OpenCV Canny in Python
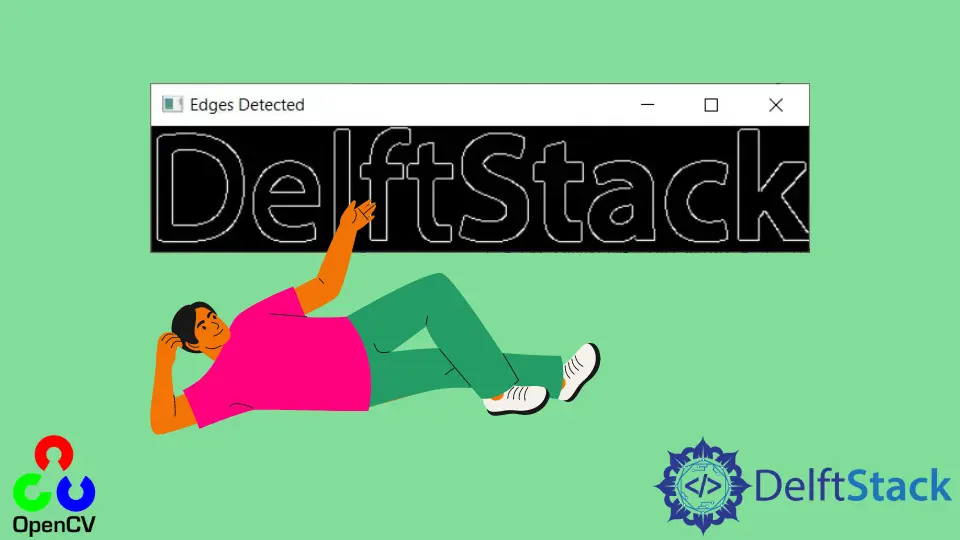
We use the opencv
library to work with images and graphics for computer vision in Artificial Intelligence, Machine Learning, and more techniques. We can use the functionalities from this library to read and process images efficiently.
This tutorial will demonstrate the cv2.canny()
function in Python.
Use the cv2.canny()
Function in Python
The cv2.canny()
function implements the Canny Edge Detection algorithm developed by John F. Canny. We can use this function to detect edges from a given image.
The algorithm in itself has multiple stages.
-
The first stage involves noise reduction, and for this, the algorithm uses a 5x5 Gaussian Filter.
-
The next stage involves finding the intensity gradient for the given image. In this stage, the image is smoothened and then passed to the Sobel kernel, where it is filtered along the x and y-axis, and its gradient is found for each axis.
-
In the third stage, every pixel is checked with the local maxima in the direction of the gradient to remove the undesirable pixels that do not form an edge.
-
The final stage is the one where the edges are classified. Two threshold values,
minVal
andmaxVal
, are taken.Edges with gradient values greater than
maxVal
are the edges, and those belowminVal
are not. The rest of these threshold values are categorized based on their connectivity.
All the above stages are implemented by the cv2.canny()
function. It is necessary to know about these stages while determining the parameters for this function.
In the following example, we will detect the edges from an image.
import cv2
img = cv2.imread("deftstack.png")
e = cv2.Canny(img, threshold1=50, threshold2=100)
cv2.imshow("Edges Detected", e)
cv2.waitKey(0)
cv2.destroyAllWindows()
In the above example, we read the image using the cv2.imread()
function. The edges are detected for this image using the cv2.canny()
function.
Notice the two parameters in the function threshold1
and threshold2
. These two parameters are the value of the minVal
and maxVal
threshold frequency discussed previously.
It is mandatory to provide these two values.
After classifying the edges for the given image, we display it in a new window using the cv2.imshow()
function. The cv2.waitkey(0)
function is used in the example to prevent the interpreter from closing the created window automatically and waiting for the user to press some key.
The cv2.destroyAllWindows()
function closes all the windows.
The cv2.canny()
function also accepts two additional optional parameters called apertureSize
and L2gradient
. The apertureSize
parameter specifies the size of the aperture for the Sobel kernel.
By default, its value is three and can take any odd value between three and five. We can increase the apertureSize
to get more features from the image.
The L2gradient
takes a True
or False
value, defaulting it as False
.
If we specify the L2gradient
parameter as True
, then the new L2Gradient
algorithm is used to calculate the gradient value; otherwise, the traditional equation is used. The new algorithm tends to be a little more accurate.
Conclusion
We discussed how to use the cv2.canny()
function in this tutorial. We discussed the Canny Edge detection algorithm and how this function implements it internally.
The function was demonstrated with an example. We also discussed the parameters of this function.
Some were mandatory, threshold1
and threshold2
, while the others were optional, apertureSize
and L2gradient
.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn