Relative Path in Python
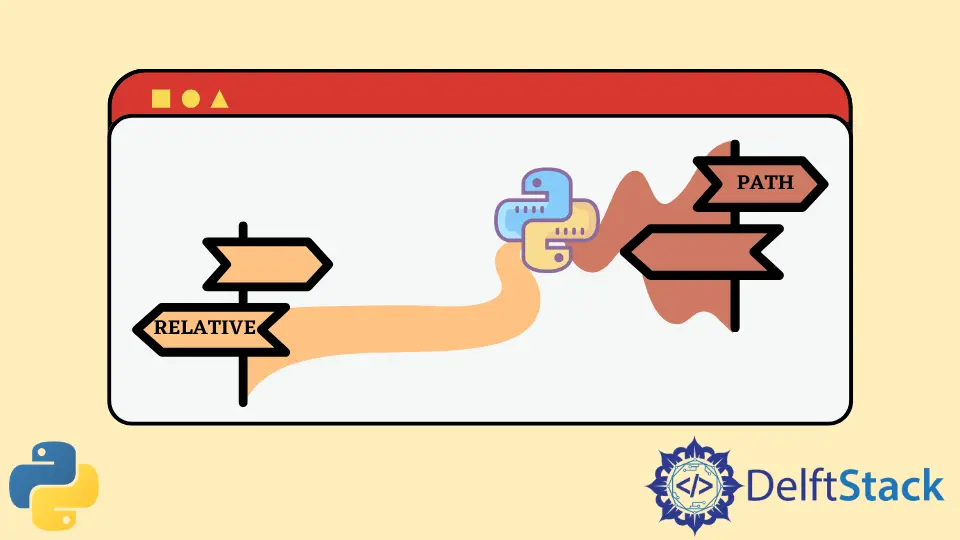
In this tutorial, we will discuss the relative path in Python.
File Path in Python
A file path specifies the location of a file in the computer. For example, C:\PythonProjects\Tutorials\Paths
is the path of a file paths.py
in my windows machine. Here, C:\
is the root directory, and PythonProjects
, Tutorials
and Paths
are subdirectories. paths.py
is a python script inside Paths
directory inside Tutorials
directory inside PythonProjects
directory inside the root directory C:\
. There are two types of file paths in Python, i.e., absolute and relative paths.
Difference Between Windows, Mac, and Linux
In Windows machines, \
is used as a separator between directory names whereas, in both Linux and Mac machines, /
is used as a separator. For example,
#file path in Windows
rootdirectory\\subdirectory\\subsubdirectory\\filename.extension
#file path in Mac and Linux
rootdirectory/subdirectory/subsubdirectory/filename.extension
In windows, there are two backslashes because each backslash needs to be escaped using another backslash. This can be managed by using os.path.join()
method. This method handles the separators according to the operating system. For example,
import os
pathname = os.path.join("root", "directory1", "directory2")
print(pathname)
Output:
#On Windows
root\directory1\directory2
#On Mac and Linux
root/directory1/directory2
This tutorial would contain \
as the separator to give the examples in Windows. We will use the following file hierarchy and set C:\PythonProjects\Tutorials\Paths
to be the current working directory.
Current Working Directory
The current working directory or cwd
in short is the directory from which the program is being executed. You can get the current working directory of the particular file by os.getcwd()
method.
import os
print(os.getcwd())
Output:
C:\PythonProjects\Tutorials\Paths
The current working directory can also be changed at runtime using the os.chdir()
method).
import os
print("Old cwd = " + os.getcwd())
os.chdir("C:\\PythonProjects\\Tutorials")
print("New cwd = " + os.getcwd())
Output:
Old cwd = C:\PythonProjects\Tutorials\Paths
New cwd = C:\PythonProjects\Tutorials
Absolute Path
An absolute path of a file is the complete path from the root directory to that particular file. For example, C:\PythonProjects\Tutorials\Paths\paths.py
is the absolute path of paths.py
file.
We can get the absolute path of the current file as shown below.
import os
absolutepath = os.path.abspath(__file__)
print(absolutepath)
Output:
C:\PythonProjects\Tutorials\Paths\paths.py
Navigate to Strings
Directory Using Absolute Path in Python
Navigating to a folder using the absolute path in Python is pretty easy. The only headache is that you have to know the exact names of all the directories from the root directory.
import os
print("Old cwd = " + os.getcwd())
os.chdir("C:\\PythonProjects\\Tutorials\\Strings")
print("New cwd = " + os.getcwd())
Relative Path
The absolute path is helpful, but it can become complex very soon. So, to minimize this complexity, a relative path is used. Relative path means the path of a certain file relative to the current working directory.
For example, if the current working directory is C:\PythonProjects\Tutorials
, the path.py
file’s relative path would be \Paths\paths.py
which is shorter and easier to use than the absolute path C:\PythonProjects\Tutorials\Paths\paths.py
.
The absolute path of a file remains the same everywhere, but the relative path changes depending upon the current working directory. This phenomenon is demonstrated in the following coding example.
Navigate to Strings
Directory Using Relative Path in Python
If we need to access files in the Strings
folder, we have to either use the complete absolute path C:\PythonProjects\Tutorials\Strings\string.py
or we can do as mentioned in the following code.
import os
import sys
absolutepath = os.path.abspath(__file__)
print(absolutepath)
fileDirectory = os.path.dirname(absolutepath)
print(fileDirectory)
# Path of parent directory
parentDirectory = os.path.dirname(fileDirectory)
print(parentDirectory)
# Navigate to Strings directory
newPath = os.path.join(parentDirectory, "Strings")
print(newPath)
Output:
C:\PythonProjects\Tutorials\Paths\paths.py
C:\PythonProjects\Tutorials\Paths
C:\PythonProjects\Tutorials
C:\PythonProjects\Tutorials\Strings
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn