How to Detect Colors in Python OpenCV
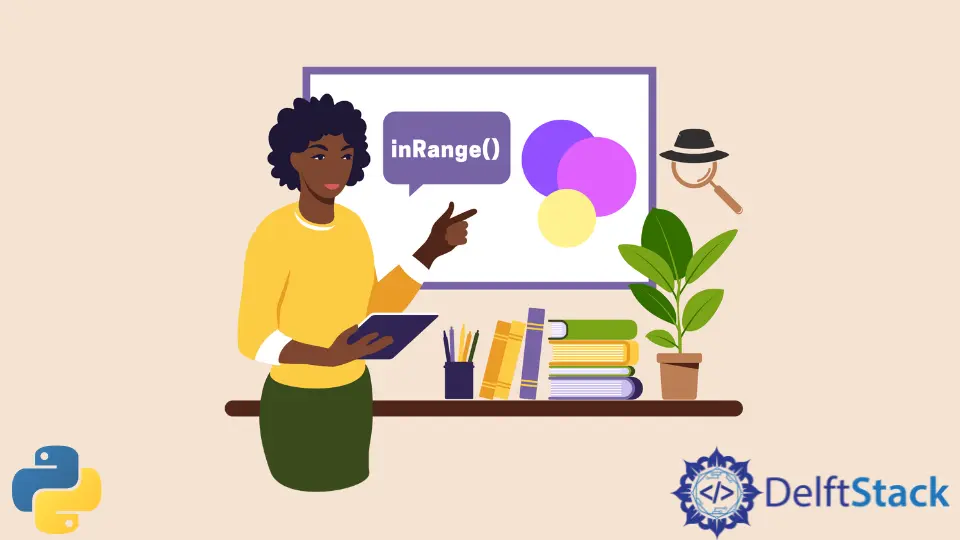
This tutorial will discuss detecting colors in images using the inRange()
function of OpenCV in Python.
Use the inRange()
Function of OpenCV to Detect Colors on Images in Python
We can detect and extract colors present in an image using the inRange()
function of OpenCV. Sometimes, we want to remove or extract color from the image for some reason.
We can use the inRange()
function of OpenCV to create a mask of color, or in other words, we can detect a color using the range of that color. The colors are stored in an RGB triplet value format inside a color image.
To create its mask, we have to use the RGB triplet value of that color’s light and dark version. For example, in a BRG image, if we want to extract the red color, we can use (0,0,50) for light red and (255,50,50) for the dark red color to create a mask of red color.
We can pass the image and the lower and upper BRG values to create a mask.
For example, let’s read an image containing some circles of different colors and create a mask of red color and a mask of green color. See the code below.
import cv2
img = cv2.imread("download.png")
# mask of red color
mask1 = cv2.inRange(img, (0, 0, 50), (50, 50, 255))
# mask of blue color
mask2 = cv2.inRange(img, (50, 0, 0), (255, 50, 50))
cv2.imshow("Original Image", img)
cv2.imshow("mask red color", mask1)
cv2.imshow("mask blue color", mask2)
cv2.waitKey(0)
Output:
We can compare the two masks with the original image to know if they contain the right color or not. We can see that mask one is on top of red color and mask two is on top of blue color.
The inRange()
function creates a new binary image similar to the original image. As you see, there are only two colors in the mask image white and black.
The inRange()
function sets the value of the color to 1 or white if color is present in the given color range and 0 if the color is not present in the specified color range. The above image was in the BRG color scale.
That’s why we used BRG values inside the inRange()
function, but we can also convert images to other color scales like HSV, and in this case, we will use the HSV scale value to create a mask. Now let’s extract the red and blue colors from the image.
To extract the two colors, we have to combine the two masks to create a single mask using the bitwise_or()
function of OpenCV. After that, we can use the bitwise_and()
function of OpenCV to extract the colors from the image using the image as the first and second argument and mask as the third argument.
See the code below.
import cv2
img = cv2.imread("download.png")
# mask of red color
mask1 = cv2.inRange(img, (0, 0, 50), (50, 50, 255))
# mask of blue color
mask2 = cv2.inRange(img, (50, 0, 0), (255, 50, 50))
# final mask
mask = cv2.bitwise_or(mask1, mask2)
target = cv2.bitwise_and(img, img, mask=mask)
cv2.imshow("Original Image", img)
cv2.imshow("mask red color", mask1)
cv2.imshow("mask blue color", mask2)
cv2.imshow("mask of both colors", mask)
cv2.imshow("target colors extracted", target)
cv2.waitKey(0)
Output:
The red and blue color is successfully extracted from the given image, as seen in the last image. To convert one color space to another, we can use the cvtColor()
function of OpenCV.
If we have an image with HSV color space, we must use the HSV values to create a mask. In HSV, color has three values Hue, Saturation, and Value which define the color.
Related Article - Python OpenCV
- How to Fix No Module Named CV2 in Mac in Python
- Image Masking in OpenCV
- How to Utilize Bitwise_AND on an Image Using OpenCV
- OpenCV Package Configuration
- OpenCV ArUco Markers
- How to SIFT Using OpenCV in Python