Python os.utime() Method
-
Syntax of
os.utime()
Method -
Example 1: Use the
os.utime()
Method in Python -
Example 2: Use Nanoseconds in the
os.utime()
Method -
Example 3: If
time
IsNone
andns
Is Unspecified in theos.utime()
Method - Conclusion
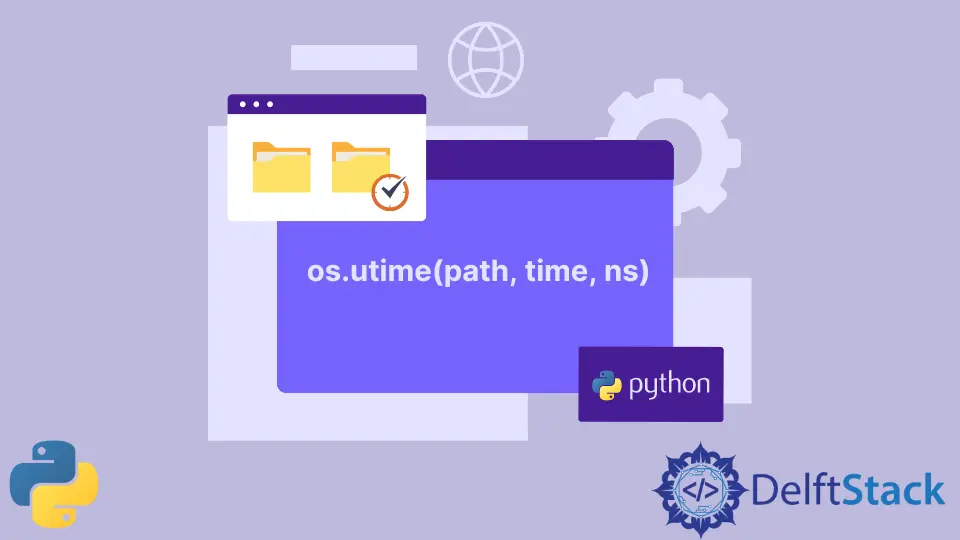
File timestamps play a pivotal role in tracking and managing changes in a file system. The timestamp typically includes information about the last time a file was accessed (atime
) and modified (mtime
).
Understanding and manipulating these timestamps can be vital for various applications, such as version control systems, backup utilities, or synchronization tools.
The Python os.utime()
method is an efficient way of finding the modified time and setting the access times of a specified path.
Python’s os.utime
function provides a convenient way to update these timestamps. It takes a filename and a tuple representing the access time and modification time and then sets the corresponding timestamps for the given file.
Syntax of os.utime()
Method
os.utime(path, time, ns)
Parameters
path |
It is an address object of a file system path or a symlink. The object can either be an str or bytes. |
time |
It is a tuple of two elements (access_time , modified_time ). Each element is an integer/float value representing times in seconds. |
ns |
It is a tuple of two elements (access_time , modified_time ). Each element is an integer/float value representing times in nanoseconds. |
Return
In the execution process, this method does not return any value.
Example 1: Use the os.utime()
Method in Python
In this example, the Python script tackles file manipulation and examines how to retrieve information about a file, specifically focusing on access and modification timestamps.
The code uses the os
module to interact with the file system, providing insights into file statistics and demonstrating the modification of timestamps using os.utime
.
file.txt
:
Sample text file.
Code:
import os
import sys
fd = os.open("file.txt", os.O_RDWR | os.O_CREAT)
# You can provide full path
path = "file.txt"
stinfo = os.stat(path)
print(stinfo)
print("The access time of the file: %s" % stinfo.st_atime)
print("The modified time of the file: %s" % stinfo.st_mtime)
os.utime(path, (1330712280, 1330712292))
print("Changes have been made.")
The code begins by using os.open
to open or create a file named "file.txt"
in read-write mode (os.O_RDWR | os.O_CREAT
).
The variable path
is assigned the absolute path to the file file.txt
. Meaning that the file directory is the same as where the script file is located.
os.stat
is used to retrieve statistical information about the file, storing the results in the stinfo
variable.
Then, the code prints the detailed file information obtained from os.stat
, providing insights into various attributes of the file. Also, the access time (st_atime
) and modification time (st_mtime
) of the file are printed, showcasing the original timestamps.
Next, the code utilizes os.utime
to set custom timestamps (1330712280
for access time and 1330712292
for modification time) for the specified file.
Lastly, a message is printed to confirm that changes to the file’s timestamps have been made.
