The HSV Color Space Using OpenCV in Python
- HSV Color Space
- Convert Image to HSV Color Space in OpenCV
- Detect Color Using HSV Color Space in OpenCV
- Conclusion
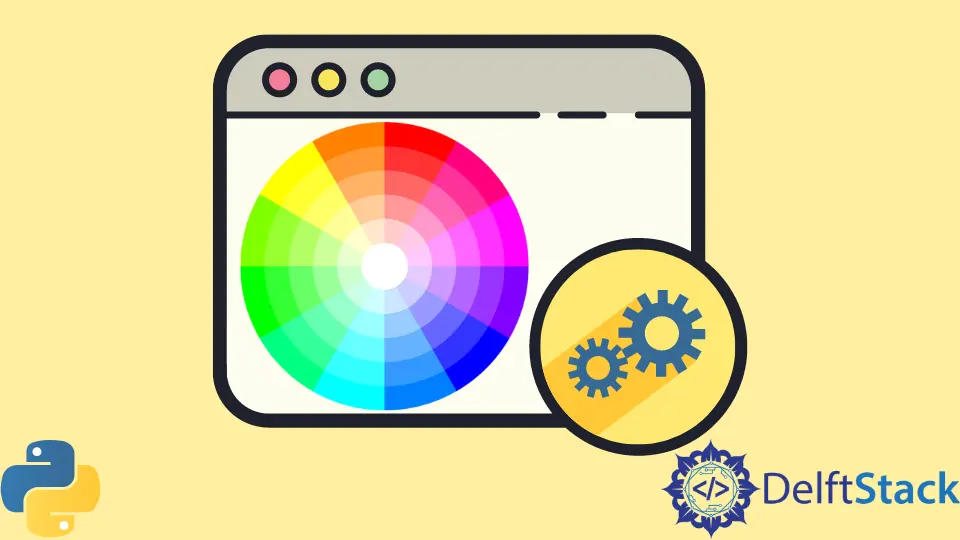
When processing images in programming, we convert them to variables with numeric values and work on such values. Every image can have some desired colors.
We use color models to map these colors to numeric values. Some common color models are RGB, CMYK, HSV, and more.
We can use the opencv
library to process and work with images and videos in Python. This library is full of functions that can implement complex algorithms and techniques for images.
This tutorial will discuss the HSV color space in the opencv
library.
HSV Color Space
By default, the RGB color model represents colors in images. This is because RGB is the most commonly used color model in graphics and can represent a vast spectrum of colors.
However, one of the most useful models is the HSV model.
The HSV model is used to represent the color ranges of the RGB model in a cylindrical shape. This color space is used highly for object tracking.
The HSV color model stands for Hue
, Saturation
, and Brightness
(Value
).
The Hue
attribute is used to specify the tint of the color being used, and the Saturation
attribute determines the amount in which the color is used. The Value
attribute is used to specify the brightness of the color.
Convert Image to HSV Color Space in OpenCV
Using the opencv
library, we can convert images from one color space to another. For this, we use the cvtColor()
function.
When an image is read using the imread()
function, it is in the RGB color space. We can convert this to HSV using the cvtColor()
function.
See the following example.
import cv2
i = cv2.imread("deftstack.png")
img = cv2.cvtColor(i, cv2.COLOR_BGR2HSV)
cv2.imshow("Final", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
Output:
In the above example, note the cv2.BGR2HSV
attribute specified in the cvtColor()
function. It specifies that we are converting the image from the RGB color space to the HSV.
The combination of the waitKey()
and destroyAllWindows()
function is used to wait for the user to press some key before closing the output window.
The cv2.BGR2HSV
converts the image from RGB to HSV with an H
range of 0 to 180. We can also use the cv2.BR2HSV_FULL
to convert the image to HSV with an H
range of 0 to 255.
Detect Color Using HSV Color Space in OpenCV
As discussed, this color space’s main use is for object tracking. We can use this model to create masks that can map some specific colors from an image.
Every color in the HSV model has some color range. The upper and lower bounds of their range can be used to detect colors.
We can use the inRange()
function to return a binary mask that detects a given color using its upper and lower bounds of the HSV color space.
See the code below.
import cv2
import numpy as np
img = cv2.imread("img3.jpeg")
hsv_img = cv2.cvtColor(img, cv2.COLOR_BGR2HSV)
bound_lower = np.array([25, 20, 20])
bound_upper = np.array([100, 255, 255])
mask_green = cv2.inRange(hsv_img, bound_lower, bound_upper)
cv2.imshow("Mask", mask_green)
cv2.waitKey(0)
cv2.destroyAllWindows()
Output:
In the above example, we create a binary mask to detect the green color in an image. The image is converted to the HSV color space, and the range for the green color is defined.
We use the upper and lower bounds with the inRange
function to detect the color and return the mask.
This mask can be mapped onto the image to detect the color and draw the boundaries for the given color.
Conclusion
This tutorial discussed color spaces and focused on the HSV color space in opencv
.
We started by discussing the basics of the HSV color space and how color is mapped in this model. The difference to the commonly used RGB model was also highlighted.
We also demonstrated how to convert an image to HSV using opencv
.
The HSV color space is used for object tracking as well. We discussed how to use it to detect color in a given image using the upper and lower bounds of the given color with the inRange
function from the opencv
library.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn