How to Utilize Bitwise_AND on an Image Using OpenCV
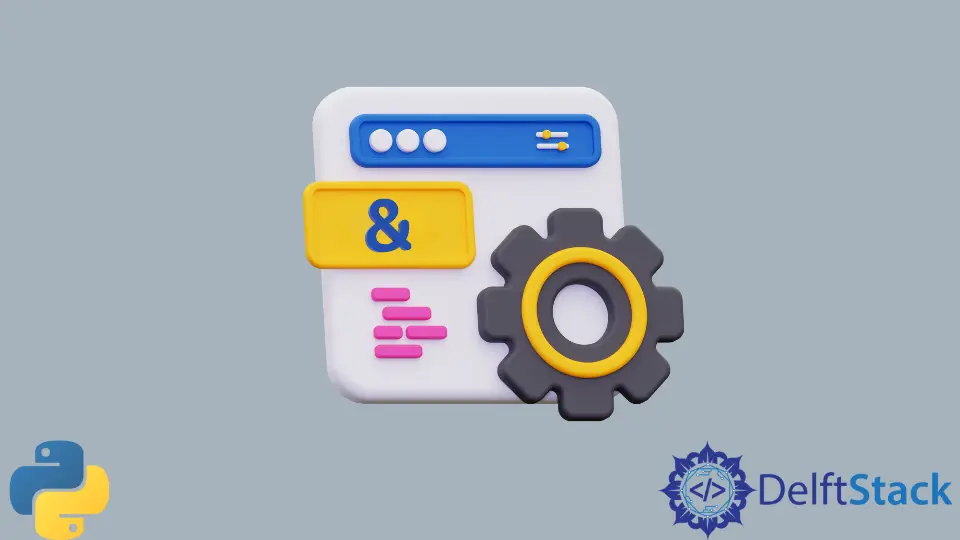
The bitwise operators are typically used to perform bitwise operations on patterns of bits or binary numbers that use individual bit manipulation. OpenCV uses this same concept to manipulate and extract information from the images.
In this article, we will specifically see how to utilize Bitwise AND
using the Python OpenCV library.
Bitwise Operators in OpenCV
The basic bitwise operators used in OpenCV are as follows.
Bitwise AND
Bitwise OR
Bitwise NOT
Bitwise XOR
Bitwise operators are used in OpenCV so that we can extract or filter out the part of an image, portraying the image and operating with non-rectangular ROIs (Region of Interest).
Use Bitwise AND
Operator on Images in OpenCV
In OpenCV, the Bitwise AND
operator is used to combine two different images into one, or it can combine some part of an image into another. It generally computes the bitwise logical combination per element of two arrays/scalar/images.
OpenCV has an inbuilt method to perform bitwise operations. So for Bitwise AND
operator in OpenCV, we use cv.bitwise_and()
.
Syntax of Python OpenCV bitwise_and()
cv.bitwise_and(src1, src2[, dst[, mask]]) -> dst
Parameters
src1 |
first input array / image. |
src2 |
second input array / image. |
dst |
output image with the same size and type as the input array. |
mask |
optional operation mask, 8-bit single channel array that specifies elements of the output array to be changed. |
Let’s see how to use the Bitwise AND
operator on an image with an example shown below.
First, we have to import OpenCV as cv
, and then using the imread()
method of OpenCV, we have to read two images using imread()
. This method will read the provided image and return the image data in array format.
Now, we have to store this data that is returned by the imread()
inside the variables named img1
and img2
. Before applying the Bitwise AND
, you must ensure that the two images you are using have the same shape, meaning the same width, height, and number of channels.
If you are unsure about the image shape, you can use the shape()
method of OpenCV as follows.
Code Snippet:
# import opencv
import cv2 as cv
# read the images
img1 = cv.imread("img1.jpg")
img2 = cv.imread("img2.jpg")
print("Shape of img_1 : ", img1.shape)
print("Shape of img_2 : ", img2.shape)
dimension = (img2.shape[1], img2.shape[0])
img1 = cv.resize(img1, dimension, interpolation=cv.INTER_AREA)
print("Shape of img_1 : ", img1.shape)
print("Shape of img_2 : ", img2.shape)
Output:
Shape of img_1 : (4000, 6000, 3)
Shape of img_2 : (3133, 4700, 3)
Shape of img_1 : (3133, 4700, 3)
Shape of img_2 : (3133, 4700, 3)
In most cases, the size of the two images will not be the same, so you might have to resize the image sizes using the resize()
method, as shown in the above code. In this case, you can either change the size of any one image or the sizes of both images.
For this example, we will change the size of img1
using the resize()
method as that of the size of img2
as shown above.
Code Snippet:
bitwise_AND = cv.bitwise_and(img1, img2)
cv.imshow("Image-1", img1)
cv.imshow("Image-2", img2)
cv.imshow("Bitwise-AND", bitwise_AND)
cv.waitKey(0)
Now that we have the two images of the same size, we can use the cv.bitwise_and()
to perform a Bitwise AND
operation on the given two images and store the output in the variable named bitwise-AND
. To display all the input, output, and resultant images, we will use the imshow()
function.
We are displaying all the images to clarify how the input images are displayed for the output image. This is what the output of the above code will look like.
We also use the waitKey()
method and pass the value 0
to it because it allows users to display a window for a short period or until any key is pressed. So, if 0
is passed in the argument, it waits until any key is pressed.
This is how we perform Bitwise AND
on two images of the same sizes.
Sahil is a full-stack developer who loves to build software. He likes to share his knowledge by writing technical articles and helping clients by working with them as freelance software engineer and technical writer on Upwork.
LinkedIn