Python에서 파일이 비어 있는지 확인
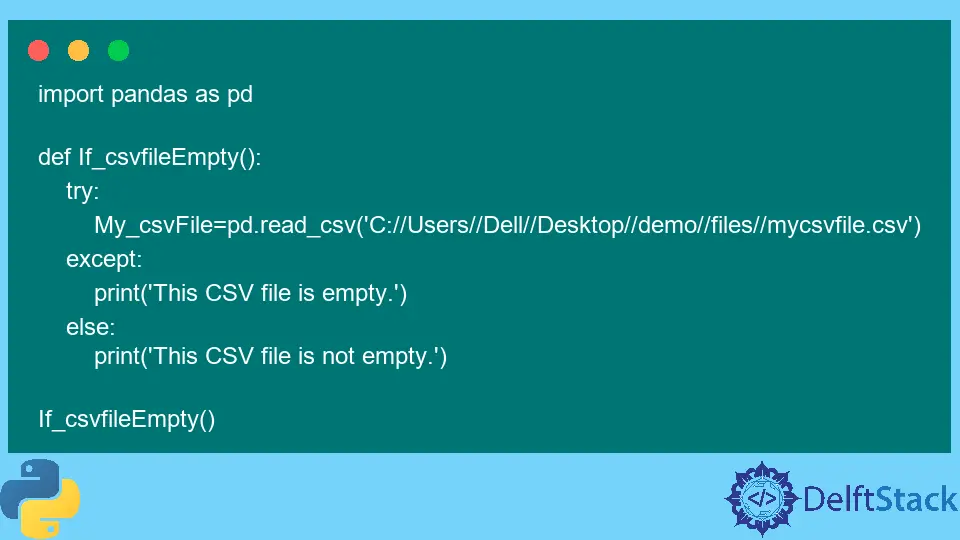
파일이 비어 있는지 확인하는 방법을 배웁니다. 또한 Python에서 빈 파일을 확인하는 동안 제기된 다양한 문제를 살펴봅니다.
Python에서 빈 텍스트 파일 확인
일괄 처리를 수행하거나 더 큰 프로세스의 해당 부분을 처리하는 경우 화면에 출력을 표시하지 않습니다. 무슨 일이 일어나고 있는지 알려주는 다른 경고가 있으므로 문제를 중단하고 수정할 수 있습니다.
하지만 이 데모에서는 csv
, text
및 excel
이라는 세 개의 파일을 만들었습니다. 세 개 모두 비어 있습니다. 우리는 코드를 매우 빠르게 입력한다는 것을 보여주고 싶습니다.
우리의 경우 파일이 비어 있기 때문에 파일 크기가 0kb이지만 귀하의 경우에는 빈 파일이 있더라도 크기가 다를 수 있습니다. 파일 크기는 Python 코드에서 파일 크기를 사용하기 때문에 파일이 비어 있는지 여부를 확인할 때 중요합니다.
이제 코드로 이동하여 텍스트 파일이 비어 있는지 여부를 테스트하고 테스트합니다. pathlib
모듈에서 Path
클래스를 가져올 것입니다. Path
클래스는 로컬 드라이브나 잠재적으로 네트워크 드라이브, 또는 파일의 실제 경로가 있는 위치에 있는 스트로크 경로 디렉토리에 대한 액세스를 제공합니다.
먼저 If_TextFileEmpty()
라는 함수를 정의하고 함수 내부에 my_file
이라는 변수를 만듭니다. Path()
클래스를 호출하고 파일의 경로를 정의합니다. 유니코드 오류를 피하기 위해 문자열 앞에 r
을 넣습니다.
my_file = Path(r"C:\Users\Dell\Desktop\demo\files\Mytextfile.txt")
이제 is_file()
메서드를 사용하여 두 파일 중 하나가 존재하는지 여부에 대한 기본 검사를 정의합니다. os
모듈의 getsize()
함수를 사용하여 파일이 비어 있는지 확인하는 다음 블록을 정의합니다.
from pathlib import Path
import os
def If_TextFileEmpty():
my_file = Path(r"C:\Users\Dell\Desktop\demo\files\Mytextfile.txt")
if my_file.is_file():
print("This file exists, ok!")
else:
print("This file does not exists, make sure are putting correct path")
if os.path.getsize(my_file) > 0:
print("This file is not an empty file, process done!")
else:
print("This is an empty file.")
If_TextFileEmpty()
두 경우 모두 if
문이 True
값을 찾지 못하면 else
블록이 실행됩니다.
This file exists, ok!
This is an empty file.
Python에서 빈 Excel 파일 확인
위의 코드에 문제가 있습니다. 빈 파일이 있지만 크기가 8kb가 아닙니다. CSV
또는 Excel
파일에서 볼 수 있습니다.
Excel 파일이 있고 크기가 8kb이므로 다른 접근 방식을 사용합니다.
이 접근 방식을 사용하기 위해 pandas
라이브러리를 가져오고 Excel 파일을 읽는 read_excel()
메서드를 호출합니다. if
문을 사용하여 empty
라는 속성을 사용하여 이 Excel 파일이 비어 있는지 확인하고 부울 값을 반환합니다.
import pandas as pd
def IsExcelFileEmpty():
My_Excelfile = pd.read_excel(
"C://Users//Dell//Desktop//demo//files//myexcelfile.xlsx"
)
if My_Excelfile.empty:
print("This excel file is empty.")
else:
print("This excel file is not empty.")
IsExcelFileEmpty()
출력:
This excel file is empty.
Python에서 빈 CSV 파일 확인
그러나 위의 코드는 csv
파일에서 작동하지 않고 빈 파일이기 때문에 오류를 발생시키며 Python에 이 파일을 읽도록 지시합니다. 이 경우 읽기를 시작하고 열을 찾지 못하고 예외가 발생합니다.
File "pandas\_libs\parsers.pyx", line 549, in pandas._libs.parsers.TextReader.__cinit__
pandas.errors.EmptyDataError: No columns to parse from file
try
블록에서 이 예외를 포착합니다. 빈
속성을 사용할 필요가 없습니다. 분명히 try
블록이 예외를 포착하면 except
블록으로 이동합니다.
import pandas as pd
def If_csvfileEmpty():
try:
My_csvFile = pd.read_csv("C://Users//Dell//Desktop//demo//files//mycsvfile.csv")
except:
print("This CSV file is empty.")
else:
print("This CSV file is not empty.")
If_csvfileEmpty()
출력:
This CSV file is empty.
Hello! I am Salman Bin Mehmood(Baum), a software developer and I help organizations, address complex problems. My expertise lies within back-end, data science and machine learning. I am a lifelong learner, currently working on metaverse, and enrolled in a course building an AI application with python. I love solving problems and developing bug-free software for people. I write content related to python and hot Technologies.
LinkedIn