How to Execute a Command on Each File in a Folder in Python
- Use the Command Prompt to Execute a Command on Every File That Is Present in a Folder in Python
-
Use the
os
Module to Execute a Command on Every File in a Folder in Python -
Use the
pathlib
Module to Execute a Command on Each File in a Folder in Python
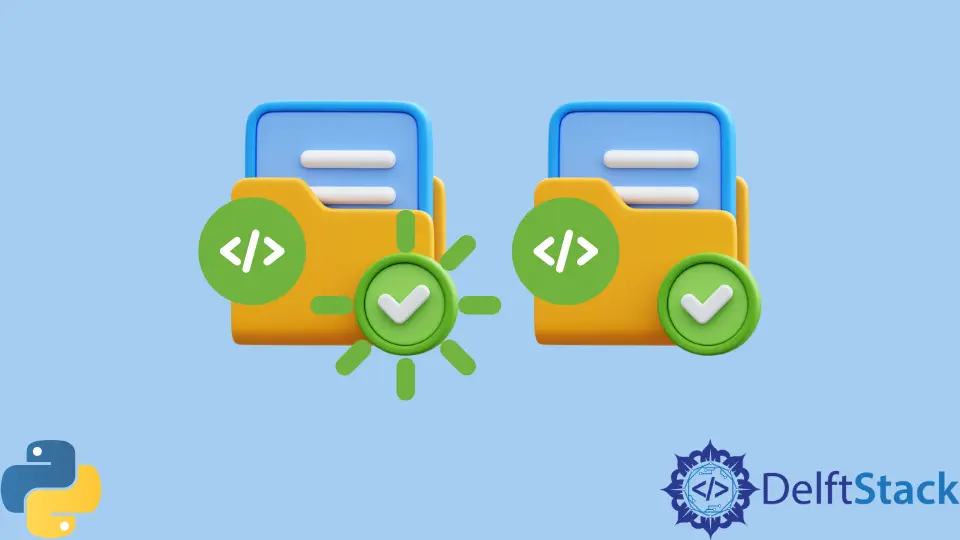
There are many interesting operations that we can perform on external files using Python, like extracting specific variables from a file, extracting the text written in a file, etc. One of the operations is executing a command in a Python file present in a specific folder using Python.
This tutorial will demonstrate how to execute a command on every file that is present in a folder using Python.
For this tutorial, we will assume two Python files with some Python statements to be executed and stored with the .py
extension present in a dictionary testFolder
.
These two python files are:
sample1.py
print("Hello World")
sample2.py
print(1 + 1)
Now, suppose these two files are stored in a folder known as testFolder
. Let us see some ways to print these files in Python.
Use the Command Prompt to Execute a Command on Every File That Is Present in a Folder in Python
To run every file that is present in a folder using the command line of the computer, we need to mention the path of the file. The command that is to be written in the command prompt is as follows:
python ../testFolder/sample1.py & python ../testFolder/sample2.py
Output:
Hello World
2
Use the os
Module to Execute a Command on Every File in a Folder in Python
The os
module in Python comes under Python’s standard utility modules. This module helps to interact with the operating system of the computer by providing various functions and methods. This module mainly comes into play when the user wants to interact with the computer’s file system.
In this method, a couple of methods are used from the os
module. The methods are os.listdir
, os.path.join
, and os.path.isfile
.
The os.listdir
method of the os
module helps get a complete list of all the files present in a directory. The argument of this method is the path of the directory. If no directory is mentioned in the argument, then the list of all the files present in the current directory will be returned by default.
The os.path.join
method of the os
module is used to concatenate one or more than one component of a specific path. This is done by a separator also known as directory separator /
.
The os.path.isfile
method of the os
module helps in checking if the file is present in the specified path or not. The argument of this method is always the path of the file.
import os
directory_name = "random"
for file_name in os.listdir(directory_name):
i = os.path.join(directory_name, file_name)
if os.path.isfile(i):
print(i)
Output:
Hello World
2
Use the pathlib
Module to Execute a Command on Each File in a Folder in Python
Python’s pathlib
module comes under the standard utility modules. This module helps in providing file paths in the form of classes and provides proper semantics to deal with the operating systems.
In this method, the path.glob()
function of the pathlib
module is used, which basically helps in accessing all the files in the given directory.
from pathlib import Path
directory_name = "random"
open_files = Path(directory_name).glob("*")
for file in open_files:
print(file)
Output:
Hello World
2
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn