How to Implement a Touch File in Python
-
Use the
pathlib.Path.touch()
Function to Implement a Touch File in Python -
Use the
os.utime()
Function to Implement a Touch File in Python -
Use the
touch
Module to Implement a Touch File in Python -
Use the
io.open
Method to Implement a Touch File in Python -
Use the
os.mknod()
Method to Implement a Touch File in Python - Comparison
- Conclusion
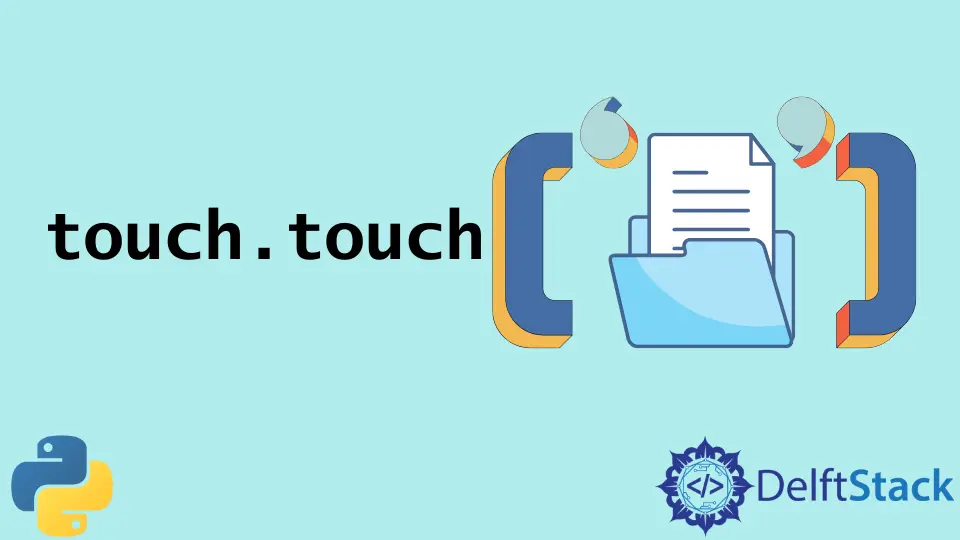
In the vast landscape of programming and scripting, the concept of “touching” a file holds a fundamental role, often serving as a subtle yet powerful operation in various scenarios.
The term “touch” refers to the action of creating an empty file or updating the timestamp of an existing file. This seemingly simple operation finds its significance in a multitude of use cases, ranging from system administration tasks to automation scripts.
Use the pathlib.Path.touch()
Function to Implement a Touch File in Python
In Python, the pathlib
module provides a convenient and object-oriented approach to handle file system paths. The Path
class in pathlib
represents a file system path and provides various methods for interacting with files and directories; one such method is touch()
.
The touch()
method is used to create an empty file at the specified path. If the file already exists, the method updates the file’s access and modification timestamps.
Here’s a basic example:
from pathlib import Path
file_path = Path("example.txt")
file_path.touch()
We first import the Path
class from the pathlib
module. Path
is a powerful class in Python for working with file system paths in an object-oriented way.
Next, a Path
object named file_path
is created, representing the file path example.txt
. This line does not create the actual file on the disk; it simply creates a representation of the file path.
Finally, the touch()
method is called on the file_path
object. This method creates an empty file at the specified path (example.txt
in this case).
In this example, example.txt
will be created in the current working directory if it doesn’t exist. If it already exists, the method will update the access and modification timestamps of the file.
It also accepts an exist_ok
parameter, which is True
by default. If this is set to False
, an error is raised if the file already exists at the given path.
Use the os.utime()
Function to Implement a Touch File in Python
The os.utime()
function can also be used to implement a touch file operation in Python. The os.utime()
function sets the access and modification time.
We can specify the time for both using the times
parameter. By default, both the values are set to the current time.
Below, we will create a function to open a file using the open()
function and then use the os.utime()
function. The file will be opened in append mode.
Example:
import os
def touch_python(file_name, times=None):
with open(file_name, "w"):
os.utime(file_name, times)
touch_python("example.txt")
We first import the os
module to our script, then make our function touch_python
.
Our touch_python
function takes two parameters: file_name
and times
. file_name
is the name of the file.
On the other hand, times
is a tuple containing the access and modification times. If not provided (default is None
), the current time is used.
We then open a file specified by file_name
in write mode ("w"
). If the file already exists, its contents are erased; otherwise, a new empty file is created.
After opening the file, the os.utime
function is called to update the access and modification timestamps. If times
is not provided (remains as None
), the current time is used for both access and modification times.
Finally, we invoke the touch_python
function with the argument "example.txt"
. It creates or updates the file named example.txt
in the current working directory.
In summary, the touch_python
function uses the open
function to create an empty file and then uses os.utime
to update the timestamps of the file, effectively emulating the behavior of the touch
command in Unix-like systems.
Use the touch
Module to Implement a Touch File in Python
The touch
module is a third-party module that can emulate the Unix touch
command. We can use it to create a touch file in Python.
We will use the touch.touch()
function with a specified filename and path.
Note: If you don’t have the
touch
library installed, you can install it using the following command:
pip install touch
Make sure to run this command in your terminal or command prompt.
