How to Delete Files and Directories Using Python
-
Delete Files Using
os
Module in Python -
Delete Files Using
pathlib
Module in Python - Delete Files With Pattern Matching in Python
-
Delete Directories Using Python
os
Module -
Delete Directories Using Python
pathlib
Module -
Delete Non-Empty Directories Using Python
shutil.rmtree
Method
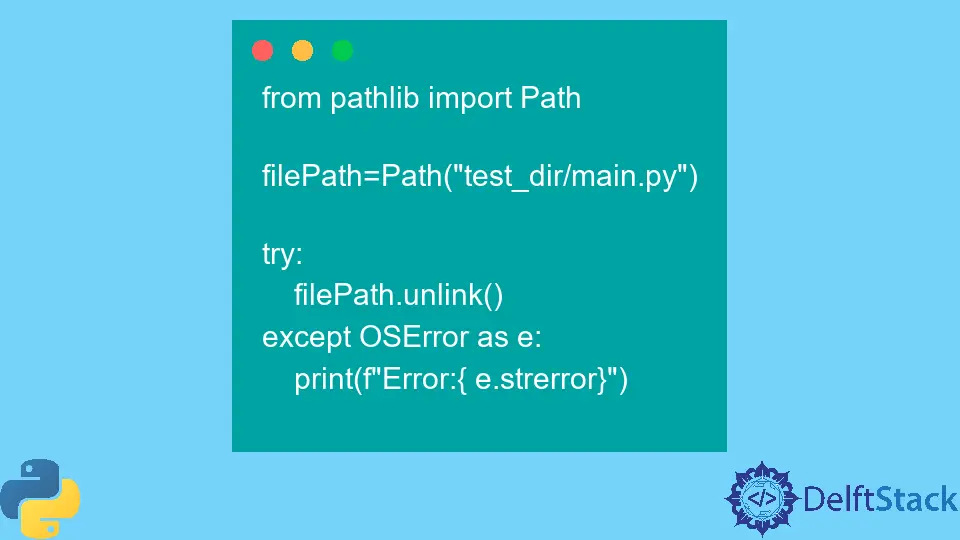
This article introduces how to delete files and directories with Python built-in packages such as os
, pathlib
and shutil
.
Delete Files Using os
Module in Python
os
is an inbuilt package available in both Python 2 and Python 3.
We can use remove()
and unlink()
from os
module to delete files using Python. Both of these functions are similar in action. Both of them take the path of the file to be deleted as an argument.
import os
os.remove("test_dir/main.py")
It deletes the file named main.py
located inside test_dir
. As we are using the relative path here, test_dir
must be in the same root folder as that of our Python program. We can also use the absolute file path.
import os
os.unlink("test_dir/main.py")
It also deletes the file named main.py
located inside test_dir
.
Both functions can only delete files. If we give the path of the directory to delete, we get the IsADirectoryError
error.
Similarly, we get FileNotFoundError
if the file is not present in the specified path.
If we do not have permission to delete the file, we get the PermissionError
error.
To tackle these errors while deleting the file, we should use the exception handling.
import os
try:
os.remove("test_dir/main.py")
except OSError as e:
print(f"Error:{ e.strerror}")
Output:
Error:No such file or directory
If there is no such file, we get error printed in output rather than the whole program to be crashed.
Delete Files Using pathlib
Module in Python
To remove files using the pathlib
module, we at first create a Path
object defined in the pathlib
module. Then we use the unlink()
method to delete the file.
from pathlib import Path
filePath = Path("test_dir/main.py")
try:
filePath.unlink()
except OSError as e:
print(f"Error:{ e.strerror}")
Here, we instantiate the filePath
object from the Path
class with the location of the file to be deleted.
pathlib
module is only available in Python 3.4 and above. For Python 2 we need to install the module using pip
.
Delete Files With Pattern Matching in Python
We can use the glob
module to match patterns in files and after collecting all the files that match the pattern, we can delete all of them using any of the methods specified above.
import os
import glob
py_files = glob.glob("test_dir/*.py")
for py_file in py_files:
try:
os.remove(py_file)
except OSError as e:
print(f"Error:{ e.strerror}")
This deletes all the files in the test_dir
directory with extension .py
.
Delete Directories Using Python os
Module
To delte the directories using Python, we can use os
, pathlib
and shutlib
directory. os
and pathlib
can only delete empty directories while shutlib
can remove non-empty directories too.
import os
dirPath = "test_dir"
try:
os.rmdir(dirPath)
except OSError as e:
print(f"Error:{ e.strerror}")
It deletes the empty directory test_dir
in our current working directory.
Delete Directories Using Python pathlib
Module
If the directory is non-empty the directory cannot be removed using this method, we get an output from the program saying Error: Directory not empty
.
We can also use the pathlib
module to remove non-empty directories.
from pathlib import Path
dirPath = Path("test_dir")
try:
dirPath.rmdir()
except OSError as e:
print(f"Error:{ e.strerror}")
It also deletes the empty directory test_dir
in our current working directory.
Delete Non-Empty Directories Using Python shutil.rmtree
Method
To delete non-empty directories using Python , we use the rmtree
method from the shutil
module.
import shutil
dirPath = "test_dir"
try:
shutil.rmtree(dirPath)
except OSError as e:
print(f"Error:{ e.strerror}")
It deletes the directory test_dir
in our current working directory and all of its contents.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedInRelated Article - Python File
- How to Get All the Files of a Directory
- How to Append Text to a File in Python
- How to Check if a File Exists in Python
- How to Find Files With a Certain Extension Only in Python
- How to Read Specific Lines From a File in Python
- How to Check if File Is Empty in Python
Related Article - Python Directory
- How to Get Home Directory in Python
- How to List All Files in Directory and Subdirectories in Python
- How to Fix the No Such File in Directory Error in Python
- How to Get Directory From Path in Python
- How to Execute a Command on Each File in a Folder in Python
- How to Count the Number of Files in a Directory in Python