How to Count the Number of Files in a Directory in Python
-
Use the
pathlib.Path.iterdir()
Function of thepathlib
Module to Count the Number of Files in a Directory in Python -
Use the
listdir()
Method of theos
Module to Count the Number of Files in a Directory in Python -
Use the
scandir()
Method of theos
Module to Count the Number of Files in a Directory in Python -
Use the
os.walk()
Method of theos
Module to Count the Number of Files in a Directory in Python -
Use the
glob
Method to Count the Number of Files in a Directory in Python - Conclusion
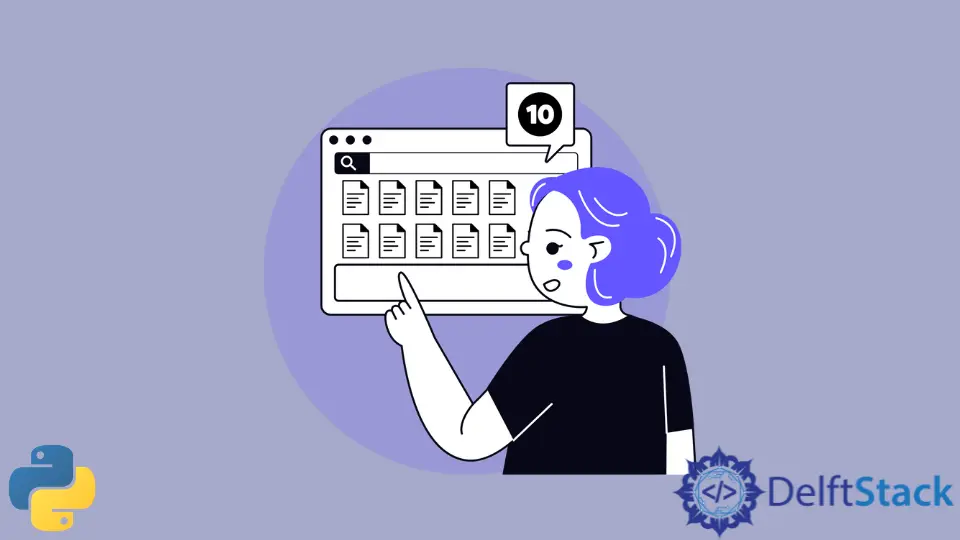
In Python, whenever someone has to work with a file and perform external operations on it, the working directory is always kept in mind. Without setting the correct working directory where the required file is present, the user cannot perform any operations on that file.
There might be situations when a user needs to know how many files are present in a particular directory.
This tutorial shows you methods on how to count the number of files in a directory in Python.
Use the pathlib.Path.iterdir()
Function of the pathlib
Module to Count the Number of Files in a Directory in Python
The pathlib
module comes under Python’s standard utility modules. This module helps the user by providing various classes and objects representing external file paths with a proper approach for interacting with operating systems.
The pathlib.Path.iterdir()
of the pathlib
module is used to get the path objects of the contents of a directory in Python; this is executed whenever the directory’s path is known.
from pathlib import Path
def count_files_in_directory(directory_path):
path = Path(directory_path)
file_count = sum(1 for entry in path.iterdir() if entry.is_file())
return file_count
directory_path = "Directory"
result = count_files_in_directory(directory_path)
print(f"Number of files in the directory: {result}")
We begin by importing the Path class from the pathlib
module.
Next, we define a function called count_files_in_directory
, designed to take a directory path as its input.
We proceed to create a Path
object using the given directory path.
Utilizing a generator expression with sum
, we efficiently count the number of files in the directory, ensuring that only files are considered by incorporating the condition entry.is_file()
.
Finally, we return the determined file count.
Use the listdir()
Method of the os
Module to Count the Number of Files in a Directory in Python
The os
module also comes under Python’s standard utility modules. It provides various methods or functions that are very useful whenever a user interacts with the operating system.
One of the methods of the os
module is the listdir()
method. This method returns a list of all the files present in a particular directory mentioned.
By default, the list of files and directories in the current working directory is returned if the user did not mention a directory.
import os
def count_files_in_directory(directory_path):
files = [
f
for f in os.listdir(directory_path)
if os.path.isfile(os.path.join(directory_path, f))
]
return len(files)
directory_path = "Directory"
result = count_files_in_directory(directory_path)
print(f"Number of files in the directory: {result}")
We start by importing the os
module. Subsequently, we define a function named count_files_in_directory
, which accepts a directory path as its parameter.