Syntax:
touch(filename, times=None, ns=None, create=True)
filename
: A string representing the path to the file you want to touch.times
(optional): A tuple containing the access time and modification time. If not specified (default isNone
), the current time is used.ns
(optional): Represents the nanosecond component of the times. If specified, it should be a tuple containing the access time and modification time in nanoseconds.create
(optional): Ifcreate
is set toTrue
(default), the file will be created if it does not exist. If set toFalse
and the file does not exist, the function does nothing.
Here is an example to better understand the function:
# <= Python 3.9
from touch import touch
file_path = "example.txt"
touch(file_path)
In this example, the touch()
function from the touch
library is used to create or update the file specified by file_path
. If the file doesn’t exist, it will be created, and if it exists, its access and modification times will be updated.
touch
package may not work with Python 3.10 due to compatibility issues. Specifically, Python 3.10 removed collections.Iterable
, which touch
relies on, causing an AttributeError
. In Python 3.10, Iterable
was moved to collections.abc
as part of the ongoing effort to organize the standard library. As a result, any package, like touch
, that hasn’t updated its imports to reflect this change will fail. Before using touch
with Python 3.10, check for package updates or consider alternative methods for your task.The advantage of this method over the rest is that we can also use it to create multiple files. For this, we will pass the filename and their paths as elements of a list.
Example:
# <= Python 3.9
from touch import touch
file_paths = ["example.txt", "example2.txt"]
touch(file_paths)
If the files don’t exist, they will be created. The touch
function makes it convenient to apply this operation to multiple files at once.
Use the io.open
Method to Implement a Touch File in Python
The io.open
method provides a versatile and robust way to touch files. In this article, we’ll explore the significance of the touch file operation, followed by a comprehensive guide on implementing it using the io.open
method.
Syntax:
io.open(
file,
mode="r",
buffering=-1,
encoding=None,
errors=None,
newline=None,
closefd=True,
opener=None,
)
file
: The file name or file descriptor to be opened.mode
: The mode in which the file is opened. This is a string containing a combination of characters that represent the file access mode (e.g.,r
for read,w
for write,a
for append).buffering
: An optional integer controlling the buffering policy. Pass0
to disable buffering,1
to line buffering, or any positive integer for a buffer size.encoding
: The name of the encoding to use, e.g.,utf-8
. If this is specified, the file is opened in text mode, and you can specify an encoding for reading or writing the file.errors
: Specifies how encoding and decoding errors are to be handled. The default isstrict
, which raises aUnicodeError
if there’s an error. Other options includeignore
andreplace
.newline
: Controls how universal newlines mode works (only relevant for text mode).None
(the default) means to use the system default, and empty string means always use\n
,\r
, or\r\n
as newline characters.closefd
: Ifclosefd
isTrue
(the default), the underlying file descriptor is closed when the file is closed. IfFalse
, the file descriptor will be kept open.opener
: An optional custom opener. If provided, it must be a callable returning an open file-like object (e.g., a file object returned byos.open
).
Here’s a straightforward example of using io.open
as a touch function:
import io
file_path = "example.txt"
with io.open(file_path, "a", encoding="utf-8"):
pass
print(f"The file '{file_path}' has been touched.")
Output:
The file 'example.txt' has been touched.
First, we import the io
module, which provides advanced file-handling capabilities in Python.
Then we set the variable file_path
to the string example.txt
, representing the path to the file we want to touch.
Now, the main operation lies in the io.open()
. The file specified by file_path
is opened in append mode ('a'
). The utf-8
encoding is explicitly specified for text files.
The with
statement ensures proper handling, automatically closing the file after the operation.
The pass
statement inside the with
block indicates that no additional code is required within the block. The focus is solely on the act of opening the file, not performing specific operations.
This approach provides a clean and efficient solution for the touch file operation in Python, whether creating a new file or updating the timestamp of an existing one.
Use the os.mknod()
Method to Implement a Touch File in Python
The os.mknod()
method in Python is used to create a special file (e.g., a block or character special file) with the specified name and mode. However, it’s important to note that this method is not commonly used for creating regular files, and its usage might not be supported on all platforms.
Here’s an example of using os.mknod()
to create a regular file, effectively implementing a touch functionality:
import os
file_path = "example.txt"
try:
os.mknod(file_path)
except FileExistsError:
pass
We begin by importing the os
module, which provides a way to interact with the operating system, including file-related operations.
The variable file_path
is set to the string 'example.txt'
, representing the path to the file we want to touch.
The core operation is encapsulated within a try
block. os.mknod(file_path)
attempts to create a filesystem node at the specified path (file_path
). The try
block allows us to catch a FileExistsError
in case the file already exists.
Finally, in the except FileExistsError
block, we handle the case where the file already exists. This step is crucial to avoid overwriting an existing file and to maintain the integrity of its content.
It’s important to mention that the use of os.mknod()
for creating regular files might not be as portable as other methods, and it’s generally recommended to use higher-level functions or libraries for file creation and manipulation.
Comparison
- Encoding:
io.open
is suitable when working with text files and needing to specify the character encoding. - File Creation: Both
io.open
andpathlib.touch
can create files if they don’t exist. - Timestamps:
pathlib.touch
andos.utime
are specifically designed for updating timestamps, whereasio.open
can also update timestamps but is more commonly used for file creation. - System Compatibility:
os.mknod
is less common and may not be available on all systems.
Conclusion
Python provides various ways to implement a touch file; choosing the most appropriate method depends on the specific requirements of your task, the Python version you are using, and the desired file manipulation outcome. Each method has its own strengths and use cases, offering flexibility for different programming scenarios.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn