How to Read Specific Lines From a File in Python
- Using fileobject.readlines() for Small Files
- Using linecache for Repeated Access
- Using enumerate() for Large Files
- Conclusion
- FAQ
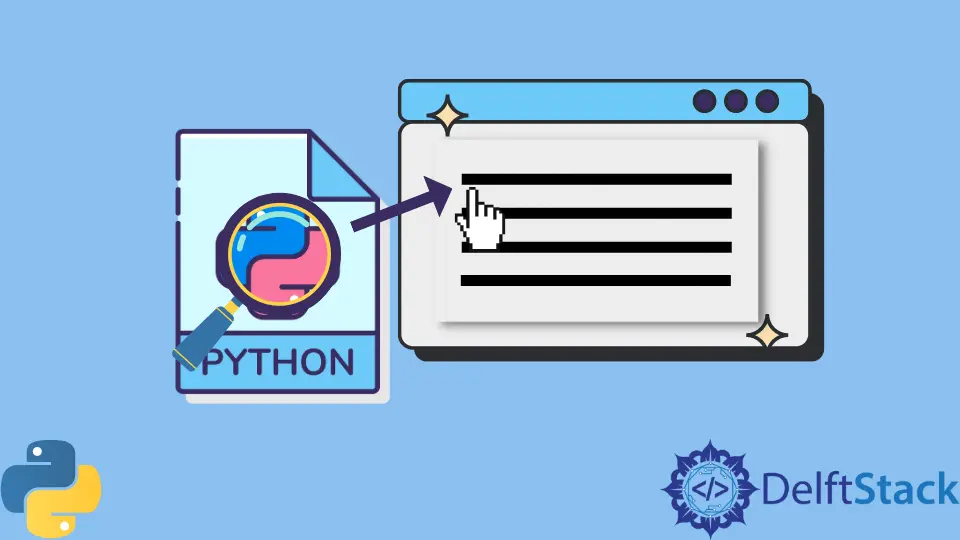
When working with files in Python, you might often find yourself needing to extract specific lines from a text file. Whether you’re processing a small configuration file or analyzing a large dataset, Python offers several methods to efficiently read specific lines.
In this article, we will explore different techniques, such as using fileobject.readlines()
for smaller files, linecache
for repeated access to specific lines, and enumerate()
for larger files. Each method serves a unique purpose, making it easier for you to choose the right approach based on your needs. Let’s dive into these methods and see how they can simplify your file handling tasks.
Using fileobject.readlines() for Small Files
The readlines()
method is a straightforward way to read all lines from a file into a list. This method is particularly effective for smaller files, where loading the entire content into memory is not a concern. Here’s how you can use it:
with open('example.txt', 'r') as file:
lines = file.readlines()
specific_line = lines[2] # Accessing the third line (index 2)
print(specific_line)
Output:
This is the third line of the file.
In this example, we open a file named example.txt
in read mode. The readlines()
method reads all lines and stores them in a list called lines
. To access a specific line, you simply use the index of that line. Remember that indexing in Python starts at zero, so lines[2]
retrieves the third line. This method is simple and effective for files with a manageable number of lines, allowing you to easily access any line by its index. However, for larger files, this approach may not be optimal since it loads the entire file into memory.
Using linecache for Repeated Access
If you need to read specific lines from a file multiple times, the linecache
module is an excellent choice. It allows you to access lines by their line number without reading the entire file into memory. This can be particularly useful for large files where performance is a concern. Here’s how to use linecache
:
import linecache
specific_line = linecache.getline('example.txt', 3) # Accessing the third line
print(specific_line)
Output:
This is the third line of the file.
In this snippet, we import the linecache
module and use the getline()
function to retrieve the third line from example.txt
. The advantage of using linecache
is that it caches the file contents, making subsequent calls to read the same line much faster. This is particularly beneficial when you need to access the same lines repeatedly, as it reduces the overhead of reading the file multiple times. However, keep in mind that linecache
reads the entire file into memory the first time it is accessed, so for very large files, it might still consume a considerable amount of memory.
Using enumerate() for Large Files
For large files where memory efficiency is critical, the enumerate()
function can be a game-changer. This method allows you to iterate over the file line by line, keeping memory usage low. Here’s how to implement it:
with open('example.txt', 'r') as file:
for index, line in enumerate(file):
if index == 2: # Accessing the third line
specific_line = line
break
print(specific_line)
Output:
This is the third line of the file.
In this example, we open example.txt
and use enumerate()
to loop through each line along with its index. When the index matches the desired line number (in this case, the third line), we store it in specific_line
and break out of the loop. This method is particularly efficient for large files as it reads one line at a time, significantly reducing memory consumption. You only load the lines you need into memory, making it a perfect choice for processing large datasets or logs.
Conclusion
Reading specific lines from a file in Python can be accomplished in various ways, each suited to different scenarios. Whether you’re handling small files with readlines()
, accessing lines multiple times with linecache
, or efficiently processing large files with enumerate()
, Python provides the tools to make your file handling tasks easier. By understanding these methods, you can choose the best approach for your specific needs and enhance the performance of your applications.
FAQ
-
What is the best method to read specific lines from a small file?
The best method for small files is to usefileobject.readlines()
, as it loads the entire content into memory for easy access. -
How does
linecache
improve performance?
linecache
caches the contents of the file after the first read, allowing for faster access to specific lines without re-reading the file. -
Is
enumerate()
suitable for large files?
Yes,enumerate()
is ideal for large files as it reads one line at a time, minimizing memory usage. -
Can I use these methods for binary files?
These methods are primarily designed for text files. For binary files, you would need to use different approaches tailored for binary data.
- How do I handle errors when accessing lines that may not exist?
You can use try-except blocks to catchIndexError
for methods that use indexing or check if the line number is within the file’s range when usinglinecache
.