How to Convert JSON to CSV in Python
- Method 1: Using the pandas Library
- Method 2: Using the csv and json Libraries
- Method 3: Handling Nested JSON
- Conclusion
- FAQ
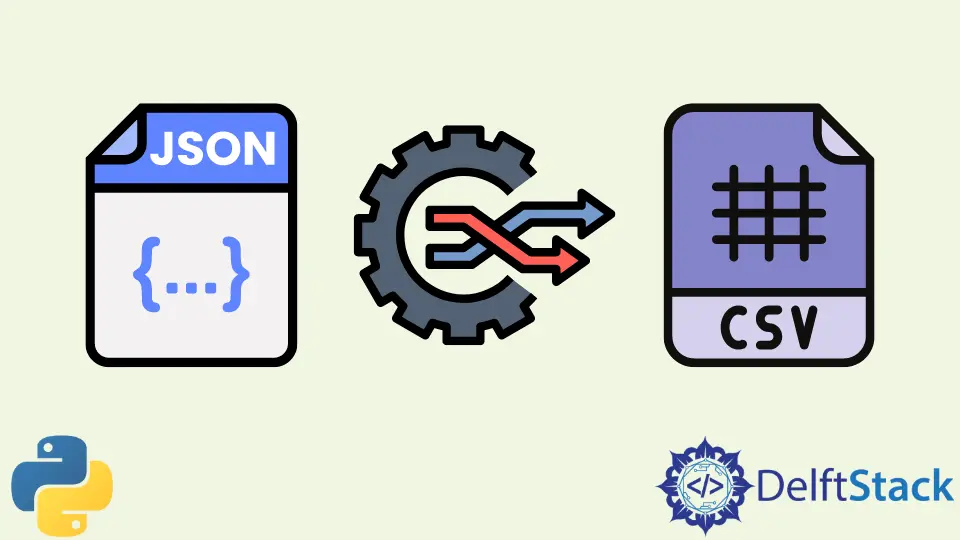
Converting JSON to CSV in Python is a common task for data analysts and developers alike. JSON, or JavaScript Object Notation, is widely used for data interchange on the web due to its human-readable format. On the other hand, CSV, or Comma-Separated Values, is a simpler format that is often used for data storage and analysis in spreadsheets.
In this tutorial, we will walk through the steps to convert JSON data into a CSV file using Python. Whether you’re handling API responses, log files, or any other JSON data, this guide will equip you with the necessary skills to make the conversion seamless. Let’s dive into the methods you can use to achieve this!
Method 1: Using the pandas Library
One of the most efficient ways to convert JSON to CSV in Python is by using the pandas library. Pandas is a powerful data manipulation tool that provides easy-to-use data structures and data analysis tools. If you haven’t installed pandas yet, you can do so using pip:
pip install pandas
Once you have pandas installed, you can use the following code snippet to convert your JSON data to a CSV file:
import pandas as pd
json_data = '''
[
{"name": "John", "age": 30, "city": "New York"},
{"name": "Anna", "age": 22, "city": "London"},
{"name": "Mike", "age": 32, "city": "Chicago"}
]
'''
df = pd.read_json(json_data)
df.to_csv('output.csv', index=False)
Output:
name,age,city
John,30,New York
Anna,22,London
Mike,32,Chicago
In this example, we first import the pandas library and define a JSON string that contains an array of objects. We then use the pd.read_json()
function to read the JSON data into a DataFrame. Finally, we use the to_csv()
method to write the DataFrame to a CSV file named output.csv
. The index=False
parameter ensures that the index column is not included in the output file. This method is straightforward and handles complex JSON structures effectively.