Output:
The output will include the original file information, access time, and modification time.
After the os.utime
operation, the output will confirm that changes have been made, reflecting the updated timestamps. The specific timestamps will depend on the values provided to os.utime
.
When using the os.utime()
method, ensure you either enter the time
parameter or the ns
parameter. It is an error to set a value for both parameters.
Example 2: Use Nanoseconds in the os.utime()
Method
In this code, we explore the manipulation of file timestamps with nanosecond precision using the os
module.
The code demonstrates how to open or create a file, retrieve its current access and modification times, and subsequently update these timestamps with specified nanosecond values using the os.utime
function.
file.txt
:
Sample text file.
Code:
import os
import sys
fd = os.open("file.txt", os.O_RDWR | os.O_CREAT)
path = "file.txt"
print("The current access time, in seconds:", os.stat(path).st_atime)
print("The current modification time, in seconds:", os.stat(path).st_mtime)
access_time_ns = 20000000012345
modified_time_ns = 10000000012345
tuples = (access_time_ns, modified_time_ns)
os.utime(path, ns=tuples)
print("The access and modification times have been changed.")
The script begins by using os.open
to open or create a file named file.txt
in read-write mode (os.O_RDWR | os.O_CREAT
).
The variable path
is assigned the relative path to the file "file.txt"
.
Next, the code prints the current access time and modification time of the file in seconds using os.stat(path).st_atime
and os.stat(path).st_mtime
, respectively.
Then, nanosecond values for the access and modification times are defined and stored in the tuples
variable.
The os.utime
function is used with the ns
argument to update the file’s timestamps with nanosecond precision.
Lastly, a message is printed to confirm that the access and modification times have been changed.
Output:
The output will include the original access and modification times of the file in seconds
After updating the timestamps with nanosecond precision using os.utime
, the confirmation message will be displayed, indicating that the changes have been successfully made.
The specific nanosecond values will depend on the provided values (access_time_ns and modified_time_ns
). The ns
is a keyword for the argument of the tuple.
No time in nanoseconds can be set without using the ns
keyword.
Example 3: If time
Is None
and ns
Is Unspecified in the os.utime()
Method
In this example, we will demonstrate the behavior of the os.utime()
method when the time
parameter is set to None
and the ns
parameter is left unspecified.
The code demonstrates the default behavior of os.utime()
in such cases and explores how the timestamps of a file are affected.
file.txt
:
Sample text file.
Code:
import os
import sys
fd = os.open("file.txt", os.O_RDWR | os.O_CREAT)
path = "file.txt"
print("The current access time, in seconds:", os.stat(path).st_atime)
print("The current modification time, in seconds:", os.stat(path).st_mtime)
os.utime(path)
print("The access and modification times have been changed.")
print("The current access time, in seconds:", os.stat(path).st_atime)
print("The current modification time, in seconds:", os.stat(path).st_mtime)
The code above focuses on file manipulation using the os
module. It initiates by opening or creating a file named "file.txt"
in read-write mode.
The file path is then specified and assigned to the variable 'path'
.
Subsequently, the script prints the current access and modification times of the file in seconds, employing os.stat(path).st_atime
and os.stat(path).st_mtime
.
The os.utime()
method is then utilized without explicitly specifying values for the time and ns
parameters, resulting in the use of the current system time as the default for time.
Following this operation, a confirmation message is displayed, affirming the successful modification of access and modification times.
Finally, the script prints the updated access and modification times to observe the changes made by the os.utime()
operation.
Output:
In the above code, the time
is None
by default, and ns
is unspecified.
The output will include the original access and modification times of the file in seconds. After the os.utime()
operation with default arguments, the confirmation message will be displayed, indicating that the changes have been successfully made.
So by default, the os.utime()
method will take the current time in nanoseconds as the parameters.
The updated access and modification times will then be printed, showcasing the impact of the os.utime()
method on the file timestamps when default values are used.
Conclusion
In conclusion, file timestamps are essential for tracking and managing changes in a file system, providing crucial information about access and modification times.
Python’s os.utime()
method proves invaluable for efficiently managing these timestamps. The flexibility of the method is demonstrated through various examples.
The first showcases basic usage, the second introduces nanosecond precision, and the third explores default behavior when time
is set to None
and ns
is unspecified.
Developers can leverage this method for diverse applications, from version control to synchronization tools, ensuring precise control over file timestamp management in Python.
Musfirah is a student of computer science from the best university in Pakistan. She has a knack for programming and everything related. She is a tech geek who loves to help people as much as possible.
LinkedIn