OpenCV sobel() Function
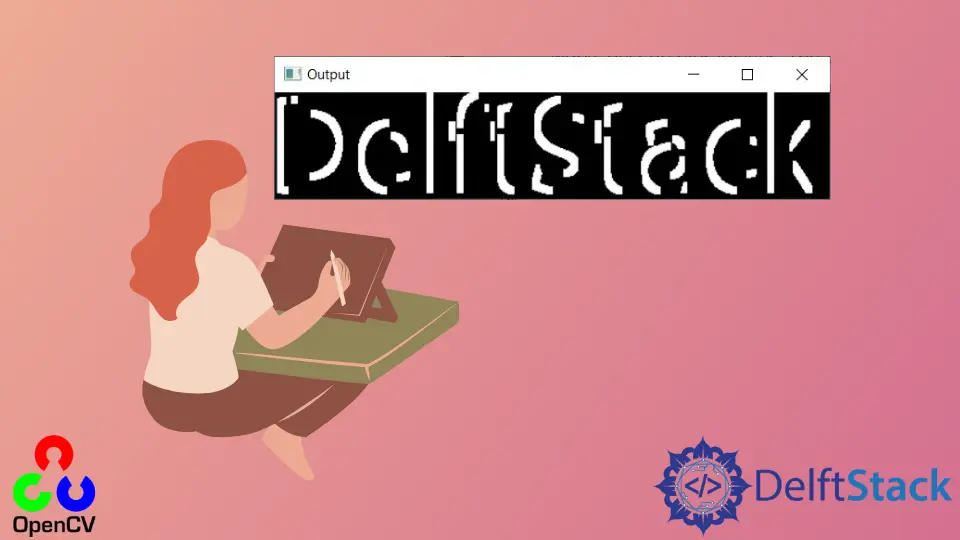
Using the OpenCV library, we can process and apply various techniques to images. Such processes form an integral part of complicated Computer Vision tasks, and one such task is edge detection in images.
Edges are the boundaries or outlines of objects in an image and are associated with very high changes in the density of pixels. By comparing the density of neighboring pixels, we can detect edges.
Various algorithms are available for edge detection and are applied in the OpenCV library. One such technique is the Sobel Edge Detection algorithm.
This tutorial will demonstrate the Sobel algorithm using OpenCV in Python.
Edge Detection Using the Sobel()
Function Using OpenCV in Python
The Sobel Edge Detection algorithm uses the image gradient to predict and find the edges in an image. We compare the pixel density to detect edges using this algorithm.
We calculate the first derivative of the function to find the peak points. These are then compared with the threshold value.
In this technique, the Sobel operator computes the gradient of the function. It combines the Gaussian smoothening and differentiation.
In general, we use kernels to smoothen or blur an image, but in this case, we will use them to calculate the gradients. The derivates are calculated along both the x and y-axis.
The gradient at a given point is calculated using both these values. This gradient value is less noise-prone and is then used for edge detection.
The OpenCV library implements this using the Sobel()
function. We need to specify the function with several parameters along with the image.
We need to mention the depth of the final image, specified with the ddepth
parameter. With the value of -1, the output image will have the same depth as the input image.
The order of derivatives to be used is specified using the dx
and dy
parameters. The size of the extended Sobel kernel is mentioned using the ksize
parameter.
The scale
and delta
parameters are optional. Let us now see an example.
import cv2
i = cv2.imread("deftstack.png")
img = cv2.GaussianBlur(i, (3, 3), sigmaX=0, sigmaY=0)
edge_sobel = cv2.Sobel(src=img, ddepth=cv2.CV_64F, dx=1, dy=1, ksize=5)
cv2.imshow("Output", edge_sobel)
cv2.waitKey(0)
cv2.destroyAllWindows()
Output:
In the above example, we used the Sobel()
function. We calculated the derivative of the first order along the x and y-axis and obtained the gradient, which is then used to find the outlines.
The final image is 64 bits, as specified in the ddepth
parameter. The kernel used is of size 5x5.
Note the GaussianBlur()
function; it is used to blur a given image, and a Sobel operator works best on blur images. The waitKey()
and destroyAllWindows()
functions prevent the output window from closing and wait for the user to press some key to exit.
As discussed, we calculated the derivative along the x and y-axis in the above example. We can also calculate the derivate along with either axis by putting the derivative of the other as 0.
For example,
import cv2
i = cv2.imread("deftstack.png")
img = cv2.GaussianBlur(i, (3, 3), sigmaX=0, sigmaY=0)
edge_sobel = cv2.Sobel(src=img, ddepth=cv2.CV_64F, dx=1, dy=0, ksize=5)
cv2.imshow("Output", edge_sobel)
cv2.waitKey(0)
cv2.destroyAllWindows()
Output:
In the above code, we calculate the derivative only along the horizontal direction and put the value of the dy
parameter as 0. To calculate the derivative vertically, we put the dx
parameter as 0.
Note that in our examples, we used the kernel size as 5x5. We can also use the 3x3 size, but the result contains many inaccuracies in this case and is not recommended.
The Scharr filter is another operator similar to Sobel and can be used in 3x3 cases.
Conclusion
This tutorial has demonstrated using the Sobel()
function from the OpenCV library. We first started by understanding about Sobel Algorithm for Edge Detection.
We understood the basics of image gradients and how the Sobel operator calculates this efficiently. Examples of different situations are demonstrated in the article.
The disadvantage of using a 3x3 kernel is also discussed, and its alternative, the Scharr filter, can be used to provide better results in this case.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python OpenCV
- How to Fix No Module Named CV2 in Mac in Python
- Image Masking in OpenCV
- How to Utilize Bitwise_AND on an Image Using OpenCV
- OpenCV Package Configuration
- OpenCV ArUco Markers
- How to SIFT Using OpenCV in Python