How to Detect Edge in Python OpenCV
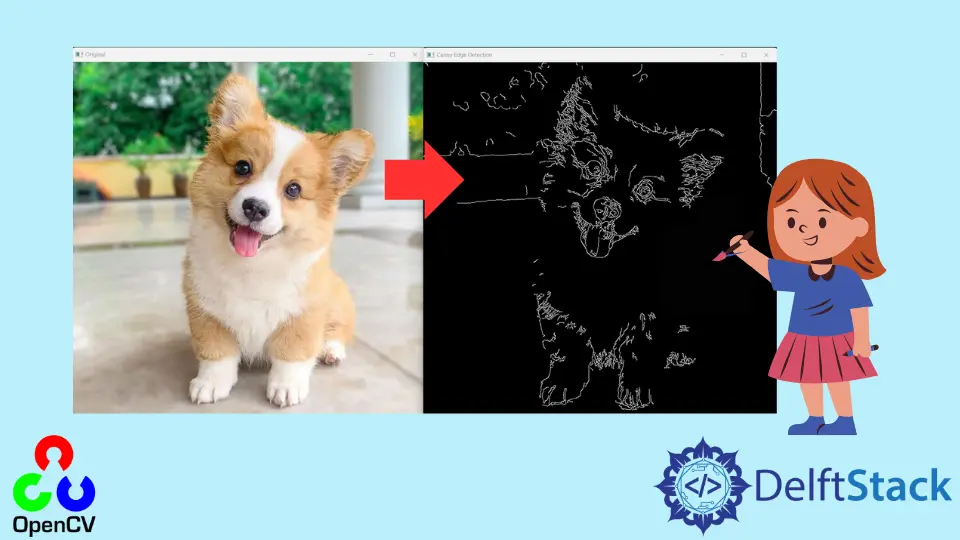
Edge detection is widely used in image processing for background removal, shape detection, and image structure analysis. In image processing, the edges present in an image are characterized as the sudden change in pixel intensity level.
For example, if there is a picture of a cat with a black background, on the edges of this picture, there will be a sudden change in color or pixel value like from black color to white color.
There are two methods in OpenCV that we can use to detect the edges present in an image, one is a Canny edge detector, and the other is a Sobel edge detector.
This tutorial will discuss detecting edges in an image using the canny or Sobel edge detector in OpenCV.
OpenCV Canny Edge Detection
We can use the Canny()
function of OpenCV for canny edge detection. We have to smooth the image using the GaussianBlur()
function for a better result.
Some extra edges can be detected during edge detection that is not part of the actual edges that we want to detect due to a sudden change in pixel intensity. That’s why we need to remove the noise present in the given image.
For example, let’s read an image using imread()
function and convert it to grayscale using the cvtColor()
function.
After that, we will smooth the image and then pass it inside the Canny()
function along with the upper and lower thresholds to detect the edges present in the image.
See the code below.
import cv2
img_src = cv2.imread("cat.jpg")
cv2.imshow("Original", img_src)
gray_img = cv2.cvtColor(img_src, cv2.COLOR_BGR2GRAY)
blur_img = cv2.GaussianBlur(gray_img, (3, 3), 0)
img_edges = cv2.Canny(image=blur_img, threshold1=50, threshold2=155)
cv2.imshow("Canny Edge Detection", img_edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
Output:
The canny edge detector is multistage, and it goes through multiple steps to find the edges present in an image. In the first step, the algorithm reduces the noise present in the given image.
In the second step, the algorithm finds the first derivative of the given image in the x and y direction, and then using this derivative, it finds the magnitude and angle of the edge gradient.
In the third step, the algorithm removes the unwanted edges by comparing the local maximum pixels with its neighborhood.
In the fourth step, the algorithm filters the edges using the two intensity levels defined by the user. If the value of an edge is between the range of the defined intensity, it will be considered a valid edge.
In the above code, the first argument image
of the Canny()
function is the given image, which should be 8-bit. The second argument threshold1
and the third argument threshold2
set the threshold range.
The Canny()
function also has two optional arguments. The first optional argument, apertureSize
, is used to set the aperture size for the Sobel operator, and by default, its value is set to 3.
The second optional argument, L2gradient
, sets the gradient type. By default, it is set to false for normal gradient, and if we set it to true the function will use L2 gradient.
If we change the threshold range in the Canny()
function, the output will change because the range filters the edges.
OpenCV Sobel Edge Detection
The canny edge detector is multistage, as discussed above. It removes the noise, finds the edges, and filters them using the threshold range.
If we don’t want to remove the noise or filter the edges, we can use the Sobel()
function of OpenCV instead of Canny()
. The Sobel()
function finds the derivative of an image in x, y, or both directions, and then it convolves the image with a kernel to get the Sobel edge image.
The Sobel()
function also finds the edges using the sudden change in pixel intensity. For example, let’s find the edges of the above cat image using the Sobel()
function.
See the code below.
import cv2
img_src = cv2.imread("cat.jpg")
cv2.imshow("Original", img_src)
gray_img = cv2.cvtColor(img_src, cv2.COLOR_BGR2GRAY)
blur_img = cv2.GaussianBlur(gray_img, (3, 3), 0)
sobel_x = cv2.Sobel(src=blur_img, ddepth=cv2.CV_64F, dx=1, dy=1, ksize=3)
cv2.imshow("Sobel Edge Detection", sobel_x)
cv2.waitKey(0)
cv2.destroyAllWindows()
Output:
As we can see, there are a lot of edges present in the above image, and it even contains the unwanted edges because the edges are not filtered, and we also did not remove the noise present in the image. The first argument src
of the Sobel()
function is the source image.
The second argument, ddepth
, is used to set the depth of the output image. The third argument, dx
, is used to set the order of the x derivative, and the fourth argument, dy
, is used to set the order of the y derivative.
The fourth argument, ksize
, is used to set the kernel size, and its value should be 1, 3, 5, or 7. The fifth argument, scale
, is used to set the scale factor for the derivative, and by default, no scale is used.
The sixth argument, delta
, is also optional and is used to set the delta value added to the output. The seventh argument, borderType
, is also optional, and it is used to set the method for pixel extrapolation, and by default, the border type is set to default border.
Check this link for more details about the border types.