Method 2: Using the csv and json Libraries
If you prefer not to use external libraries, Python’s built-in csv
and json
libraries can also accomplish the task. This method is particularly useful if you want to avoid additional dependencies. Here’s how you can do it:
import json
import csv
json_data = '''
[
{"name": "John", "age": 30, "city": "New York"},
{"name": "Anna", "age": 22, "city": "London"},
{"name": "Mike", "age": 32, "city": "Chicago"}
]
'''
data = json.loads(json_data)
with open('output.csv', mode='w', newline='') as file:
writer = csv.DictWriter(file, fieldnames=data[0].keys())
writer.writeheader()
writer.writerows(data)
Output:
name,age,city
John,30,New York
Anna,22,London
Mike,32,Chicago
In this method, we start by importing the necessary libraries. The JSON string is loaded using json.loads()
, which converts it into a Python list of dictionaries. We then open a new CSV file in write mode and create a csv.DictWriter
object. The fieldnames
parameter is set to the keys of the first dictionary in the list. After writing the header with writeheader()
, we use writerows()
to write all the data to the CSV file. This approach is efficient and works well for simple JSON data structures.
Method 3: Handling Nested JSON
Sometimes, your JSON data might be nested, meaning that some fields contain arrays or other JSON objects. To handle such cases, you can flatten the JSON structure before converting it to CSV. Here’s an example:
import pandas as pd
import json
json_data = '''
[
{"name": "John", "age": 30, "address": {"city": "New York", "zip": "10001"}},
{"name": "Anna", "age": 22, "address": {"city": "London", "zip": "E1 6AN"}},
{"name": "Mike", "age": 32, "address": {"city": "Chicago", "zip": "60601"}}
]
'''
data = json.loads(json_data)
flat_data = []
for item in data:
flat_item = {
'name': item['name'],
'age': item['age'],
'city': item['address']['city'],
'zip': item['address']['zip']
}
flat_data.append(flat_item)
df = pd.DataFrame(flat_data)
df.to_csv('output_flat.csv', index=False)
Output:
name,age,city,zip
John,30,New York,10001
Anna,22,London,E1 6AN
Mike,32,Chicago,60601
In this example, we start with a nested JSON structure where the address
field contains another JSON object. We parse the JSON data and then create a flat list of dictionaries. Each dictionary contains the necessary fields, including those from the nested object. Finally, we convert this flat list into a DataFrame and write it to a CSV file. This method is especially useful for more complex JSON structures where direct conversion would not yield usable results.
Conclusion
Converting JSON to CSV in Python is a straightforward process, whether you choose to leverage libraries like pandas or stick with built-in modules like csv and json. Each method has its strengths, and the choice largely depends on your specific needs and the complexity of your JSON data. By following the examples provided, you can easily handle various JSON structures and create clean CSV files for your data analysis tasks. With practice, this skill will become a valuable addition to your Python toolkit.
FAQ
-
What is the difference between JSON and CSV?
JSON is a lightweight data interchange format that is easy for humans to read and write, while CSV is a simple format used for tabular data representation. -
Can I convert large JSON files to CSV using Python?
Yes, Python can handle large JSON files, but it may require more memory. Consider using chunking methods or libraries like Dask for very large datasets. -
Is it necessary to use pandas for this conversion?
No, you can use Python’s built-in libraries like csv and json to perform the conversion without any external dependencies. -
How do I handle nested JSON structures when converting to CSV?
Flatten the JSON structure by extracting the necessary fields before writing to CSV. You can use loops or libraries like pandas to help with this. -
Can I convert CSV back to JSON in Python?
Yes, you can easily convert CSV back to JSON using pandas or built-in libraries, depending on your requirements.
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInRelated Article - Python JSON
- How to Get JSON From URL in Python
- How to Pretty Print a JSON File in Python
- How to Append Data to a JSON File Using Python
- How to Compare Multilevel JSON Objects Using JSON Diff in Python
- How to Flatten JSON in Python
- How to Serialize a Python Class Object to JSON
Related Article - Python CSV
- How to Import Multiple CSV Files Into Pandas and Concatenate Into One DataFrame
- How to Split CSV Into Multiple Files in Python
- How to Compare Two CSV Files and Print Differences Using Python
- How to Convert XLSX to CSV File in Python
- How to Write List to CSV Columns in Python
- How to Write to CSV Line by Line in Python