在 Python 中实现 touch 文件
-
在 Python 中使用
pathlib.Path.touch()
函数实现touch
文件 -
在 Python 中使用
os.utime()
函数实现touch
文件 -
在 Python 中使用触控模块实现
touch
文件
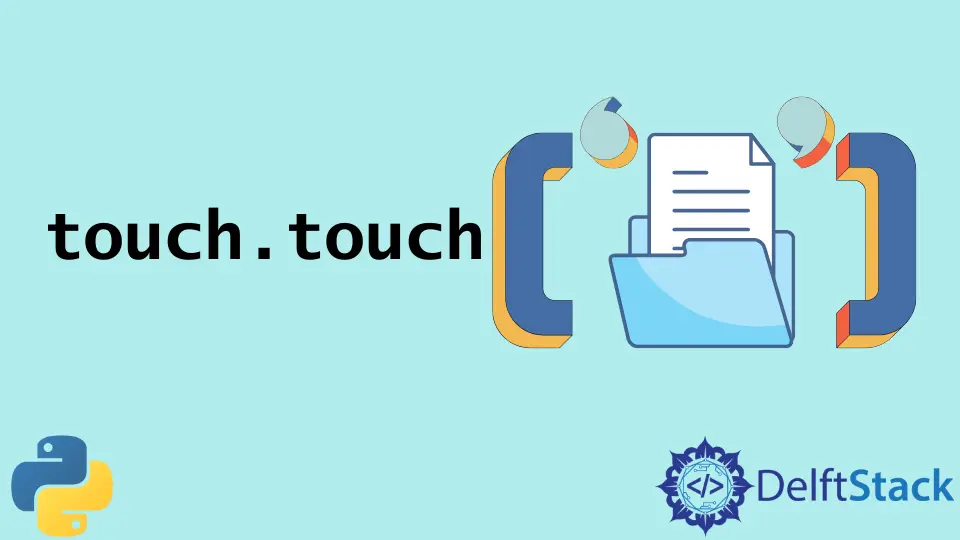
Unix 系统有一个实用命令叫做 touch
。此实用程序将给定文件的访问和修改时间设置为当前时间。
我们将讨论如何在 Python 中实现 touch 文件。
在 Python 中使用 pathlib.Path.touch()
函数实现 touch
文件
pathlib
模块允许我们创建 Path
对象来表示不同的文件系统路径并在操作系统之间工作。
我们可以使用 pathlib.Path.touch()
函数来模拟 touch
命令。它在 Python 中的指定路径创建一个文件。我们使用 mode
参数指定文件模式和访问标志。
它还接受默认为 True 的 exist_ok
参数。如果将其设置为 False,则如果文件已存在于给定路径中,则会引发错误。
请参阅下面的代码。
from pathlib import Path
Path("somefile.txt").touch()
在 Python 中使用 os.utime()
函数实现 touch
文件
os.utime()
函数设置访问和修改时间。我们可以使用 times
参数指定两者的时间。默认情况下,这两个值都设置为当前时间。
我们将创建一个使用 open()
函数打开文件的函数,然后使用 os.time()
函数。该文件将以附加模式打开。
例如,
import os
def touch_python(f_name, times=None):
with open(f_name, "a"):
os.utime(f_name, times)
touch_python("file.txt")
在 Python 中使用触控模块实现 touch
文件
touch 模块是一个第三方模块,可以模拟 Unix touch
命令。我们可以使用它在 Python 中创建 touch
文件。我们将使用带有指定文件名和路径的 touch.touch()
函数。
例如,
import touch
touch.touch("somefile.txt")
这种方法优于其他方法的优点是我们还可以使用它来创建多个文件。为此,我们将文件名及其路径作为列表元素传递。
请参阅以下示例。
import touch
touch.touch(["somefile.txt", "somefile2.txt"])
任何已经存在的文件都将被替换。
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn