How to Check if File Is Empty in Python
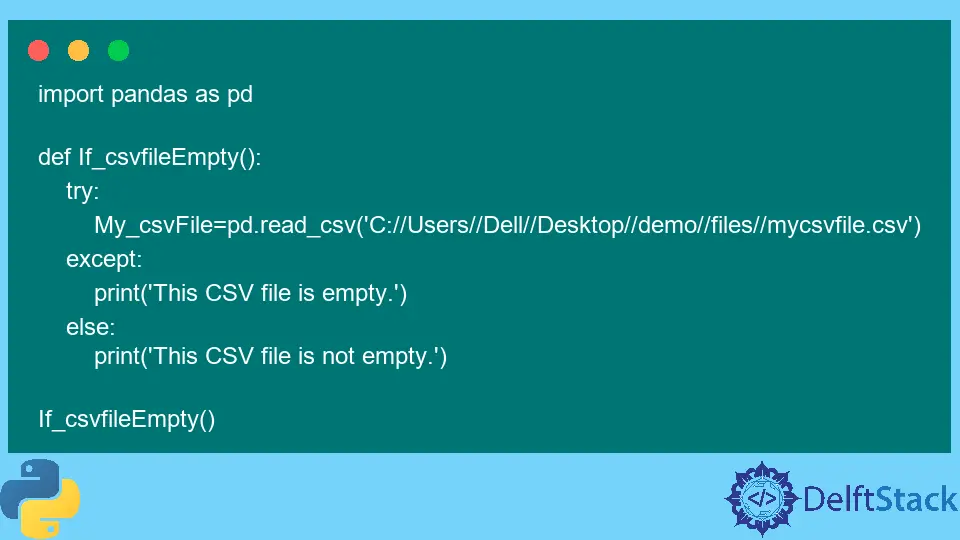
We will learn how to check whether files are empty or not. We also have a look at different issues that were raised while checking an empty file in Python.
Check Empty Text File in Python
If you are doing batch processing or processing that part of a bigger process, you will not be putting any output to a screen. You would have different alerts to let you know what is going on so you can interrupt and fix things.
But for this demonstration, we have created three files, csv
, text
and excel
; all three are empty. We want to show you that we enter the code very quickly.
In our case, the file sizes have 0 kb because our files are empty, but in your case, sizes can be different even though you have an empty file. File sizes are important when we check whether a file is empty because we use the file size in our Python code.
Now we will jump into the code to test whether a text file is empty or not, and to test; we will import the Path
class from the pathlib
module. The Path
class gives us access to the directory of stroke paths on either our local drive or potentially on our network drive, or wherever the actual path to the file is.
First, we define a function called If_TextFileEmpty()
and create a variable called my_file
inside the function. We will call the Path()
class and define a file’s path; we put r
before the string to avoid a unicode error.
my_file = Path(r"C:\Users\Dell\Desktop\demo\files\Mytextfile.txt")
Now we will define a basic check whether either file does exist using the is_file()
method. We will define the next block to check whether a file is empty using the getsize()
function from the os
module.
from pathlib import Path
import os
def If_TextFileEmpty():
my_file = Path(r"C:\Users\Dell\Desktop\demo\files\Mytextfile.txt")
if my_file.is_file():
print("This file exists, ok!")
else:
print("This file does not exists, make sure are putting correct path")
if os.path.getsize(my_file) > 0:
print("This file is not an empty file, process done!")
else:
print("This is an empty file.")
If_TextFileEmpty()
In both cases, if the if
statement does not find a True
value, then the else
block will be executed.
This file exists, ok!
This is an empty file.
Check Empty Excel File in Python
There is a problem with the above code; we have an empty file, but its size is not 8kb. It is seen with CSV
or Excel
files.
Since we have an Excel file and its size is 8kb, we will use a different approach.
To use this approach, we will import the pandas
library and call the read_excel()
method that reads an Excel file. Using the if
statement, we will use a property called empty
to check whether this Excel file is empty and return a Boolean value.
import pandas as pd
def IsExcelFileEmpty():
My_Excelfile = pd.read_excel(
"C://Users//Dell//Desktop//demo//files//myexcelfile.xlsx"
)
if My_Excelfile.empty:
print("This excel file is empty.")
else:
print("This excel file is not empty.")
IsExcelFileEmpty()
Output:
This excel file is empty.
Check Empty CSV File in Python
But the above code does not work with the csv
file and raises an error because this is an empty file, and we are instructing Python about reading this file. In this case, it will start reading, find no column, and raise an exception.
File "pandas\_libs\parsers.pyx", line 549, in pandas._libs.parsers.TextReader.__cinit__
pandas.errors.EmptyDataError: No columns to parse from file
We will catch this exception in the try
block. We do not need to use an empty
property; obviously, it will go into the except
block if the try
block catches an exception.
import pandas as pd
def If_csvfileEmpty():
try:
My_csvFile = pd.read_csv("C://Users//Dell//Desktop//demo//files//mycsvfile.csv")
except:
print("This CSV file is empty.")
else:
print("This CSV file is not empty.")
If_csvfileEmpty()
Output:
This CSV file is empty.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn