在 Python 中读取文本文件并打印其内容
Jesse John
2023年1月30日
Python
Python File
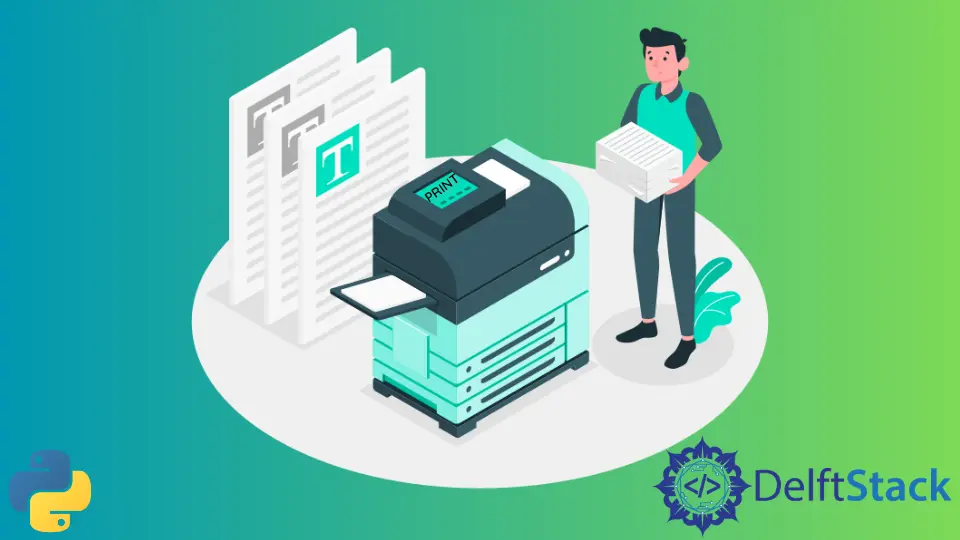
本文将演示如何使用 Python 读取文本文件并将其内容打印到屏幕上。
在 Python 中读取文件的注意事项
不要打开二进制文件
本文中描述的方法仅适用于文本文件。Python 在读取文件时修改行尾字符;因此,决不能像这样打开二进制文件。
阅读有关 Reading and Writing Files 的官方 Python 文档以获取更多详细信息。
避免一次读取整个文件
最好一次读取一行文本文件,并在读取下一行之前将每一行打印到屏幕上。这将确保即使大于内存的文件也完全打印到屏幕上。
在 Python 中读取文本文件并打印其内容
创建文件对象
第一步是使用 open()
函数以只读模式创建文件对象。第一个参数是文件名和路径;第二个参数是模式。
该函数还有其他参数,其中最常见的是 encoding
。如果省略,则默认值取决于平台。
通常使用 encoding = 'utf-8'
。
示例代码:
# Create the file object.
# Give the correct filename with path in the following line.
file_object = open("path_to_TEXT_file.txt", "r", encoding="utf-8")
循环并打印文件对象中的字符串
Python 提供了一种非常有效的方式来读取和打印文件对象的每一行。
一个简单的 for
循环用于此目的。以下代码中的单词 string
只是一个变量名;代码循环遍历文件中的所有行。
示例代码:
# Loop over and print each line in the file object.
for string in file_object:
print(string)
解释:
- 循环在每次迭代时打印一行文本文件。
- 每行在遇到换行符的地方结束。
- 打印完所有行后循环终止。
关闭文件对象
在代码打印文件中的所有行之后,必须关闭对象以释放内存。
示例代码:
# Close the file object.
file_object.close()
完整的示例代码:
# python3
# coding: utf-8
# Create the file object.
# Give the correct filename with path in the following line.
file_object = open("path_to_TEXT_file.txt", "r", encoding="utf-8")
# Loop over and print each line in the file object.
for string in file_object:
print(string)
# Close the file object.
file_object.close()
Enjoying our tutorials? Subscribe to DelftStack on YouTube to support us in creating more high-quality video guides. Subscribe
作者: Jesse John