如何使用 Python 刪除檔案和資料夾
-
使用 Python 中的
os
模組刪除檔案 -
在 Python 中使用
pathlib
模組刪除檔案 - 在 Python 中用模式匹配刪除檔案
-
使用 Python
os
模組刪除資料夾 -
使用 Python
pathlib
模組刪除資料夾 -
使用 Python
shutil.rmtree
方法刪除非空資料夾
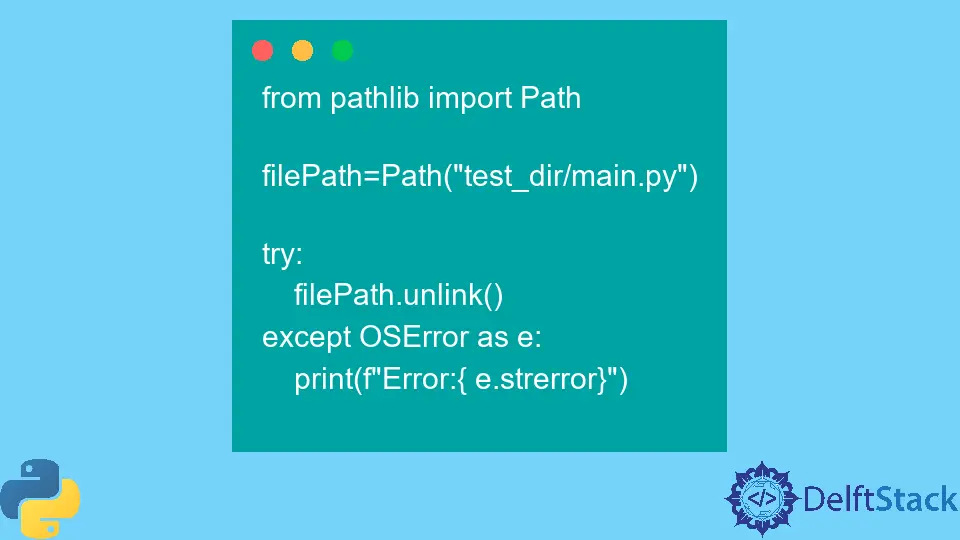
本文介紹瞭如何使用 Python 內建包如 os
、pathlib
和 shutil
來刪除檔案和資料夾。
使用 Python 中的 os
模組刪除檔案
os
是一個內建模組,在 Python 2 和 Python 3 中都可以使用。
我們可以使用 os
模組中的 remove()
和 unlink()
來使用 Python 刪除檔案。這兩個函式的作用是相似的。它們都把要刪除的檔案的路徑作為引數。
import os
os.remove("test_dir/main.py")
它刪除的檔名為 main.py
,位於 test_dir
內。由於我們在這裡使用的是相對路徑,所以 test_dir
必須和我們的 Python 程式在同一個根資料夾下。我們也可以使用絕對檔案路徑。
import os
os.unlink("test_dir/main.py")
它也會刪除位於 test_dir
內的名為 main.py
的檔案。
這兩個函式都只能刪除檔案。如果我們給出要刪除的資料夾的路徑,我們會得到 IsADirectoryError
錯誤。
同樣,如果檔案不存在於指定的路徑中,我們會得到 FileNotFoundError
。
如果我們沒有刪除檔案的許可權,我們會得到 PermissionError
錯誤。
為了在刪除檔案時處理這些錯誤,我們應該使用異常處理。
import os
try:
os.remove("test_dir/main.py")
except OSError as e:
print(f"Error:{ e.strerror}")
輸出:
Error:No such file or directory
如果沒有這樣的檔案,則會在輸出中列印出錯誤,而不是讓整個程式崩潰。
在 Python 中使用 pathlib
模組刪除檔案
為了使用 pathlib
模組刪除檔案,我們首先建立一個在 pathlib
模組中定義的 Path
物件。然後我們使用 unlink()
方法來刪除檔案。
from pathlib import Path
filePath = Path("test_dir/main.py")
try:
filePath.unlink()
except OSError as e:
print(f"Error:{ e.strerror}")
在這裡,我們從 Path
類中例項化出 filePath
物件,其中包含要刪除的檔案的位置。
pathlib
模組只在 Python 3.4 及以上版本中可用。對於 Python 2,我們需要使用 pip
安裝該模組。
在 Python 中用模式匹配刪除檔案
我們可以使用 glob
模組來匹配檔案中的模式,在收集到所有符合模式的檔案後,我們可以使用上面指定的任何方法刪除所有的檔案。
import os
import glob
py_files = glob.glob("test_dir/*.py")
for py_file in py_files:
try:
os.remove(py_file)
except OSError as e:
print(f"Error:{ e.strerror}")
這將刪除 test_dir
資料夾下所有副檔名為 .py
的檔案。
使用 Python os
模組刪除資料夾
要用 Python 刪除資料夾,我們可以使用 os
、pathlib
和 shutlib
資料夾,os
和 pathlib
只能刪除空資料夾,而 shutlib
也可以刪除非空資料夾。os
和 pathlib
只能刪除空資料夾,而 shutlib
也可以刪除非空資料夾。
import os
dirPath = "test_dir"
try:
os.rmdir(dirPath)
except OSError as e:
print(f"Error:{ e.strerror}")
它刪除了我們當前工作資料夾中的空資料夾 test_dir
。
使用 Python pathlib
模組刪除資料夾
如果資料夾是非空的資料夾不能用這個方法刪除,我們就會從程式中得到錯誤輸出- Error: Directory not empty
。
我們也可以使用 pathlib
模組來刪除非空資料夾。
from pathlib import Path
dirPath = Path("test_dir")
try:
dirPath.rmdir()
except OSError as e:
print(f"Error:{ e.strerror}")
它也會刪除我們當前工作資料夾中的空資料夾 test_dir
。
使用 Python shutil.rmtree
方法刪除非空資料夾
要使用 Python 刪除非空資料夾,我們使用 shutil
模組中的 rmtree
方法。
import shutil
dirPath = "test_dir"
try:
shutil.rmtree(dirPath)
except OSError as e:
print(f"Error:{ e.strerror}")
它刪除了我們當前工作資料夾中的 test_dir
資料夾及其所有內容。
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn相關文章 - Python File
- Python 如何得到資料夾下的所有檔案
- Python 如何查詢具有特定副檔名的檔案
- 如何在 Python 中從檔案讀取特定行
- 在 Python 中讀取文字檔案並列印其內容
- 在 Python 中逐行讀取 CSV
- 在 Python 中實現 touch 檔案