How to Append Text to a File in Python
-
file.write
to Append Text to a File Witha
Mode -
Add Optional
file
Parameter to theprint
Function in Python 3 - Add New Line in Appending Text to a File
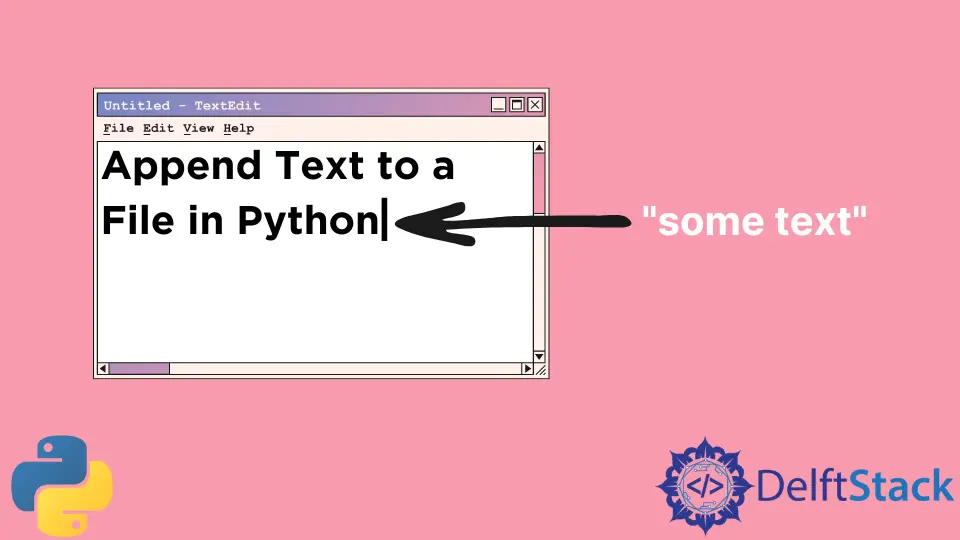
This tutorial article will introduce how to append text to a file in Python.
file.write
to Append Text to a File With a
Mode
You could open the file in a
or a+
mode if you want to append text to a file.
destFile = r"temp.txt"
with open(destFile, "a") as f:
f.write("some appended text")
The code above appends the text some appended text
next to the last character in the file. For example, if the file ends with this is the last sentence
, then it becomes this is the last sentencesome appended text
after appending.
It will create the file if the file doesn’t exist in the given path.
Add Optional file
Parameter to the print
Function in Python 3
In Python 3, you could print the text to the file with the optional file
parameter enabled.
destFile = r"temp.txt"
Result = "test"
with open(destFile, "a") as f:
print("The result will be {}".format(Result), file=f)
Add New Line in Appending Text to a File
If you prefer to add the text in the new line, you need to add the carriage break \r\n
after the appended text to guarantee the next appended text will be added to the new line.
destFile = r"temp.txt"
with open(destFile, "a") as f:
f.write("the first appended text\r\n")
f.write("the second appended text\r\n")
f.write("the third appended text\r\n")
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook