在 Python 中實現 touch 檔案
-
在 Python 中使用
pathlib.Path.touch()
函式實現touch
檔案 -
在 Python 中使用
os.utime()
函式實現touch
檔案 -
在 Python 中使用觸控模組實現
touch
檔案
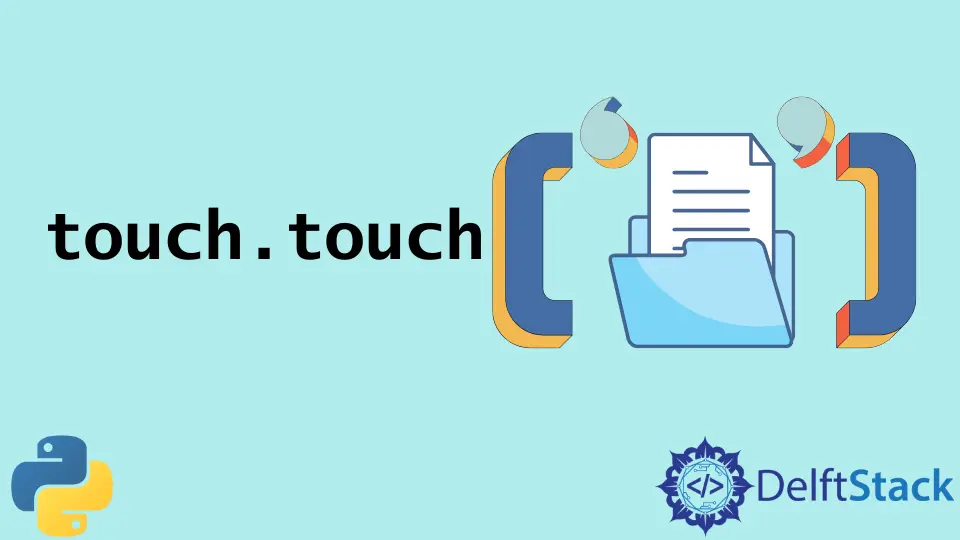
Unix 系統有一個實用命令叫做 touch
。此實用程式將給定檔案的訪問和修改時間設定為當前時間。
我們將討論如何在 Python 中實現 touch 檔案。
在 Python 中使用 pathlib.Path.touch()
函式實現 touch
檔案
pathlib
模組允許我們建立 Path
物件來表示不同的檔案系統路徑並在作業系統之間工作。
我們可以使用 pathlib.Path.touch()
函式來模擬 touch
命令。它在 Python 中的指定路徑建立一個檔案。我們使用 mode
引數指定檔案模式和訪問標誌。
它還接受預設為 True 的 exist_ok
引數。如果將其設定為 False,則如果檔案已存在於給定路徑中,則會引發錯誤。
請參閱下面的程式碼。
from pathlib import Path
Path("somefile.txt").touch()
在 Python 中使用 os.utime()
函式實現 touch
檔案
os.utime()
函式設定訪問和修改時間。我們可以使用 times
引數指定兩者的時間。預設情況下,這兩個值都設定為當前時間。
我們將建立一個使用 open()
函式開啟檔案的函式,然後使用 os.time()
函式。該檔案將以附加模式開啟。
例如,
import os
def touch_python(f_name, times=None):
with open(f_name, "a"):
os.utime(f_name, times)
touch_python("file.txt")
在 Python 中使用觸控模組實現 touch
檔案
touch 模組是一個第三方模組,可以模擬 Unix touch
命令。我們可以使用它在 Python 中建立 touch
檔案。我們將使用帶有指定檔名和路徑的 touch.touch()
函式。
例如,
import touch
touch.touch("somefile.txt")
這種方法優於其他方法的優點是我們還可以使用它來建立多個檔案。為此,我們將檔名及其路徑作為列表元素傳遞。
請參閱以下示例。
import touch
touch.touch(["somefile.txt", "somefile2.txt"])
任何已經存在的檔案都將被替換。
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn