在 Python 中讀取文字檔案並列印其內容
Jesse John
2023年1月30日
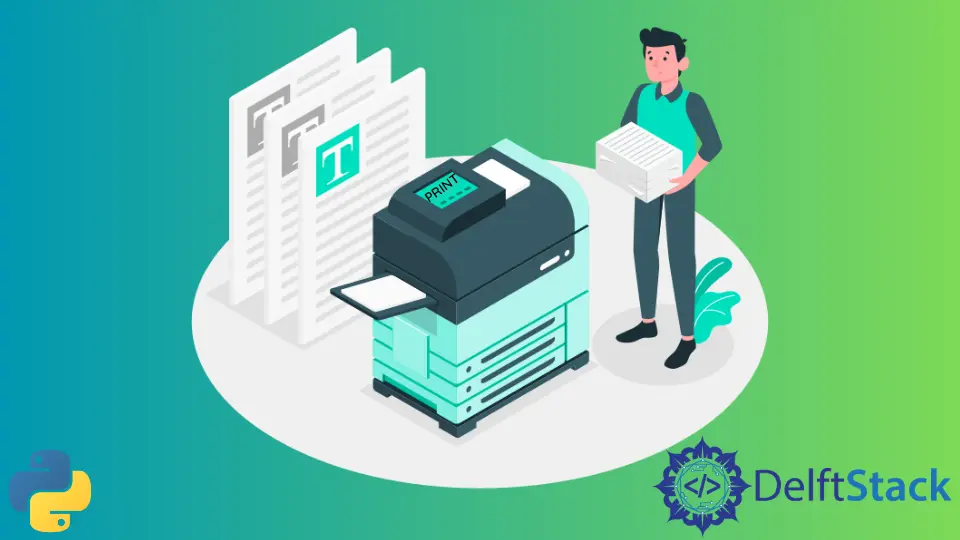
本文將演示如何使用 Python 讀取文字檔案並將其內容列印到螢幕上。
在 Python 中讀取檔案的注意事項
不要開啟二進位制檔案
本文中描述的方法僅適用於文字檔案。Python 在讀取檔案時修改行尾字元;因此,決不能像這樣開啟二進位制檔案。
閱讀有關 Reading and Writing Files 的官方 Python 文件以獲取更多詳細資訊。
避免一次讀取整個檔案
最好一次讀取一行文字檔案,並在讀取下一行之前將每一行列印到螢幕上。這將確保即使大於記憶體的檔案也完全列印到螢幕上。
在 Python 中讀取文字檔案並列印其內容
建立檔案物件
第一步是使用 open()
函式以只讀模式建立檔案物件。第一個引數是檔名和路徑;第二個引數是模式。
該函式還有其他引數,其中最常見的是 encoding
。如果省略,則預設值取決於平臺。
通常使用 encoding = 'utf-8'
。
示例程式碼:
# Create the file object.
# Give the correct filename with path in the following line.
file_object = open("path_to_TEXT_file.txt", "r", encoding="utf-8")
迴圈並列印檔案物件中的字串
Python 提供了一種非常有效的方式來讀取和列印檔案物件的每一行。
一個簡單的 for
迴圈用於此目的。以下程式碼中的單詞 string
只是一個變數名;程式碼迴圈遍歷檔案中的所有行。
示例程式碼:
# Loop over and print each line in the file object.
for string in file_object:
print(string)
解釋:
- 迴圈在每次迭代時列印一行文字檔案。
- 每行在遇到換行符的地方結束。
- 列印完所有行後迴圈終止。
關閉檔案物件
在程式碼列印檔案中的所有行之後,必須關閉物件以釋放記憶體。
示例程式碼:
# Close the file object.
file_object.close()
完整的示例程式碼:
# python3
# coding: utf-8
# Create the file object.
# Give the correct filename with path in the following line.
file_object = open("path_to_TEXT_file.txt", "r", encoding="utf-8")
# Loop over and print each line in the file object.
for string in file_object:
print(string)
# Close the file object.
file_object.close()
作者: Jesse John