders within a project structure.
How to Set Relative Path Using the os.path
Module
Code Input:
import os
# Specify the relative path to a file or directory
relative_path = "documents/example.txt"
# Get the current working directory
current_directory = os.getcwd()
# Combine the current directory and the relative path
file_path = os.path.join(current_directory, relative_path)
print("Relative Path in Python:", file_path)
Output:
How to Set Relative Path Using the pathlib
Module
Code Input:
from pathlib import Path
# Specify the relative path to a file or directory
relative_path = "documents/example.txt"
# Get the current working directory
current_directory = Path.cwd()
# Combine the current directory and the relative path
file_path = current_directory / relative_path
print("Relative Path in Python:", file_path)
Output:
Conclusion
In the post in conclusion, this guide delves into the crucial skill of setting file paths in Python, specifically focusing on the Windows operating system. Starting with the fundamentals of file paths and the potential challenges associated with backslashes, it proceeds to demonstrate practical examples.
The guide explores the use of double backslashes, raw string literals, and the os.path.join()
and pathlib.Path()
functions for constructing file paths. Each method is illustrated with examples and outputs, showcasing how to set paths for such file in various scenarios.
Additionally, the guide extends its coverage to absolute file paths, showcasing approaches using both os.path
and pathlib
modules.