To obtain a list of entries in the directory, we use os.listdir(directory_path)
.
We then employ a list comprehension [f for f in os.listdir(directory_path)]
to filter out only the files by checking os.path.isfile(os.path.join(directory_path, f))
.
Finally, we return the count of files present in the directory.
Use the scandir()
Method of the os
Module to Count the Number of Files in a Directory in Python
The os.scandir
method is a valuable addition to Python’s file and directory manipulation toolkit. Introduced in Python 3.5, it enhances the functionality of os.listdir
by returning an iterator over the entries in a given directory.
Each entry returned by os.scandir
is an os.DirEntry
object, providing additional information about the entry, such as file type and attributes.
The primary advantage of os.scandir
is its performance. It can be notably faster than os.listdir
, especially when working with large directories, as it avoids unnecessary stat system calls.
import os
def count_files_in_directory(directory_path):
file_count = sum(1 for entry in os.scandir(directory_path) if entry.is_file())
return file_count
directory_path = "Directory"
result = count_files_in_directory(directory_path)
print(f"Number of files in the directory: {result}")
We initiate by importing the os
module. Following that, we define a function named count_files_in_directory
, designed to accept a directory path as its input.
Employing os.scandir(directory_path)
, we acquire an iterator over the entries in the directory.
Through the use of a generator expression with sum
, we efficiently count the number of files, ensuring that only entries identified as files via entry.is_file()
are considered.
Finally, we return the determined count of files in the directory.
Use the os.walk()
Method of the os
Module to Count the Number of Files in a Directory in Python
The os.walk
method is a robust tool for traversing directory trees in Python. It generates the file names in a directory tree by walking either top-down or bottom-up through the directory tree.
For each directory in the tree, it yields a tuple containing the directory path, a list of subdirectories, and a list of file names.
import os
def count_files_in_directory(directory_path):
file_count = 0
for _, _, files in os.walk(directory_path):
file_count += len(files)
return file_count
directory_path = "Directory"
result = count_files_in_directory(directory_path)
print(f"Number of files in the directory: {result}")
We begin by importing the os
module. Next, we define a function called count_files_in_directory
, designed to accept a directory path as its input.
Using a for
loop, we iterate over the tuples generated by os.walk(directory_path)
.
In each iteration, we accumulate the count of files by adding the length of the files list.
Finally, we return the determined count of files in the directory.
Use the glob
Method to Count the Number of Files in a Directory in Python
The glob
module is a versatile tool in Python for pattern matching and file handling. The glob
method specifically allows for file path expansion using wildcards, making it a powerful choice for tasks such as file enumeration.
It is particularly useful when you want to filter files based on a specific pattern or extension within a directory.
import glob
import os
def count_files_in_directory(directory_path):
files = glob.glob(f"{directory_path}/*")
file_count = sum(1 for file in files if not os.path.isdir(file))
return file_count
directory_path = "Directory"
result = count_files_in_directory(directory_path)
print(f"Number of files in the directory: {result}")
We start by importing the glob
module.
Subsequently, we define a function called count_files_in_directory
, which accepts a directory path as its parameter.
To obtain a list of file paths in the specified directory, we utilize glob.glob(f"{directory_path}/*")
.
Employing a list comprehension, we filter out directories and exclusively count the files.
Finally, we return the determined count of files in the directory.
Conclusion
This comprehensive tutorial explores various methods for counting the number of files in a directory using Python. The tutorial first introduces the pathlib
module, demonstrating the use of the Path.iterdir()
function and recognizing the significance of setting the correct working directory for file operations.
Subsequently, it explores alternatives using the os
module, showcasing the listdir()
and scandir()
methods, each offering unique advantages in terms of simplicity and performance. Additionally, the tutorial delves into the robust os.walk()
method, providing a versatile solution for traversing directory trees.
Lastly, the tutorial highlights the versatility of the glob
module, which is especially useful for pattern-based file enumeration. With practical code examples and explanations, this tutorial equips Python developers with diverse approaches to efficiently count files in a directory, catering to different preferences and requirements.
Lakshay Kapoor is a final year B.Tech Computer Science student at Amity University Noida. He is familiar with programming languages and their real-world applications (Python/R/C++). Deeply interested in the area of Data Sciences and Machine Learning.
LinkedIn